A Complete Guide to JavaScript DOM Selector Methods
JavaScript offers several methods for selecting DOM elements, including getElementById, querySelector, and getElementsByClassName. This article provides an in-depth exploration of each method's characteristics and practical usage, focusing on performance considerations and implementation best practices. Sample Code Let's examine how each method works using this HTML example: The Adventures of Sherlock Holmes A Study in Scarlet The Hound of the Baskervilles 1. getElementById: Fast and Type-Safe Element Selection const title = document.getElementById('title'); This is the simplest and fastest element selection method. It should be your first choice when ID-based element selection is possible. Features: Extremely fast due to internal ID mapping Type-safe return value (single element or null) Clear and readable code intention Limitations: Only works with ID attributes Cannot select multiple elements While less common in modern framework-based development, it remains a crucial tool when working with vanilla JavaScript. 2. getElementsByClassName: Dynamic Element Selection const titles = document.getElementsByClassName('title'); console.log([...titles]); // Convert HTMLCollection to array This method uniquely detects DOM element additions and deletions automatically. Features: Automatically detects and reflects DOM structure changes Faster than querySelectorAll for class-based searches Implementation Considerations: Returns HTMLCollection, requiring conversion for array operations Live updates can lead to unexpected behavior if not handled properly // Not recommended const elements = document.getElementsByClassName('target'); for (let i = 0; i el.remove()); 3. querySelector/querySelectorAll: Flexible Element Selection // Single element selection const firstTitle = document.querySelector('.title'); // Multiple element selection const allTitles = document.querySelectorAll('.title'); // Complex condition selection const mysteryBook = document.querySelector('.title[data-category="mystery"]'); These are the most widely used methods in modern web development. Features: Flexible element selection using CSS selectors Intuitive API design Consistent syntax for single and multiple element selection Considerations: Slower performance compared to other methods Complex selectors can reduce code readability Performance Analysis While performance differences are negligible in typical web applications, they become significant with extensive DOM operations: // Inefficient implementation for (let i = 0; i { // Operations }); } 2. Dynamic Content Handling // Good practice: Use live HTMLCollection const dynamicList = document.getElementsByClassName('dynamic-item'); // Automatically updates when elements are added/removed // Alternative: Use MutationObserver for complex cases const observer = new MutationObserver(() => { console.log('DOM updated'); }); Summary When choosing a DOM selector method, consider: Element identification method (ID, class, complex conditions) Performance requirements Dynamic update needs Code readability Making informed choices based on these factors leads to efficient and maintainable implementations. Additional Resources For more advanced DOM manipulation techniques and performance optimization strategies, consider exploring: MDN Web Docs: Document Object Model Web Performance: DOM Access Fundamentals
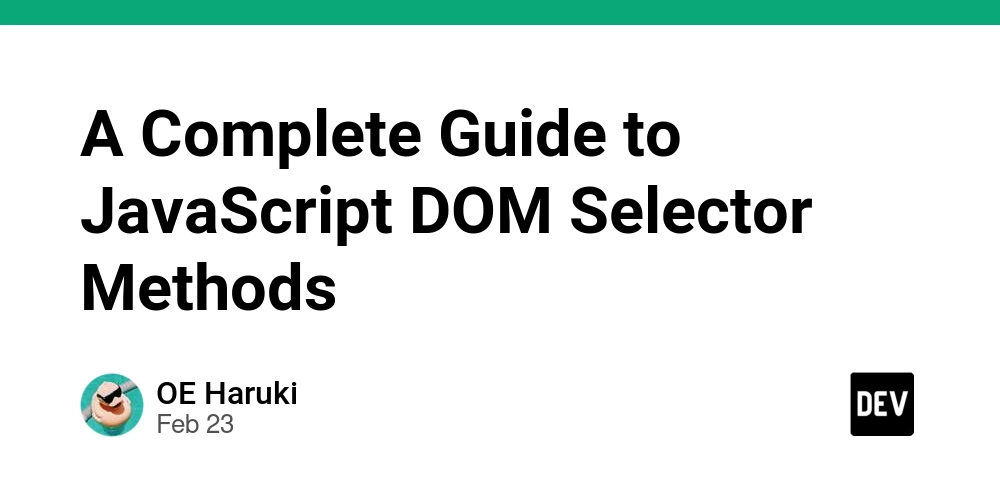
JavaScript offers several methods for selecting DOM elements, including getElementById
, querySelector
, and getElementsByClassName
.
This article provides an in-depth exploration of each method's characteristics and practical usage, focusing on performance considerations and implementation best practices.
Sample Code
Let's examine how each method works using this HTML example:
id="title" class="title">The Adventures of Sherlock Holmes
class="title">A Study in Scarlet
class="title" data-category="mystery">The Hound of the Baskervilles
1. getElementById: Fast and Type-Safe Element Selection
const title = document.getElementById('title');
This is the simplest and fastest element selection method. It should be your first choice when ID-based element selection is possible.
Features:
- Extremely fast due to internal ID mapping
- Type-safe return value (single element or null)
- Clear and readable code intention
Limitations:
- Only works with ID attributes
- Cannot select multiple elements
While less common in modern framework-based development, it remains a crucial tool when working with vanilla JavaScript.
2. getElementsByClassName: Dynamic Element Selection
const titles = document.getElementsByClassName('title');
console.log([...titles]); // Convert HTMLCollection to array
This method uniquely detects DOM element additions and deletions automatically.
Features:
- Automatically detects and reflects DOM structure changes
- Faster than querySelectorAll for class-based searches
Implementation Considerations:
- Returns HTMLCollection, requiring conversion for array operations
- Live updates can lead to unexpected behavior if not handled properly
// Not recommended
const elements = document.getElementsByClassName('target');
for (let i = 0; i < elements.length; i++) {
elements[i].remove(); // Unstable due to changing element count
}
// Recommended approach
[...document.getElementsByClassName('target')]
.forEach(el => el.remove());
3. querySelector/querySelectorAll: Flexible Element Selection
// Single element selection
const firstTitle = document.querySelector('.title');
// Multiple element selection
const allTitles = document.querySelectorAll('.title');
// Complex condition selection
const mysteryBook = document.querySelector('.title[data-category="mystery"]');
These are the most widely used methods in modern web development.
Features:
- Flexible element selection using CSS selectors
- Intuitive API design
- Consistent syntax for single and multiple element selection
Considerations:
- Slower performance compared to other methods
- Complex selectors can reduce code readability
Performance Analysis
While performance differences are negligible in typical web applications, they become significant with extensive DOM operations:
// Inefficient implementation
for (let i = 0; i < 1000; i++) {
document.querySelector('.title').style.color = 'red';
}
// Efficient implementation
const element = document.querySelector('.title');
for (let i = 0; i < 1000; i++) {
element.style.color = 'red';
}
Method Selection Guidelines
Here's when to use each method:
- For ID-based element selection:
getElementById
- When monitoring dynamic elements:
getElementsByClassName
- For complex selection criteria:
querySelector
/querySelectorAll
Understanding each method's characteristics enables maintainable implementation.
Performance Comparison Table
Method | Speed | Live Updates | Return Type | Best Use Case |
---|---|---|---|---|
getElementById | Fastest | No | Element \ | null |
getElementsByClassName | Fast | Yes | HTMLCollection | Dynamic list monitoring |
querySelector | Medium | No | Element \ | null |
querySelectorAll | Slowest | No | NodeList | Multiple element selection |
Implementation Best Practices
1. Element Caching
// Good practice: Cache frequently used elements
const container = document.getElementById('main-container');
const items = container.getElementsByClassName('item');
// Poor practice: Repeated queries
function updateItems() {
document.querySelectorAll('.item').forEach(item => {
// Operations
});
}
2. Dynamic Content Handling
// Good practice: Use live HTMLCollection
const dynamicList = document.getElementsByClassName('dynamic-item');
// Automatically updates when elements are added/removed
// Alternative: Use MutationObserver for complex cases
const observer = new MutationObserver(() => {
console.log('DOM updated');
});
Summary
When choosing a DOM selector method, consider:
- Element identification method (ID, class, complex conditions)
- Performance requirements
- Dynamic update needs
- Code readability
Making informed choices based on these factors leads to efficient and maintainable implementations.
Additional Resources
For more advanced DOM manipulation techniques and performance optimization strategies, consider exploring: