Clean Code and Architecture for Game Development
This post is an excerpt from the book Learning Game Architecture with Unity Architecture, architecture, architecture — it's everywhere. Be it in buildings, crafts, or games. But before jumping directly into Game Architecture and why it should be considered for your project, let's first discuss the No-Architecture problem — and why following a no-architecture approach could turn into a nightmare for your project in the long run. The No-Architecture problem A No-Architecture issue, in the context of software development, especially game development, refers to a situation where a project lacks a well-defined and structured architectural framework. In simpler terms, it means that the software or game has been developed without a clear plan or design for how its components, systems, and modules should interact and work together. In summary, a No- Architecture issue indicates a lack of proper planning and structure in game development, leading to disorganization, inefficiency, and a range of challenges that can impede the success of a game project. Addressing this issue often involves implementing a well-thought-out architectural framework to guide development and promote more efficient and maintainable projects. When a game project lacks a well-defined architectural framework, it often encounters difficulties during the various phases of development, particularly when it comes to bug fixes. Fixing bugs at later stages, such as during feature implementation, testing, or even post-release phases, can prove to be a daunting task. The absence of a clear architectural roadmap makes it harder to trace the origin of bugs, leading to extended debugging periods. This delay can have significant repercussions, both in terms of financial costs and man-hours. How Game Architecture manages to avoid issues poised by No-Architecture problem Game architecture plays a crucial role in better project management for game development. game architecture is required for better project management in game development because it brings structure, organization, and efficiency to the development process. It allows for smooth collaboration, scalability, and modularity while promoting code quality and maintainability. By following sound architectural principles, game developers can better manage their projects, meet deadlines, and deliver high-quality games to their players. Let us elaborate on how game architecture focuses on breaking down the project into logical modules, reusing these modules for efficiency, incorporating software design principles for clean code, and leveraging game design patterns for modular and maintainable code. So now we can identify the three pillars of game architecture as: Modular project breakdown Guided by software design principles Harnessing game design patterns Modular project breakdown Game architecture involves a systematic breakdown of a game project into logical and self-contained modules. Each module typically represents a specific aspect or feature of the game, such as gameplay mechanics, graphics rendering, audio, and user interfaces. Efficient development: Breaking the project into modules allows different teams or developers to work on distinct parts of the game simultaneously. This parallel development process enhances efficiency and helps meet project deadlines. Code re-usability: Game architecture encourages the creation of reusable modules. These modules can be employed not only within the current project but also in future projects. This re-usability minimizes redundancy, saves development time, and ensures consistency across different games. Guided by software design principles To maintain clean and well-structured code, game architecture draws upon established software design principles. Some popular software design principles are SOLID principles, Don’t Repeat yourself (DRY) principles and Keep it simple and Stupid (KISS) principles. These principles guide the architectural decisions to ensure code quality and maintainability. Modularity: Modularity is a fundamental software design principle, and game architecture aligns with it. Each module encapsulates a specific functionality or set of features, making it easier to manage and maintain. Changes to one module have minimal impact on the others, reducing the risk of unintended side effects and simplifying project management. Abstraction: Game architecture utilizes abstraction to hide the complexity of underlying systems. This makes the code more understandable and maintainable. Abstraction enables developers to focus on high-level concepts without getting bogged down in the implementation details. Separation of concerns: Game architecture enforces a separation of concerns, where each module has a specific, well-defined responsibility. This separation helps developers address specific issues or make improvements without affecting unrelated parts of the g
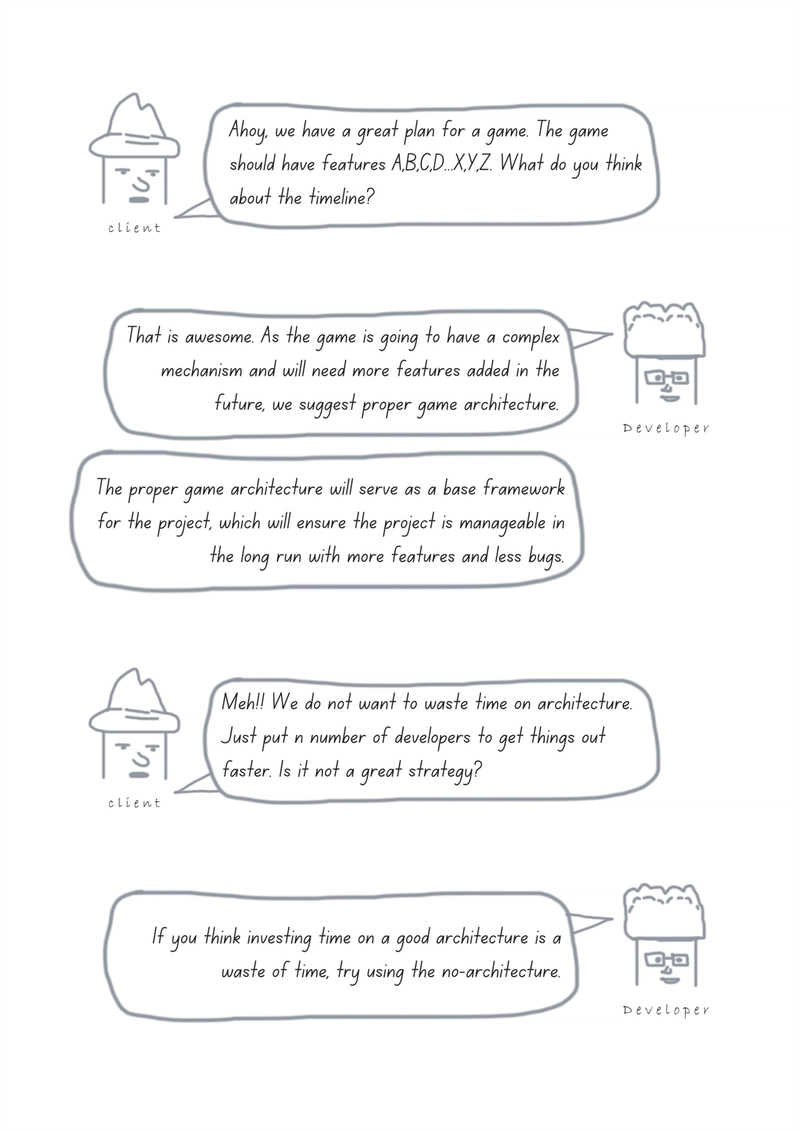
This post is an excerpt from the book Learning Game Architecture with Unity
Architecture, architecture, architecture — it's everywhere. Be it in buildings, crafts, or games.
But before jumping directly into Game Architecture and why it should be considered for your project, let's first discuss the No-Architecture problem — and why following a no-architecture approach could turn into a nightmare for your project in the long run.
The No-Architecture problem
A No-Architecture issue, in the context of software development, especially game development, refers to a situation where a project lacks a well-defined and structured architectural framework. In simpler terms, it means that the software or game has been developed without a clear plan or design for how its components, systems, and modules should interact and work together.
In summary, a No- Architecture issue indicates a lack of proper planning and structure in game development, leading to disorganization, inefficiency, and a range of challenges that can impede the success of a game project. Addressing this issue often involves implementing a well-thought-out architectural framework to guide development and promote more efficient and maintainable projects.
When a game project lacks a well-defined architectural framework, it often encounters difficulties during the various phases of development, particularly when it comes to bug fixes. Fixing bugs at later stages, such as during feature implementation, testing, or even post-release phases, can prove to be a daunting task. The absence of a clear architectural roadmap makes it harder to trace the origin of bugs, leading to extended debugging periods. This delay can have significant repercussions, both in terms of financial costs and man-hours.
How Game Architecture manages to avoid issues poised by No-Architecture problem
Game architecture plays a crucial role in better project management for game development. game architecture is required for better project management in game development because it brings structure, organization, and efficiency to the development process. It allows for smooth collaboration, scalability, and modularity while promoting code quality and maintainability. By following sound architectural principles, game developers can better manage their projects, meet deadlines, and deliver high-quality games to their players.
Let us elaborate on how game architecture focuses on breaking down the project into logical modules, reusing these modules for efficiency, incorporating software design principles for clean code, and leveraging game design patterns for modular and maintainable code.
So now we can identify the three pillars of game architecture as:
- Modular project breakdown
- Guided by software design principles
- Harnessing game design patterns
Modular project breakdown
Game architecture involves a systematic breakdown of a game project into logical and self-contained modules. Each module typically represents a specific aspect or feature of the game, such as gameplay mechanics, graphics rendering, audio, and user interfaces.
- Efficient development: Breaking the project into modules allows different teams or developers to work on distinct parts of the game simultaneously. This parallel development process enhances efficiency and helps meet project deadlines.
- Code re-usability: Game architecture encourages the creation of reusable modules. These modules can be employed not only within the current project but also in future projects. This re-usability minimizes redundancy, saves development time, and ensures consistency across different games.
Guided by software design principles
To maintain clean and well-structured code, game architecture draws upon established software design principles. Some popular software design principles are SOLID principles, Don’t Repeat yourself (DRY) principles and Keep it simple and Stupid (KISS) principles. These principles guide the architectural decisions to ensure code quality and maintainability.
- Modularity: Modularity is a fundamental software design principle, and game architecture aligns with it. Each module encapsulates a specific functionality or set of features, making it easier to manage and maintain. Changes to one module have minimal impact on the others, reducing the risk of unintended side effects and simplifying project management.
- Abstraction: Game architecture utilizes abstraction to hide the complexity of underlying systems. This makes the code more understandable and maintainable. Abstraction enables developers to focus on high-level concepts without getting bogged down in the implementation details.
- Separation of concerns: Game architecture enforces a separation of concerns, where each module has a specific, well-defined responsibility. This separation helps developers address specific issues or make improvements without affecting unrelated parts of the game.
Harnessing game design patterns
Game architecture incorporates game design patterns to create more modular and maintainable code. Some popular game design patterns include the Singleton, Observer, Command, and State Machine patterns. We will learn more about these patterns in upcoming chapters. These patterns are solutions to common design challenges in game development, improving the overall structure of the game.
- Modular code: Game design patterns emphasize modular design. They provide blueprints for organizing code into manageable components, making it easier to develop, understand, and modify specific functionalities.
- Maintainable code: By following established game design patterns, developers create code that is inherently maintainable. This means that as the game evolves and requires updates, developers can make changes with confidence, knowing that the existing architectural structure supports maintainability.
All these points related to Game architecture and their implementation are covered in detail in the book Learning Game Architecture with Unity, which is available @ Amazon, Amazon India, BPB International, BPB India.
In summary, game architecture promotes efficient development by breaking the project into logical modules and encouraging code re-usability. It leverages software design principles to ensure clean, organized code, while integrating game design patterns to create modular and maintainable solutions. This holistic approach is crucial for successful game development, helping developers manage projects effectively and deliver high-quality games.