What is the difference between React Router and React Router DOM?
react-router and react-router-dom are libraries used for routing in React applications. They allow you to create single-page applications (SPAs) where navigation between different views or pages happens without a full page reload. Here's a detailed explanation of both: 1. What is react-router? react-router is the core routing library for React applications. It provides the basic fundamental routing functionality, such as matching URLs to components and managing navigation. but it does not include anything specific to web applications. It contains essential components like Routes, Route, and Outlet. It is framework-agnostic, meaning it does not just depend on the web. but it can be used with React for web applications, React Native for mobile apps, or even other environments. You don't typically install or use react-router directly in web projects. This package is used as a foundation for other libraries like react-router-dom (for web apps) and react-router-native (for React Native apps). Key Features: Declarative routing (defining routes using components). Dynamic routing (routes can be added or removed at runtime). Nested routes (routes within routes). Programmatic navigation (e.g., redirecting users programmatically). Use Case: react-router is typically used as a dependency for other routing libraries like react-router-dom (for web) or react-router-native (for mobile). 2. What is react-router-dom? react-router-dom is the official routing library for React web applications. It is built on top of react-router and provides additional components and hooks specifically designed for web development. It provides additional web-related components like BrowserRouter, HashRouter, Link, NavLink, and hooks like useNavigate, useParams, and useLocation. Key Features: BrowserRouter: Uses the HTML5 History API (pushState, replaceState, etc.) to keep the UI in sync with the URL. HashRouter: Uses the hash portion of the URL (e.g., #/about) for routing, which is useful for older browsers or static deployments. Link and NavLink: Components for creating navigation links. useHistory (in older versions, replaced with useNavigate in v6) useNavigate: A hook for programmatic navigation. useParams: A hook for accessing route parameters (e.g., :id in /users/:id). useLocation: A hook for accessing the current URL location. Use Case: react-router-dom is used for building web applications with client-side routing. It is the most common choice for React web apps. 3. Key Differences Between react-router and react-router-dom Feature react-router react-router-dom Purpose Core routing library for React Web-specific routing library for React apps Web/ Browser Support No direct support Supports web routing (BrowserRouter, HashRouter) Components No UI components. Basic essential routing components like Routes, Route and Outlet. Web-specific components like BrowserRouter, Link, NavLink etc. Hooks Basic routing hooks Web-specific hooks like useNavigate, useLocation, etc. Environment Works with React, React Native, etc. Specifically for web applications Dependency Independent Depends on react-router Installation To use react-router-dom in your project, install it via npm or yarn: npm: npm install react-router-dom or with yarn: yarn add react-router-dom Example Usage of react-router-dom Basic Routing Setup: import React from 'react'; import { BrowserRouter as Router, Routes, Route, Link } from 'react-router-dom'; import Home from './Home'; import About from './About'; import UserProfile from './UserProfile'; function App() { return ( Home About User Profile ); } export default App; Using useNavigate for Programmatic Navigation: import React from 'react'; import { useNavigate } from 'react-router-dom'; function LoginButton() { const navigate = useNavigate(); const handleLogin = () => { // Perform login logic navigate('/dashboard'); // Redirect to the dashboard after login }; return Login; } export default LoginButton; Using useParams to Access Route Parameters: import React from 'react'; import { useParams } from 'react-router-dom'; function UserProfile() { const { id } = useParams(); // Access the `id` parameter from the URL return User ID: {id}; } export default UserProfile; 4. How They Work Together? react-router-dom is built on top of react-router. It extends the core functionality of react-router with web-specific features. When you install react-router-dom, it automatically includes react-router as a dependency. 5. Which One Should You Use? If you are building a React web application, use react-router-dom. If you are creating a library or a React Native app, use react-router (along with react-router-native for
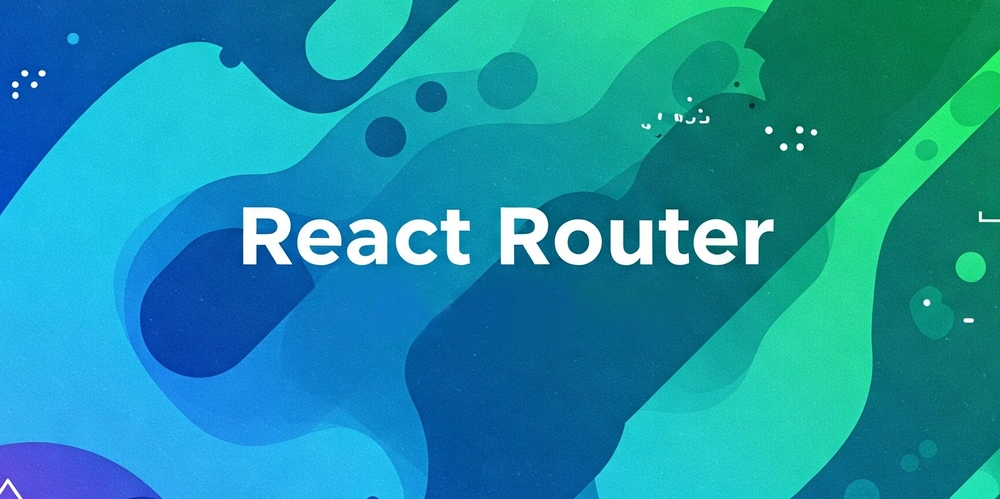
react-router
and react-router-dom
are libraries used for routing in React applications. They allow you to create single-page applications (SPAs) where navigation between different views or pages happens without a full page reload. Here's a detailed explanation of both:
1. What is react-router
?
-
react-router
is the core routing library for React applications. - It provides the basic fundamental routing functionality, such as matching URLs to components and managing navigation. but it does not include anything specific to web applications.
- It contains essential components like
Routes
,Route
, andOutlet
. - It is framework-agnostic, meaning it does not just depend on the web. but it can be used with React for web applications, React Native for mobile apps, or even other environments.
- You don't typically install or use
react-router
directly in web projects. - This package is used as a foundation for other libraries like
react-router-dom
(for web apps) andreact-router-native
(for React Native apps). -
Key Features:
- Declarative routing (defining routes using components).
- Dynamic routing (routes can be added or removed at runtime).
- Nested routes (routes within routes).
- Programmatic navigation (e.g., redirecting users programmatically).
-
Use Case:
-
react-router
is typically used as a dependency for other routing libraries likereact-router-dom
(for web) orreact-router-native
(for mobile).
-
2. What is react-router-dom
?
-
react-router-dom
is the official routing library for React web applications. It is built on top ofreact-router
and provides additional components and hooks specifically designed for web development. - It provides additional web-related components like
BrowserRouter
,HashRouter
,Link
,NavLink
, and hooks likeuseNavigate
,useParams
, anduseLocation
. -
Key Features:
-
BrowserRouter
: Uses the HTML5 History API (pushState
,replaceState
, etc.) to keep the UI in sync with the URL. -
HashRouter
: Uses the hash portion of the URL (e.g.,#/about
) for routing, which is useful for older browsers or static deployments. -
Link
andNavLink
: Components for creating navigation links. -
useHistory
(in older versions, replaced withuseNavigate
in v6) -
useNavigate
: A hook for programmatic navigation. -
useParams
: A hook for accessing route parameters (e.g.,:id
in/users/:id
). -
useLocation
: A hook for accessing the current URL location.
-
-
Use Case:
-
react-router-dom
is used for building web applications with client-side routing. It is the most common choice for React web apps.
-
3. Key Differences Between react-router
and react-router-dom
Feature | react-router |
react-router-dom |
---|---|---|
Purpose | Core routing library for React | Web-specific routing library for React apps |
Web/ Browser Support | No direct support | Supports web routing (BrowserRouter , HashRouter ) |
Components | No UI components. Basic essential routing components like Routes , Route and Outlet . |
Web-specific components like BrowserRouter , Link , NavLink etc. |
Hooks | Basic routing hooks | Web-specific hooks like useNavigate , useLocation , etc. |
Environment | Works with React, React Native, etc. | Specifically for web applications |
Dependency | Independent | Depends on react-router
|
Installation
To use react-router-dom
in your project, install it via npm or yarn:
npm:
npm install react-router-dom
or with yarn:
yarn add react-router-dom
Example Usage of react-router-dom
Basic Routing Setup:
import React from 'react';
import { BrowserRouter as Router, Routes, Route, Link } from 'react-router-dom';
import Home from './Home';
import About from './About';
import UserProfile from './UserProfile';
function App() {
return (
<Router>
<nav>
<Link to="/">HomeLink>
<Link to="/about">AboutLink>
<Link to="/user/123">User ProfileLink>
nav>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
<Route path="/user/:id" element={<UserProfile />} />
Routes>
Router>);
}
export default App;
Using useNavigate
for Programmatic Navigation:
import React from 'react';
import { useNavigate } from 'react-router-dom';
function LoginButton() {
const navigate = useNavigate();
const handleLogin = () => {
// Perform login logic
navigate('/dashboard'); // Redirect to the dashboard after login
};
return <button onClick={handleLogin}>Loginbutton>;
}
export default LoginButton;
Using useParams
to Access Route Parameters:
import React from 'react';
import { useParams } from 'react-router-dom';
function UserProfile() {
const { id } = useParams(); // Access the `id` parameter from the URL
return <div>User ID: {id}div>;
}
export default UserProfile;
4. How They Work Together?
-
react-router-dom
is built on top ofreact-router
. It extends the core functionality ofreact-router
with web-specific features. - When you install
react-router-dom
, it automatically includesreact-router
as a dependency.
5. Which One Should You Use?
- If you are building a React web application, use
react-router-dom
. - If you are creating a library or a React Native app, use
react-router
(along withreact-router-native
for mobile apps). - If you're creating a custom routing solution, you might need
react-router
internally.
6. What Has Changed in 2025?
- React Router v6+ is now the standard, focusing on component-based routing.
- Hooks like
useHistory
(deprecated) are fully replaced byuseNavigate
. -
react-router
is rarely used directly in web projects, whilereact-router-dom
is the main package for web routing.
Summary
-
react-router
: The core routing library for React. -
react-router-dom
: The web-specific routing library built on top ofreact-router
. - Use
react-router-dom
for web applications to take advantage of web-specific features likeBrowserRouter
,Link
, anduseNavigate
.
If you're building a web application, you only need to install and use react-router-dom
. It will automatically include react-router
as a dependency.