Why Is Profiling Important for Node.js Applications?
When developing Node.js applications, performance issues can often creep in unnoticed, making your application sluggish and inefficient. Profiling is a powerful technique that helps developers analyze and optimize performance bottlenecks. But why is profiling important for Node.js applications? 1. Understanding Node.js Performance Challenges Node.js operates on a single-threaded event loop, which allows it to handle multiple I/O operations efficiently. However, this design has its own set of challenges: Blocking Operations: A single CPU-intensive task can block the entire event loop, affecting the performance of all requests. Memory Leaks: Unoptimized memory usage can cause increased heap allocation, leading to slowdowns or crashes. Garbage Collection Overhead: Poor memory management can lead to excessive garbage collection cycles, negatively impacting response times. Inefficient Code Paths: Nested loops, redundant function calls, and slow database queries can introduce latency. Profiling helps in identifying these issues by providing insights into CPU usage, memory consumption, function execution time, and more. 2. What Is Profiling in Node.js? Profiling is the process of collecting data about an application's runtime behavior to diagnose performance bottlenecks. In Node.js, profiling mainly focuses on: CPU Profiling: Analyzing which functions consume the most CPU time. Memory Profiling: Identifying memory leaks and excessive memory allocations. Event Loop Profiling: Monitoring how efficiently the event loop processes tasks. Async Profiling: Understanding how asynchronous tasks impact application performance. These insights help developers optimize their code for speed and efficiency. 3. Key Benefits of Profiling Node.js Applications A. Identifying Bottlenecks Profiling highlights which parts of the code are consuming excessive CPU time or memory, allowing developers to optimize those sections. B. Improving Scalability A well-profiled application handles more concurrent users efficiently, making it easier to scale. C. Reducing Response Times By eliminating performance bottlenecks, applications become faster, improving user experience. D. Optimizing Resource Usage Profiling ensures that CPU, memory, and I/O resources are utilized effectively, leading to cost savings in cloud environments. E. Preventing Application Crashes Memory leaks and CPU overloads can cause applications to crash. Profiling helps detect these issues early. 4. Node.js Profiling Techniques There are several ways to profile a Node.js application, each serving a specific purpose. A. Using Node.js Built-in Profiler Node.js has a built-in profiler that works with V8 (Google’s JavaScript engine). You can use it as follows: node --prof app.js This generates a isolate-0xnnnnnnnnnnnn-v8.log file, which you can analyze using the node --prof-process command: node --prof-process isolate-0xnnnnnnnnnnnn-v8.log > processed.txt This output helps identify slow functions in the application. B. Using Chrome DevTools for CPU Profiling You can profile Node.js applications using Chrome DevTools: Start the Node.js application with the --inspect flag: node --inspect app.js Open Chrome and go to chrome://inspect Click on Open dedicated DevTools for Node Go to the Profiler tab and start recording Analyze the recorded performance data This provides a graphical view of function execution times. C. Using Node Clinic Node Clinic is an excellent tool for profiling Node.js applications. It offers multiple tools like: Clinic Doctor: Analyzes the application’s health. Clinic Flame: Generates flame graphs for CPU profiling. Clinic Bubbleprof: Visualizes async operations. To install Node Clinic: npm install -g clinic Run it with: clinic doctor -- node app.js This provides a report with performance recommendations. D. Using Heap Snapshots for Memory Profiling Memory leaks are a common issue in Node.js applications. To detect memory leaks, you can generate heap snapshots using Chrome DevTools. Start the Node.js application with the --inspect flag. Open Chrome DevTools (chrome://inspect). Go to the Memory tab. Capture heap snapshots before and after executing operations. Compare heap snapshots to identify memory leaks. 5. Common Node.js Performance Issues and How Profiling Helps Let’s look at some common Node.js performance problems and how profiling helps resolve them. A. Blocking the Event Loop Problem: A CPU-heavy operation (e.g., large JSON parsing) blocks the event loop, delaying all other requests. How Profiling Helps: CPU profiling reveals functions consuming the most execution time. The solution is to offload heavy tasks to worker threads. Example: const { Worker } = require('worker_threads'); const worke
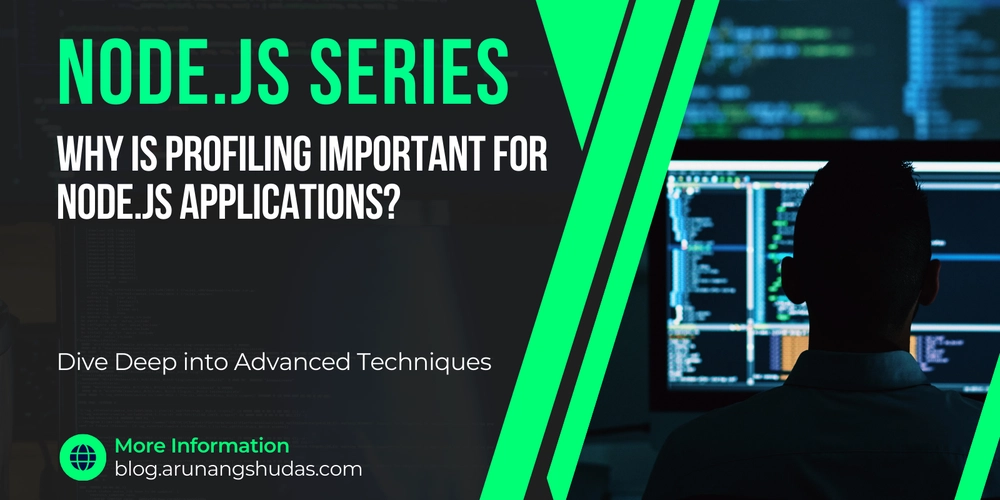
When developing Node.js applications, performance issues can often creep in unnoticed, making your application sluggish and inefficient. Profiling is a powerful technique that helps developers analyze and optimize performance bottlenecks. But why is profiling important for Node.js applications?
1. Understanding Node.js Performance Challenges
Node.js operates on a single-threaded event loop, which allows it to handle multiple I/O operations efficiently. However, this design has its own set of challenges:
- Blocking Operations: A single CPU-intensive task can block the entire event loop, affecting the performance of all requests.
- Memory Leaks: Unoptimized memory usage can cause increased heap allocation, leading to slowdowns or crashes.
- Garbage Collection Overhead: Poor memory management can lead to excessive garbage collection cycles, negatively impacting response times.
- Inefficient Code Paths: Nested loops, redundant function calls, and slow database queries can introduce latency.
Profiling helps in identifying these issues by providing insights into CPU usage, memory consumption, function execution time, and more.
2. What Is Profiling in Node.js?
Profiling is the process of collecting data about an application's runtime behavior to diagnose performance bottlenecks. In Node.js, profiling mainly focuses on:
- CPU Profiling: Analyzing which functions consume the most CPU time.
- Memory Profiling: Identifying memory leaks and excessive memory allocations.
- Event Loop Profiling: Monitoring how efficiently the event loop processes tasks.
- Async Profiling: Understanding how asynchronous tasks impact application performance.
These insights help developers optimize their code for speed and efficiency.
3. Key Benefits of Profiling Node.js Applications
A. Identifying Bottlenecks
Profiling highlights which parts of the code are consuming excessive CPU time or memory, allowing developers to optimize those sections.
B. Improving Scalability
A well-profiled application handles more concurrent users efficiently, making it easier to scale.
C. Reducing Response Times
By eliminating performance bottlenecks, applications become faster, improving user experience.
D. Optimizing Resource Usage
Profiling ensures that CPU, memory, and I/O resources are utilized effectively, leading to cost savings in cloud environments.
E. Preventing Application Crashes
Memory leaks and CPU overloads can cause applications to crash. Profiling helps detect these issues early.
4. Node.js Profiling Techniques
There are several ways to profile a Node.js application, each serving a specific purpose.
A. Using Node.js Built-in Profiler
Node.js has a built-in profiler that works with V8 (Google’s JavaScript engine). You can use it as follows:
node --prof app.js
This generates a isolate-0xnnnnnnnnnnnn-v8.log
file, which you can analyze using the node --prof-process
command:
node --prof-process isolate-0xnnnnnnnnnnnn-v8.log > processed.txt
This output helps identify slow functions in the application.
B. Using Chrome DevTools for CPU Profiling
You can profile Node.js applications using Chrome DevTools:
- Start the Node.js application with the
--inspect
flag:
node --inspect app.js
- Open Chrome and go to
chrome://inspect
- Click on Open dedicated DevTools for Node
- Go to the Profiler tab and start recording
- Analyze the recorded performance data
This provides a graphical view of function execution times.
C. Using Node Clinic
Node Clinic is an excellent tool for profiling Node.js applications. It offers multiple tools like:
- Clinic Doctor: Analyzes the application’s health.
- Clinic Flame: Generates flame graphs for CPU profiling.
- Clinic Bubbleprof: Visualizes async operations.
To install Node Clinic:
npm install -g clinic
Run it with:
clinic doctor -- node app.js
This provides a report with performance recommendations.
D. Using Heap Snapshots for Memory Profiling
Memory leaks are a common issue in Node.js applications. To detect memory leaks, you can generate heap snapshots using Chrome DevTools.
- Start the Node.js application with the
--inspect
flag. - Open Chrome DevTools (
chrome://inspect
). - Go to the Memory tab.
- Capture heap snapshots before and after executing operations.
Compare heap snapshots to identify memory leaks.
5. Common Node.js Performance Issues and How Profiling Helps
Let’s look at some common Node.js performance problems and how profiling helps resolve them.
A. Blocking the Event Loop
Problem: A CPU-heavy operation (e.g., large JSON parsing) blocks the event loop, delaying all other requests.
How Profiling Helps:
CPU profiling reveals functions consuming the most execution time. The solution is to offload heavy tasks to worker threads.
Example:
const { Worker } = require('worker_threads');
const worker = new Worker('./heavyTask.js');
worker.on('message', (result) => {
console.log('Processed:', result);
});
B. Memory Leaks
Problem: Unreleased objects in memory cause increasing heap size, leading to slow performance or crashes.
How Profiling Helps:
Heap snapshots help track memory allocation. If certain objects persist across multiple snapshots, they might be causing a memory leak.
Example Fix:
let cache = new Map();
setInterval(() => cache.clear(), 60000); // Clear cache periodically
C. Slow Database Queries
Problem: Unoptimized database queries cause high response times.
How Profiling Helps:
Profiling tools like clinic flame
highlight database calls that take too long.
Example Fix:
Use indexing and connection pooling:
db.collection('users').createIndex({ email: 1 });
D. Inefficient Async Operations
Problem: Nested asynchronous calls (callback hell) cause performance degradation.
How Profiling Helps:
clinic bubbleprof
helps visualize async operations and find inefficiencies.
Example Fix:
Use async/await instead of callbacks:
async function fetchData() {
const data = await db.collection('users').find().toArray();
return data;
}
6. Best Practices for Profiling Node.js Applications
- Profile in a Staging Environment: Running profilers in production may impact performance.
- Use Multiple Profiling Tools: Each tool provides unique insights.
- Automate Performance Testing: Use tools like Artillery or K6 for load testing.
- Analyze Trends: Regular profiling helps detect performance degradation over time.
Conclusion
Profiling is crucial for optimizing Node.js applications. It helps identify performance bottlenecks, reduce response times, and improve scalability.
You may also like:
Read more blogs from Here
Share your experiences in the comments, and let’s discuss how to tackle them!
Follow me on Linkedin