Building an NSFW AI Image Generator with Next.js and Tailwind CSS
Building an NSFW AI Image Generator with Next.js and Tailwind CSS Creating an AI image generator that can handle NSFW (Not Safe For Work) content is an exciting challenge that combines cutting-edge technologies. In this tutorial, we'll walk through building an NSFW AI image generator using Next.js, TypeScript, and Tailwind CSS. We'll cover setting up the project, integrating the UI library, and handling AI-generated images securely. Introduction AI image generators have gained popularity for their ability to create images from textual descriptions. By leveraging frameworks like Next.js and libraries like Tailwind CSS, we can build a responsive and performant web application. In this tutorial, we'll focus on: Setting up a Next.js project with TypeScript Integrating Tailwind CSS for styling Building the user interface for input and image display Implementing the logic to handle AI image generation, including NSFW content Deploying the application Let's get started! Prerequisites Before we begin, make sure you have the following installed: Node.js (>= 14.x) npm or yarn package manager Basic knowledge of React and TypeScript is also recommended. 1. Setting Up the Project with Next.js and TypeScript First, we'll initialize a new Next.js project with TypeScript support. Initialize the Project Run the following command to create a new Next.js app: npx create-next-app@latest nsfw-ai-image-generator --typescript This command sets up a new Next.js project named nsfw-ai-image-generator with TypeScript configuration. Project Structure The project structure should look like this: nsfw-ai-image-generator/ ├── pages/ │ ├── _app.tsx │ ├── index.tsx ├── public/ ├── styles/ │ └── globals.css ├── tsconfig.json ├── package.json 2. Integrating Tailwind CSS Next, we'll add Tailwind CSS to style our application. Install Tailwind CSS Run the following command to install Tailwind CSS and its dependencies: npm install -D tailwindcss postcss autoprefixer Initialize Tailwind Configuration Generate the Tailwind and PostCSS configuration files: npx tailwindcss init -p Configure Tailwind Modify tailwind.config.js to specify the paths to all of your template files: module.exports = { content: [ './pages/**/*.{js,ts,jsx,tsx}', './components/**/*.{js,ts,jsx,tsx}', ], theme: { extend: {}, }, plugins: [], } Include Tailwind in CSS Add the Tailwind directives to styles/globals.css: @tailwind base; @tailwind components; @tailwind utilities; 3. Building the NSFW AI Image Generator UI Now, let's build the user interface for our application. Creating the Input Form Open pages/index.tsx and replace its content with the following: import { useState } from 'react'; const Home = () => { const [prompt, setPrompt] = useState(''); const [imageSrc, setImageSrc] = useState(''); const [isLoading, setIsLoading] = useState(false); const [error, setError] = useState(''); const handleGenerateImage = async () => { setIsLoading(true); setError(''); try { const response = await fetch('/api/generate', { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ prompt }), }); if (!response.ok) throw new Error('Image generation failed'); const { imageUrl, isNSFW } = await response.json(); if (isNSFW) { setError('NSFW content detected'); return; } setImageSrc(imageUrl); } catch (err) { setError(err.message || 'Failed to generate image'); } finally { setIsLoading(false); } }; return ( NSFW AI Image Generator setPrompt(e.target.value)} placeholder="Enter your prompt..." className="flex-1 p-2 border rounded-md" /> {isLoading ? 'Generating...' : 'Generate'} {error && ( {error} )} {imageSrc && ( Generated Image: )} ); }; export default Home; 4. Implementing AI Image Generation We'll use the Replicate API for Stable Diffusion implementation. Create a new API route at pages/api/generate.ts: import type { NextApiRequest, NextApiResponse } from 'next'; export default async function handler( req: NextApiRequest, res: NextApiResponse ) { const { prompt } = req.body; try { const response = await fetch('https://api.replicate.com/v1/predictions', { method: 'POST', headers: { 'Content-Type': 'application/json', Authorization: `Token ${process.env.REPLICATE_API_TOKEN}`, }, body: JSON.stringify({
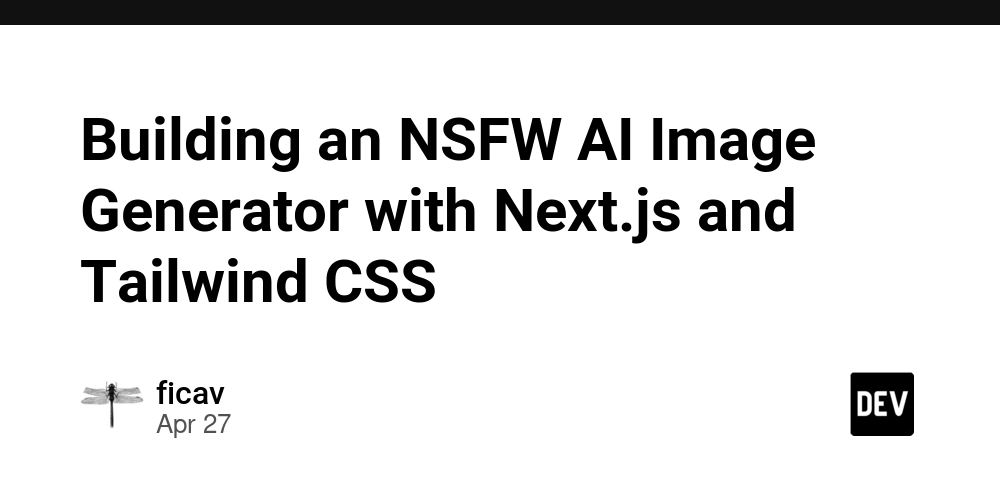
Building an NSFW AI Image Generator with Next.js and Tailwind CSS
Creating an AI image generator that can handle NSFW (Not Safe For Work) content is an exciting challenge that combines cutting-edge technologies. In this tutorial, we'll walk through building an NSFW AI image generator using Next.js, TypeScript, and Tailwind CSS. We'll cover setting up the project, integrating the UI library, and handling AI-generated images securely.
Introduction
AI image generators have gained popularity for their ability to create images from textual descriptions. By leveraging frameworks like Next.js and libraries like Tailwind CSS, we can build a responsive and performant web application. In this tutorial, we'll focus on:
- Setting up a Next.js project with TypeScript
- Integrating Tailwind CSS for styling
- Building the user interface for input and image display
- Implementing the logic to handle AI image generation, including NSFW content
- Deploying the application
Let's get started!
Prerequisites
Before we begin, make sure you have the following installed:
- Node.js (>= 14.x)
- npm or yarn package manager
Basic knowledge of React and TypeScript is also recommended.
1. Setting Up the Project with Next.js and TypeScript
First, we'll initialize a new Next.js project with TypeScript support.
Initialize the Project
Run the following command to create a new Next.js app:
npx create-next-app@latest nsfw-ai-image-generator --typescript
This command sets up a new Next.js project named nsfw-ai-image-generator
with TypeScript configuration.
Project Structure
The project structure should look like this:
nsfw-ai-image-generator/
├── pages/
│ ├── _app.tsx
│ ├── index.tsx
├── public/
├── styles/
│ └── globals.css
├── tsconfig.json
├── package.json
2. Integrating Tailwind CSS
Next, we'll add Tailwind CSS to style our application.
Install Tailwind CSS
Run the following command to install Tailwind CSS and its dependencies:
npm install -D tailwindcss postcss autoprefixer
Initialize Tailwind Configuration
Generate the Tailwind and PostCSS configuration files:
npx tailwindcss init -p
Configure Tailwind
Modify tailwind.config.js
to specify the paths to all of your template files:
module.exports = {
content: [
'./pages/**/*.{js,ts,jsx,tsx}',
'./components/**/*.{js,ts,jsx,tsx}',
],
theme: {
extend: {},
},
plugins: [],
}
Include Tailwind in CSS
Add the Tailwind directives to styles/globals.css
:
@tailwind base;
@tailwind components;
@tailwind utilities;
3. Building the NSFW AI Image Generator UI
Now, let's build the user interface for our application.
Creating the Input Form
Open pages/index.tsx
and replace its content with the following:
import { useState } from 'react';
const Home = () => {
const [prompt, setPrompt] = useState('');
const [imageSrc, setImageSrc] = useState('');
const [isLoading, setIsLoading] = useState(false);
const [error, setError] = useState('');
const handleGenerateImage = async () => {
setIsLoading(true);
setError('');
try {
const response = await fetch('/api/generate', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ prompt }),
});
if (!response.ok) throw new Error('Image generation failed');
const { imageUrl, isNSFW } = await response.json();
if (isNSFW) {
setError('NSFW content detected');
return;
}
setImageSrc(imageUrl);
} catch (err) {
setError(err.message || 'Failed to generate image');
} finally {
setIsLoading(false);
}
};
return (
<div className="min-h-screen bg-gray-100 py-8 px-4">
<div className="max-w-2xl mx-auto">
<h1 className="text-4xl font-bold text-center mb-8">
NSFW AI Image Generator
h1>
<div className="bg-white rounded-lg shadow-md p-6">
<div className="flex gap-4 mb-4">
<input
type="text"
value={prompt}
onChange={(e) => setPrompt(e.target.value)}
placeholder="Enter your prompt..."
className="flex-1 p-2 border rounded-md"
/>
<button
onClick={handleGenerateImage}
disabled={isLoading}
className="bg-blue-600 text-white px-6 py-2 rounded-md hover:bg-blue-700 disabled:opacity-50"
>
{isLoading ? 'Generating...' : 'Generate'}
button>
div>
{error && (
<div className="text-red-500 mb-4">{error}div>
)}
{imageSrc && (
<div className="mt-6">
<h2 className="text-xl font-semibold mb-4">Generated Image:h2>
<img
src={imageSrc}
alt="Generated"
className="w-full h-auto rounded-lg shadow-md"
/>
div>
)}
div>
div>
div>
);
};
export default Home;
4. Implementing AI Image Generation
We'll use the Replicate API for Stable Diffusion implementation. Create a new API route at pages/api/generate.ts
:
import type { NextApiRequest, NextApiResponse } from 'next';
export default async function handler(
req: NextApiRequest,
res: NextApiResponse
) {
const { prompt } = req.body;
try {
const response = await fetch('https://api.replicate.com/v1/predictions', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
Authorization: `Token ${process.env.REPLICATE_API_TOKEN}`,
},
body: JSON.stringify({
version: 'a9758cbfbd5f3c2094457d996681af52552901775aa2d6dd0b17fd15df959bef',
input: {
prompt: prompt,
width: 512,
height: 512,
num_outputs: 1,
},
}),
});
const prediction = await response.json();
// Add NSFW detection logic here
const isNSFW = await checkNSFW(prediction.output[0]);
res.status(200).json({
imageUrl: prediction.output[0],
isNSFW
});
} catch (error) {
res.status(500).json({ error: error.message });
}
}
// Example NSFW detection using NSFWJS
async function checkNSFW(imageUrl: string): Promise<boolean> {
const tf = await import('@tensorflow/tfjs');
const nsfwjs = await import('nsfwjs');
const model = await nsfwjs.load();
const image = await fetch(imageUrl);
const buffer = await image.arrayBuffer();
const img = tf.node.decodeImage(new Uint8Array(buffer), 3);
const predictions = await model.classify(img);
return predictions.some(p =>
['Hentai', 'Porn', 'Sexy'].includes(p.className)
);
}
5. Handling NSFW Content Safely
Implement multiple layers of safety:
- Input Validation: Filter explicit prompts using regex patterns
- Output Filtering: Use NSFWJS for image classification
- Content Moderation: Integrate third-party APIs like Sightengine
Add input validation middleware to your API route:
function validatePrompt(prompt: string): boolean {
const blockedTerms = [
'nudity', 'explicit', 'porn',
'sexual', 'nsfw', 'adult'
];
return !new RegExp(blockedTerms.join('|'), 'i').test(prompt);
}
6. Deployment
Deploy to Vercel with proper environment variables:
- Create
.env.local
file:
REPLICATE_API_TOKEN=your_api_token_here
- Configure in
vercel.json
:
{
"build": {
"env": {
"REPLICATE_API_TOKEN": "@replicate-api-token"
}
}
}
- Run deployment:
vercel deploy --prod
Conclusion
In this tutorial, we've built an NSFW-aware AI image generator using:
- Next.js for server-side logic and API routes
- Tailwind CSS for modern styling
- Stable Diffusion for image generation
- TensorFlow.js for NSFW classification
Remember to implement additional safety measures and consider ethical implications before deploying such applications to production. Always comply with your local regulations and AI service providers' terms of service.