10 JavaScript Tricks Every Developer Should Know
Title: 10 JavaScript Tricks Every Developer Should Know Hello to all the code enthusiasts out there! Today, we're going to delve into the world of JavaScript, a cornerstone language of the web, and explore some tricks that can elevate your coding skills to a new level. Whether you're a novice developer or a seasoned programmer, these JavaScript tricks are sure to add a new dimension to your coding repertoire. So let's get started! Destructuring: Destructuring in JavaScript is a handy way to extract multiple properties from an object or array in a single statement. It can significantly simplify your code and make it more readable. For example: let [a, b] = [1, 2]; console.log(a); // outputs: 1 console.log(b); // outputs: 2 The same can be done with objects: let {name, age} = {name: "John", age: 22}; console.log(name); // outputs: John console.log(age); // outputs: 22 Optional Chaining: Optional chaining is a recent addition to JavaScript (ES2020), which allows you to access deeply nested object properties without having to check if each reference in the chain is valid. It helps in writing cleaner and safer code. Here's how it works: let user = {}; // an empty object console.log(user?.address?.street); // outputs: undefined, instead of throwing an error Spread/Rest Operators: The spread operator (…) in JavaScript is used to spread the elements of an array or object. The rest operator, also (…), is used to collect the rest of the elements into an array. Both these operators contribute to shorter and cleaner code: let arr1 = [1, 2, 3]; let arr2 = [...arr1, 4, 5]; // outputs: [1, 2, 3, 4, 5] For the rest operator: let [first, ...rest] = [1, 2, 3, 4, 5]; console.log(first); // outputs: 1 console.log(rest); // outputs: [2, 3, 4, 5] Short-circuit Evaluation: Short-circuit evaluation in JavaScript involves logical operators (&& and ||) to create shorter code. It can be used to set default values, validate objects before using them, and more: let name = user && user.name; let port = serverPort || 3000; Template Literals: Template literals provide an easy way to interpolate variables and expressions into strings. They are enclosed by backtick () characters instead of quotes: let name = "John"; console.log(`Hello, ${name}!`); // outputs: Hello, John! Arrow Functions: Arrow functions offer a more concise syntax for writing function expressions. They are ideal for non-method functions and they do not have their own 'this', arguments, super, or new.target: let hello = () => "Hello World"; console.log(hello()); // outputs: Hello World Nullish Coalescing Operator: The nullish coalescing operator (??) is a logical operator that returns its right-hand side operand when its left-hand side operand is null or undefined, and otherwise returns its left-hand side operand. let name = null ?? "default name"; console.log(name); // outputs: default name Ternary Operator: The ternary operator is a quicker way to write simple if-else statements. The syntax is: condition ? value_if_true : value_if_false: let age = 15; let type = age >= 18 ? "Adult" : "Minor"; console.log(type); // outputs: Minor Array Methods (map, reduce, filter): JavaScript provides powerful array methods like map, reduce, and filter. They are more efficient and elegant to use in comparison to traditional for-loops: let numbers = [1, 2, 3, 4, 5]; let doubled = numbers.map(n => n * 2); // outputs: [2, 4, 6, 8, 10] Async/Await: Async/Await makes asynchronous code look and behave more like synchronous code. This syntactic sugar on top of promises makes asynchronous code easier to understand and write: async function fetchUser() { let response = await fetch('https://api.github.com/users'); let data = await response.json(); console.log(data); } fetchUser(); That's all for this post. I hope you found these JavaScript tricks useful. Happy coding!
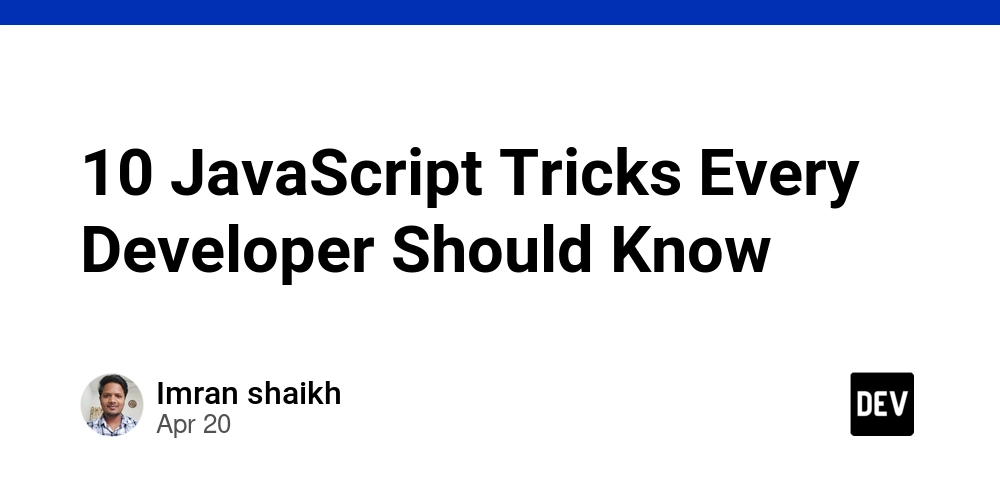
Title: 10 JavaScript Tricks Every Developer Should Know
Hello to all the code enthusiasts out there! Today, we're going to delve into the world of JavaScript, a cornerstone language of the web, and explore some tricks that can elevate your coding skills to a new level. Whether you're a novice developer or a seasoned programmer, these JavaScript tricks are sure to add a new dimension to your coding repertoire. So let's get started!
- Destructuring:
Destructuring in JavaScript is a handy way to extract multiple properties from an object or array in a single statement. It can significantly simplify your code and make it more readable. For example:
let [a, b] = [1, 2];
console.log(a); // outputs: 1
console.log(b); // outputs: 2
The same can be done with objects:
let {name, age} = {name: "John", age: 22};
console.log(name); // outputs: John
console.log(age); // outputs: 22
- Optional Chaining:
Optional chaining is a recent addition to JavaScript (ES2020), which allows you to access deeply nested object properties without having to check if each reference in the chain is valid. It helps in writing cleaner and safer code. Here's how it works:
let user = {}; // an empty object
console.log(user?.address?.street); // outputs: undefined, instead of throwing an error
- Spread/Rest Operators:
The spread operator (…) in JavaScript is used to spread the elements of an array or object. The rest operator, also (…), is used to collect the rest of the elements into an array. Both these operators contribute to shorter and cleaner code:
let arr1 = [1, 2, 3];
let arr2 = [...arr1, 4, 5]; // outputs: [1, 2, 3, 4, 5]
For the rest operator:
let [first, ...rest] = [1, 2, 3, 4, 5];
console.log(first); // outputs: 1
console.log(rest); // outputs: [2, 3, 4, 5]
- Short-circuit Evaluation:
Short-circuit evaluation in JavaScript involves logical operators (&& and ||) to create shorter code. It can be used to set default values, validate objects before using them, and more:
let name = user && user.name;
let port = serverPort || 3000;
- Template Literals:
Template literals provide an easy way to interpolate variables and expressions into strings. They are enclosed by backtick () characters instead of quotes:
let name = "John";
console.log(`Hello, ${name}!`); // outputs: Hello, John!
- Arrow Functions:
Arrow functions offer a more concise syntax for writing function expressions. They are ideal for non-method functions and they do not have their own 'this', arguments, super, or new.target:
let hello = () => "Hello World";
console.log(hello()); // outputs: Hello World
- Nullish Coalescing Operator:
The nullish coalescing operator (??) is a logical operator that returns its right-hand side operand when its left-hand side operand is null or undefined, and otherwise returns its left-hand side operand.
let name = null ?? "default name";
console.log(name); // outputs: default name
- Ternary Operator:
The ternary operator is a quicker way to write simple if-else statements. The syntax is: condition ? value_if_true : value_if_false:
let age = 15;
let type = age >= 18 ? "Adult" : "Minor";
console.log(type); // outputs: Minor
- Array Methods (map, reduce, filter):
JavaScript provides powerful array methods like map, reduce, and filter. They are more efficient and elegant to use in comparison to traditional for-loops:
let numbers = [1, 2, 3, 4, 5];
let doubled = numbers.map(n => n * 2); // outputs: [2, 4, 6, 8, 10]
- Async/Await:
Async/Await makes asynchronous code look and behave more like synchronous code. This syntactic sugar on top of promises makes asynchronous code easier to understand and write:
async function fetchUser() {
let response = await fetch('https://api.github.com/users');
let data = await response.json();
console.log(data);
}
fetchUser();
That's all for this post. I hope you found these JavaScript tricks useful. Happy coding!