An Introduction to Functional Programming
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. It encourages writing clearer, more predictable code and has gained popularity with the rise of languages that support it. In this post, we’ll explore the core concepts of functional programming and its benefits. What is Functional Programming? Functional programming is based on the concept of pure functions, which have the following properties: Deterministic: Given the same input, a pure function always returns the same output. No Side Effects: Pure functions do not modify any external state or variables. Functional programming emphasizes immutability, higher-order functions, and function composition to build software. Key Concepts of Functional Programming First-Class Functions: Functions can be assigned to variables, passed as arguments, or returned from other functions. Higher-Order Functions: Functions that take other functions as parameters or return functions as results. Immutability: Once created, data cannot be changed. Instead, new data structures are created from existing ones. Pure Functions: Functions that have no side effects and always produce the same output for the same input. Function Composition: Combining simple functions to create more complex functions. Lazy Evaluation: Values are computed only when needed, which can improve performance. Benefits of Functional Programming Modularity: Code is easier to understand and maintain when broken into small, independent functions. Reusability: Functions can be reused in different contexts, reducing code duplication. Easier Testing: Pure functions are easier to test since their output is solely based on their input. Concurrency: Immutability and stateless functions make concurrent programming safer and simpler. Functional Programming Languages While many programming languages support functional programming concepts, some are designed specifically for it: Haskell: A purely functional programming language. Scala: A language that combines functional and object-oriented programming. F#: A functional-first language in the .NET ecosystem. Elixir: A functional language designed for scalable and maintainable applications. JavaScript: Supports functional programming principles and higher-order functions. Python: Allows functional programming features through functions like map(), filter(), and reduce(). Example: Functional Programming in JavaScript const numbers = [1, 2, 3, 4, 5]; // Pure function const square = (x) => x * x; // Higher-order function const squaredNumbers = numbers.map(square); console.log(squaredNumbers); // [1, 4, 9, 16, 25] Example: Function Composition const add = (x) => (y) => x + y; const increment = add(1); const result = increment(5); console.log(result); // 6 Conclusion Functional programming offers a powerful approach to writing clean, maintainable, and predictable code. By embracing its principles, developers can create applications that are easier to reason about and maintain over time. Whether you’re exploring a new language or enhancing your current programming skills, understanding functional programming is a valuable addition to your toolkit.
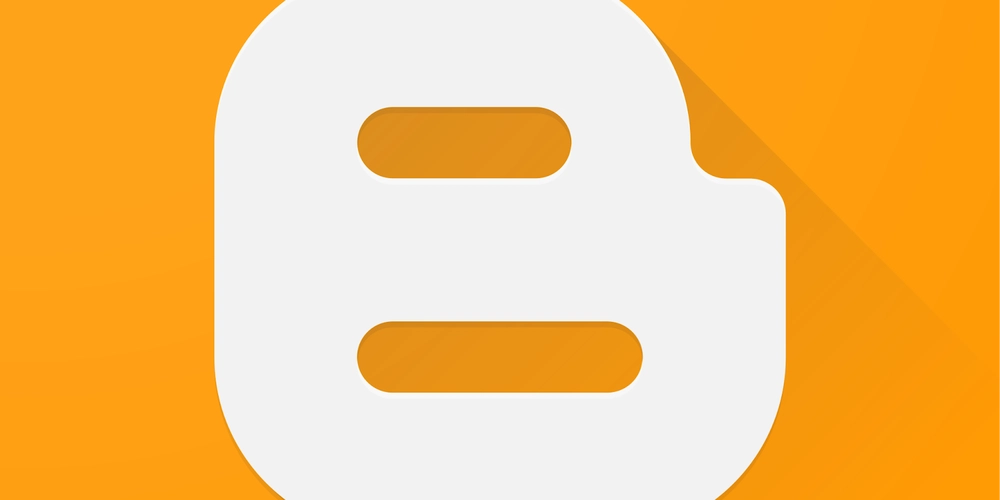
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. It encourages writing clearer, more predictable code and has gained popularity with the rise of languages that support it. In this post, we’ll explore the core concepts of functional programming and its benefits.
What is Functional Programming?
Functional programming is based on the concept of pure functions, which have the following properties:
- Deterministic: Given the same input, a pure function always returns the same output.
- No Side Effects: Pure functions do not modify any external state or variables.
Functional programming emphasizes immutability, higher-order functions, and function composition to build software.
Key Concepts of Functional Programming
- First-Class Functions: Functions can be assigned to variables, passed as arguments, or returned from other functions.
- Higher-Order Functions: Functions that take other functions as parameters or return functions as results.
- Immutability: Once created, data cannot be changed. Instead, new data structures are created from existing ones.
- Pure Functions: Functions that have no side effects and always produce the same output for the same input.
- Function Composition: Combining simple functions to create more complex functions.
- Lazy Evaluation: Values are computed only when needed, which can improve performance.
Benefits of Functional Programming
- Modularity: Code is easier to understand and maintain when broken into small, independent functions.
- Reusability: Functions can be reused in different contexts, reducing code duplication.
- Easier Testing: Pure functions are easier to test since their output is solely based on their input.
- Concurrency: Immutability and stateless functions make concurrent programming safer and simpler.
Functional Programming Languages
While many programming languages support functional programming concepts, some are designed specifically for it:
- Haskell: A purely functional programming language.
- Scala: A language that combines functional and object-oriented programming.
- F#: A functional-first language in the .NET ecosystem.
- Elixir: A functional language designed for scalable and maintainable applications.
- JavaScript: Supports functional programming principles and higher-order functions.
-
Python: Allows functional programming features through functions like
map()
,filter()
, andreduce()
.
Example: Functional Programming in JavaScript
const numbers = [1, 2, 3, 4, 5];
// Pure function
const square = (x) => x * x;
// Higher-order function
const squaredNumbers = numbers.map(square);
console.log(squaredNumbers); // [1, 4, 9, 16, 25]
Example: Function Composition
const add = (x) => (y) => x + y;
const increment = add(1);
const result = increment(5);
console.log(result); // 6
Conclusion
Functional programming offers a powerful approach to writing clean, maintainable, and predictable code. By embracing its principles, developers can create applications that are easier to reason about and maintain over time. Whether you’re exploring a new language or enhancing your current programming skills, understanding functional programming is a valuable addition to your toolkit.