What is the most concise way to test a boolean condition with many AND clauses?
I have a function which returns the availability of a module, where the module is only available if multiple conditions are all met. The code looks like this: bool isShipAvailable() { bool isAvailable; isAvailable = this->areSystemsCalibrated; isAvailable = isAvailable && this->areEnginesFunctional; isAvailable = isAvailable && this->isWarpDriveOnline; isAvailable = isAvailable && this->isFluxCapacitorOnline; isAvailable = isAvailable && this->areStabilizersOnline; return isAvailable; } I'm trying to test this code without creating something large or difficult to maintain. I know I could write tests for all permutations, but would it be acceptable to test the case where all conditions are true and then test each scenario where only one condition is false? Would that be making too many assumptions about the implementation in the tests? Edit: I'm not asking how to refactor the boolean logic to be concise, but rather what the shortest way to test every permutation of that logic would be. The details of the implementation itself are not terribly important.
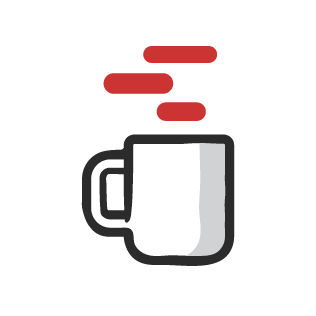
I have a function which returns the availability of a module, where the module is only available if multiple conditions are all met. The code looks like this:
bool isShipAvailable() {
bool isAvailable;
isAvailable = this->areSystemsCalibrated;
isAvailable = isAvailable && this->areEnginesFunctional;
isAvailable = isAvailable && this->isWarpDriveOnline;
isAvailable = isAvailable && this->isFluxCapacitorOnline;
isAvailable = isAvailable && this->areStabilizersOnline;
return isAvailable;
}
I'm trying to test this code without creating something large or difficult to maintain. I know I could write tests for all permutations, but would it be acceptable to test the case where all conditions are true
and then test each scenario where only one condition is false
? Would that be making too many assumptions about the implementation in the tests?
Edit: I'm not asking how to refactor the boolean logic to be concise, but rather what the shortest way to test every permutation of that logic would be. The details of the implementation itself are not terribly important.