Building Accessible Angular Applications (WCAG, ARIA)
Accessibility (a11y) is essential for building inclusive Angular applications that can be used by everyone, including users with disabilities. By following WCAG (Web Content Accessibility Guidelines) and using ARIA (Accessible Rich Internet Applications), you can ensure your apps are usable and compliant. Why Accessibility Matters Legal compliance: Many countries enforce accessibility regulations (e.g., ADA, EN 301 549). Inclusive design: Helps users with visual, auditory, motor, and cognitive impairments. Better UX: Often results in cleaner, more usable UI for all users. WCAG Principles Overview WCAG guidelines are based on four main principles: Perceivable – Users must be able to perceive the content. Operable – Interface components must be operable via keyboard and other inputs. Understandable – Content must be easy to read and operate. Robust – Content must be accessible by assistive technologies. Key Accessibility Features in Angular 1. Semantic HTML Angular supports all native HTML elements. Always use semantic tags (, , , , etc.) over generic elements like . Submit Submit 2. ARIA Attributes ARIA attributes enhance the accessibility of dynamic components. X Use ARIA roles and attributes only when semantic HTML isn’t enough: Settings 3. Keyboard Navigation Ensure all interactive components are accessible using a keyboard: Use tabindex="0" to make custom components focusable. Use Angular’s @HostListener('keydown', ['$event']) to handle keyboard events. @HostListener('keydown', ['$event']) onKeyDown(event: KeyboardEvent) { if (event.key === 'Enter') { this.performAction(); } } 4. Focus Management Handle focus programmatically when modals or dynamic elements appear: import { ElementRef, ViewChild } from '@angular/core'; @ViewChild('closeButton') closeButton!: ElementRef; ngAfterViewInit() { this.closeButton.nativeElement.focus(); } 5. Forms and Labels Ensure form inputs have associated labels: Email: 6. Color Contrast and Visual Indicators Use high-contrast colors and visible focus indicators. Tools like axe or Lighthouse can help audit contrast ratios. Testing Accessibility Use the following tools to test a11y in Angular apps: axe DevTools (browser extension) NVDA / VoiceOver / JAWS screen readers Chrome Lighthouse a11y audit Angular cdk/a11y for utilities like FocusMonitor import { FocusMonitor } from '@angular/cdk/a11y'; constructor(private fm: FocusMonitor) {} Conclusion By incorporating accessibility into your Angular development process, you not only meet compliance standards but also create better user experiences for everyone. Focus on semantic HTML, ARIA usage, keyboard support, and thorough testing. Accessibility isn’t an afterthought — it's a core part of building quality software. Ready to level up your Angular apps with a11y? Let’s do it!
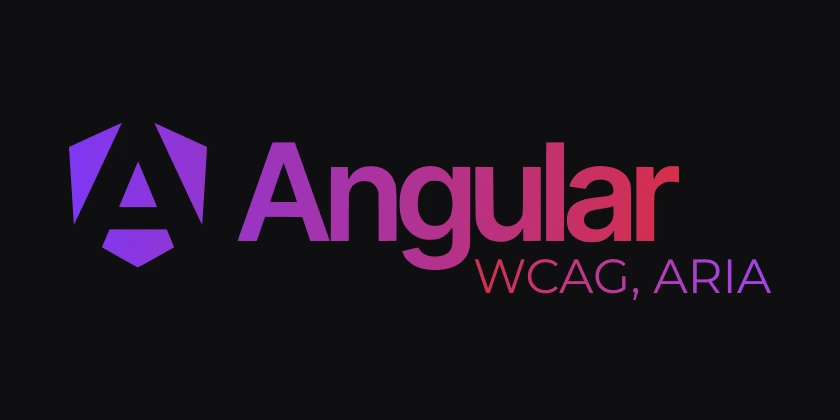
Accessibility (a11y) is essential for building inclusive Angular applications that can be used by everyone, including users with disabilities. By following WCAG (Web Content Accessibility Guidelines) and using ARIA (Accessible Rich Internet Applications), you can ensure your apps are usable and compliant.
Why Accessibility Matters
- Legal compliance: Many countries enforce accessibility regulations (e.g., ADA, EN 301 549).
- Inclusive design: Helps users with visual, auditory, motor, and cognitive impairments.
- Better UX: Often results in cleaner, more usable UI for all users.
WCAG Principles Overview
WCAG guidelines are based on four main principles:
- Perceivable – Users must be able to perceive the content.
- Operable – Interface components must be operable via keyboard and other inputs.
- Understandable – Content must be easy to read and operate.
- Robust – Content must be accessible by assistive technologies.
Key Accessibility Features in Angular
1. Semantic HTML
Angular supports all native HTML elements. Always use semantic tags ( ARIA attributes enhance the accessibility of dynamic components. Use ARIA roles and attributes only when semantic HTML isn’t enough: Ensure all interactive components are accessible using a keyboard:
Handle focus programmatically when modals or dynamic elements appear: Ensure form inputs have associated labels: Use high-contrast colors and visible focus indicators. Tools like axe or Lighthouse can help audit contrast ratios.
Use the following tools to test a11y in Angular apps:
By incorporating accessibility into your Angular development process, you not only meet compliance standards but also create better user experiences for everyone. Focus on semantic HTML, ARIA usage, keyboard support, and thorough testing.
Accessibility isn’t an afterthought — it's a core part of building quality software.
Ready to level up your Angular apps with a11y? Let’s do it! ,
,
,
, etc.) over generic elements like
Submit
2. ARIA Attributes
id="dialogTitle">Settings
3. Keyboard Navigation
tabindex="0"
to make custom components focusable.@HostListener('keydown', ['$event'])
to handle keyboard events.
@HostListener('keydown', ['$event'])
onKeyDown(event: KeyboardEvent) {
if (event.key === 'Enter') {
this.performAction();
}
}
4. Focus Management
import { ElementRef, ViewChild } from '@angular/core';
@ViewChild('closeButton') closeButton!: ElementRef;
ngAfterViewInit() {
this.closeButton.nativeElement.focus();
}
5. Forms and Labels
id="email" type="email" />
6. Color Contrast and Visual Indicators
Testing Accessibility
FocusMonitor
import { FocusMonitor } from '@angular/cdk/a11y';
constructor(private fm: FocusMonitor) {}
Conclusion