Building a Simple Dodge Game with HTML Canvas and JavaScript
In this tutorial, we'll create a basic browser game where players control a red square that must dodge falling blue squares. This project is perfect for beginners looking to understand HTML5 Canvas and basic game development concepts. You can see the complete code on Github What We'll Build Our game will feature: A player-controlled red square at the bottom of the screen Multiple blue squares are falling from the top Keyboard arrow controls for movement Game over when a collision is detected Let's get started! Step 1: Setting Up the HTML Structure First, we need to create an HTML file with a canvas element: Square Dodge Game // Our game code will go here The canvas element will be our game area with dimensions of 900x600 pixels. Step 2: Setting Up Variables and Initial Game State Now, let's define the variables we'll need for our game: const canvas = document.querySelector("canvas") const ctx = canvas.getContext("2d") let rightPressed = false let leftPressed = false playerX = canvas.width / 2 const playerSize = 50 let interval = 0 const squares = [] These variables will: Get a reference to our canvas and its drawing context Track the keyboard input state (left and right arrow keys) Set the player's initial X position to the center of the canvas Define the size of all squares Create an interval variable for our game loop Create an array to store our falling square objects Step 3: Creating the Falling Squares Let's populate our game with some falling squares: for (let i = 0; i
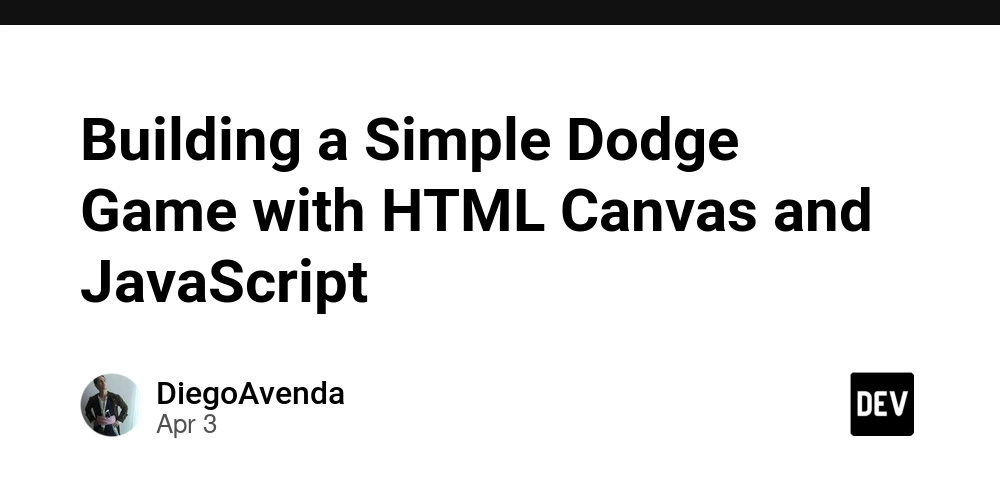
In this tutorial, we'll create a basic browser game where players control a red square that must dodge falling blue squares. This project is perfect for beginners looking to understand HTML5 Canvas and basic game development concepts.
You can see the complete code on Github
What We'll Build
Our game will feature:
- A player-controlled red square at the bottom of the screen
- Multiple blue squares are falling from the top
- Keyboard arrow controls for movement
- Game over when a collision is detected
Let's get started!
Step 1: Setting Up the HTML Structure
First, we need to create an HTML file with a canvas element:
lang="en">
charset="UTF-8" />
name="viewport" content="width=device-width, initial-scale=1.0" />
Square Dodge Game
// Our game code will go here
The canvas element will be our game area with dimensions of 900x600 pixels.
Step 2: Setting Up Variables and Initial Game State
Now, let's define the variables we'll need for our game:
const canvas = document.querySelector("canvas")
const ctx = canvas.getContext("2d")
let rightPressed = false
let leftPressed = false
playerX = canvas.width / 2
const playerSize = 50
let interval = 0
const squares = []
These variables will:
- Get a reference to our canvas and its drawing context
- Track the keyboard input state (left and right arrow keys)
- Set the player's initial X position to the center of the canvas
- Define the size of all squares
- Create an interval variable for our game loop
- Create an array to store our falling square objects
Step 3: Creating the Falling Squares
Let's populate our game with some falling squares:
for (let i = 0; i < 5; i++) {
squares.push({
x: Math.random() * (canvas.width - playerSize),
y: Math.random() * (canvas.height - playerSize),
size: playerSize,
})
}
This loop creates 5 squares with:
- Random X positions within the canvas width
- Random Y positions within the canvas height
- The same size as our player square
Step 4: Drawing the Squares and Handling Their Movement
Next, we'll create a function to draw and update our falling squares:
function drawSquares() {
squares.forEach((square) => {
ctx.beginPath()
ctx.rect(square.x, square.y, square.size, square.size)
ctx.fillStyle = "blue"
ctx.fill()
ctx.closePath()
square.y += 1 // Move the square down
if (square.y > canvas.height) {
square.y = 0 - square.size
square.x = Math.random() * (canvas.width - playerSize)
}
// Collision detection
if (
playerX < square.x + square.size &&
playerX + playerSize > square.x &&
canvas.height - playerSize - 5 < square.y + square.size
) {
alert("Game Over")
document.location.reload()
clearInterval(interval) // Needed for Chrome to end game
}
})
}
This function:
- Draws each square in blue
- Moves each square down by 1 pixel each frame
- Resets squares to the top with a new random X position when they go off-screen
- Checks for collisions with the player and triggers game over if detected
Step 5: Drawing the Player
Now, let's create a function to draw our player:
function drawPlayer() {
ctx.beginPath()
ctx.rect(
playerX,
canvas.height - playerSize - 5,
playerSize,
playerSize
)
ctx.fillStyle = "red"
ctx.fill()
ctx.closePath()
}
This draws a red square at the bottom of the canvas, with its position determined by the playerX
variable.
Step 6: The Main Game Loop
Now we'll create our main drawing function that runs continuously:
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height)
ctx.beginPath()
ctx.strokeRect(0, 0, canvas.width, canvas.height)
ctx.closePath()
drawPlayer()
drawSquares()
if (rightPressed) {
playerX += 7
} else if (leftPressed) {
playerX -= 7
}
}
interval = setInterval(draw, 10)
This function:
- Clears the canvas
- Draws a border around the game area
- Draws the player and falling squares
- Updates the player's position based on keyboard input
- Runs every 10 milliseconds (about 100 frames per second)
Step 7: Handling Keyboard Input
Finally, we need to add event listeners for keyboard input:
document.addEventListener("keydown", keyDownHandler, false)
document.addEventListener("keyup", keyUpHandler, false)
function keyDownHandler(e) {
if (e.key === "Right" || e.key === "ArrowRight") {
rightPressed = true
} else if (e.key === "Left" || e.key === "ArrowLeft") {
leftPressed = true
}
}
function keyUpHandler(e) {
if (e.key === "Right" || e.key === "ArrowRight") {
rightPressed = false
} else if (e.key === "Left" || e.key === "ArrowLeft") {
leftPressed = false
}
}
These functions:
- Add event listeners for key presses and releases
- Track when the left and right arrow keys are pressed
- Update the corresponding Boolean variables that control player movement.
This simple game demonstrates the fundamentals of game development: drawing to a canvas, handling user input, implementing game physics, and detecting collisions. These basics allow you to expand and create more complex and engaging games!