Why useEffect? no pun intended lol!
Understanding useEffect in React: Why You Can't Just Call a Function A Saturday Morning Realization So it’s a Saturday morning, and I’m basking in the sun, flipping through the last pages of The Psychology of Money by Morgan Housel. I just finished the final chapter. Not gonna lie, I was just floating the whole time because I'm not a US citizen, so understanding their economy with that well-detailed history workflow was very unrelatable. I am in Kenya, so we have completely different economies, but of course, there are some similarities here and there. Anyway, enough about my side quests. I am here to talk about useEffect in React. Reading the last chapter felt like reading useEffect for the first time. When I first encountered it, I didn’t understand why I needed it. Why couldn’t I just call a function inside my component like in regular JavaScript? Say, for example, it's an API call—why not write the function directly in my component? If you’re wondering the same thing, give yourself a little pat on the back because that's a great question. What you need to understand is, React's rendering process is different from the normal execution of JavaScript functions. Let’s break it down. The Problem: Why Not Just Call a Function? In JavaScript, you can normally define and call functions inside your code. So why not just do this inside a React component? function App() { const [count, setCount] = useState(0); const [text, setText] = useState(""); fetch("https://api.example.com/data") .then((response) => response.json()) .then((data) => console.log(data)); return ( setCount((count) => count + 1)}> I've been clicked {count} times setText(e.target.value)} /> ); }
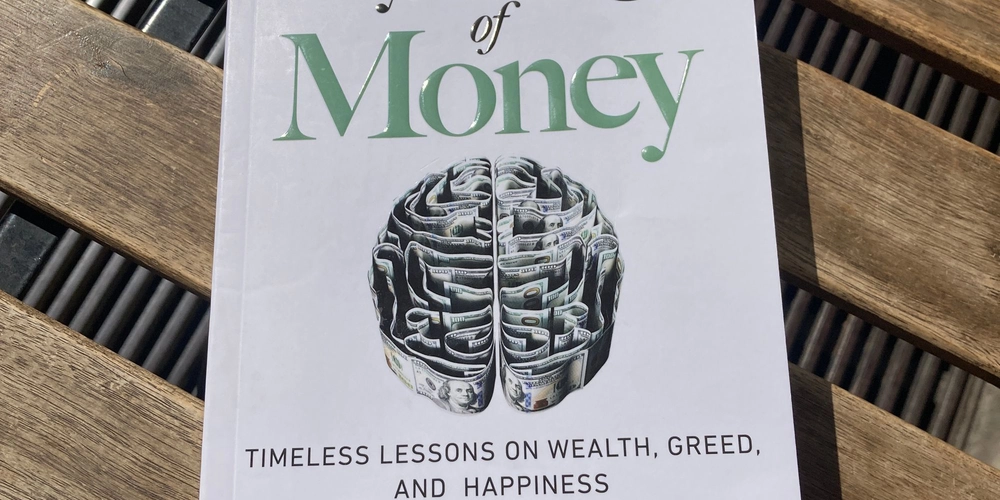
Understanding useEffect in React: Why You Can't Just Call a Function
A Saturday Morning Realization
So it’s a Saturday morning, and I’m basking in the sun, flipping through the last pages of The Psychology of Money by Morgan Housel. I just finished the final chapter. Not gonna lie, I was just floating the whole time because I'm not a US citizen, so understanding their economy with that well-detailed history workflow was very unrelatable. I am in Kenya, so we have completely different economies, but of course, there are some similarities here and there.
Anyway, enough about my side quests. I am here to talk about useEffect
in React. Reading the last chapter felt like reading useEffect
for the first time. When I first encountered it, I didn’t understand why I needed it. Why couldn’t I just call a function inside my component like in regular JavaScript? Say, for example, it's an API call—why not write the function directly in my component? If you’re wondering the same thing, give yourself a little pat on the back because that's a great question. What you need to understand is, React's rendering process is different from the normal execution of JavaScript functions. Let’s break it down.
The Problem: Why Not Just Call a Function?
In JavaScript, you can normally define and call functions inside your code. So why not just do this inside a React component?
function App() {
const [count, setCount] = useState(0);
const [text, setText] = useState("");
fetch("https://api.example.com/data")
.then((response) => response.json())
.then((data) => console.log(data));
return (
<div>
<button onClick={() => setCount((count) => count + 1)}>
I've been clicked {count} times
button>
<input
type="text"
placeholder="Type away..."
value={text}
onChange={(e) => setText(e.target.value)}
/>
div>
);
}