What is Javascript?
As we all know that JavaScript is one of the widely used programming languages across all domains, such as web, mobile, game development etc. till date now. But why it becomes so much popular among all the existing languages? Because it is easy to learn, dynamic, high-level, interpreted, light-weight scripting programming language that allows to add interactivity on the web page. Let's break it down what all these keywords mean. Dynamic means we can run JavaScript code in user's local computer as well as on the server. a) We can dynamically generate or update any html element like p, ul, li, div etc. tags inside client's browser using DOM's API (which is browser's API resides in the browser). b) Also we cam dynamically generate new content on the server side by writing application logic e.g., pulling data from a database. High-level means it is human readable language. Interpreted means the code starts running from top to bottom and when we run that code, we get immediate human readable result. We don't need to translate the code into machine code before running like in C, C++ etc. How to use JavaScript in HTML? There are 3 ways, we can place JavaScript code in html page depending upon use cases. 1. Inline JavaScript - We can use it inside HTML tags by using JavaScript attributes. E.g., Click me! 2. Internal JavaScript - with Script tag We can add script tag at the bottom of your body means just before your closing body tag or at the top before closing head tag function display(){ console.log("I am in the script tag") } 3 External JavaScript - Just like we use link tag for linking stylesheet to html, use script tag for attaching JavaScript file to html. For this, create new file with extension .js in the same or different directory path. Add that file path in the html page using src (source) attribute. This is important because it tells the browser to fetch data from "xyz.js" file. Otherwise js file code won't run. Benefits: Platform independent - We can run JavaScript code in any machine. It is supported by all major browsers and enabled by default. Many JavaScript open source libraries and frameworks are available in the market. e.g. a) We can use Chart.js library to display static or dynamic data in bar, line, pie charts or maps. b) Reactjs, Vuejs or Angularjs web frameworks simplifies the web development and makes developer's life ease. c) React Native is another framework is used for building Android, iOS mobile apps. d) Using Nodejs framework, we can run JavaScript code outside of the browser. 3.Client-side validations - Before submitting the form and send data to server, JavaScript quickly checks whether user have entered a 10-digit number for the mobile phone field or not. Because of it server load reduces by performing logical operations in the client side itself. 4.Single threaded - JavaScript executes one task at a time. However with the help of event loop concept, it can handle multiple tasks simultaneously. 5.JavaScript is synchronous by default, meaning it executes code line by line in a sequential manner. However, it can handle asynchronous tasks like fetching data from database using features like callbacks, Promises, and async/await. Limitations: Weakly typed language - JavaScript does not allow the programmer to define the variable type. As a result, you can get unexpected results, such as 1 + 1 + '1' = '21' '1' + 1 + 1 = '111'
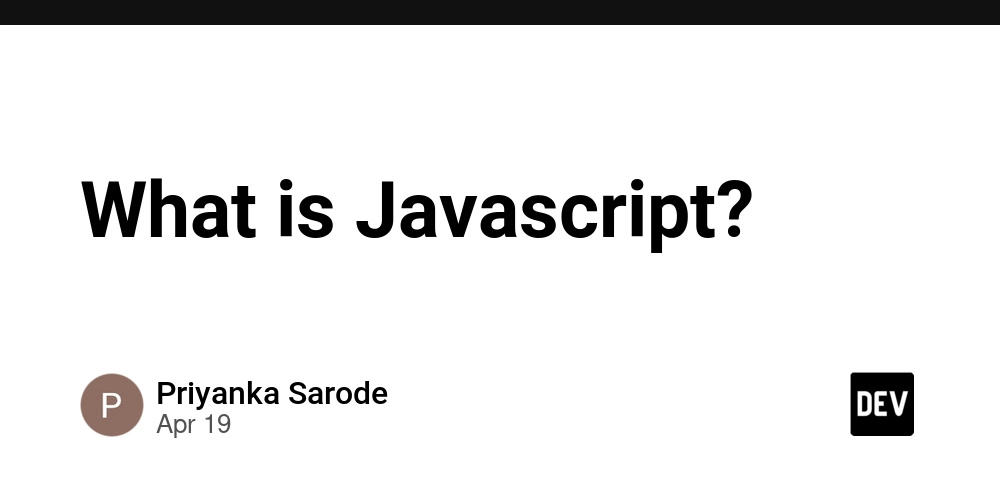
As we all know that JavaScript is one of the widely used programming languages across all domains, such as web, mobile, game development etc. till date now.
But why it becomes so much popular among all the existing languages?
Because it is easy to learn, dynamic, high-level, interpreted, light-weight scripting programming language that allows to add interactivity on the web page.
Let's break it down what all these keywords mean.
- Dynamic means we can run JavaScript code in user's local computer as well as on the server.
- a) We can dynamically generate or update any html element like p, ul, li, div etc. tags inside client's browser using DOM's API (which is browser's API resides in the browser).
- b) Also we cam dynamically generate new content on the server side by writing application logic e.g., pulling data from a database.
- High-level means it is human readable language.
- Interpreted means the code starts running from top to bottom and when we run that code, we get immediate human readable result. We don't need to translate the code into machine code before running like in C, C++ etc.
How to use JavaScript in HTML?
There are 3 ways, we can place JavaScript code in html page depending upon use cases.
- 1. Inline JavaScript - We can use it inside HTML tags by using JavaScript attributes. E.g.,
- 2. Internal JavaScript - with Script tag We can add script tag at the bottom of your body means just before your closing body tag or at the top before closing head tag
- 3 External JavaScript - Just like we use link tag for linking stylesheet to html, use script tag for attaching JavaScript file to html. For this, create new file with extension .js in the same or different directory path. Add that file path in the html page using src (source) attribute. This is important because it tells the browser to fetch data from "xyz.js" file. Otherwise js file code won't run.
Benefits:
- Platform independent - We can run JavaScript code in any machine. It is supported by all major browsers and enabled by default.
- Many JavaScript open source libraries and frameworks are available in the market. e.g.
a) We can use Chart.js library to display static or dynamic data in bar, line, pie charts or maps.
b) Reactjs, Vuejs or Angularjs web frameworks simplifies the web development and makes developer's life ease.
c) React Native is another framework is used for building Android, iOS mobile apps.
d) Using Nodejs framework, we can run JavaScript code outside of the browser.
3.Client-side validations - Before submitting the form and send data to server, JavaScript quickly checks whether user have entered a 10-digit number for the mobile phone field or not. Because of it server load reduces by performing logical operations in the client side itself.
4.Single threaded - JavaScript executes one task at a time. However with the help of event loop concept, it can handle multiple tasks simultaneously.
5.JavaScript is synchronous by default, meaning it executes code line by line in a sequential manner. However, it can handle asynchronous tasks like fetching data from database using features like callbacks, Promises, and async/await.
Limitations:
- Weakly typed language - JavaScript does not allow the programmer to define the variable type. As a result, you can get unexpected results, such as
1 + 1 + '1' = '21'
'1' + 1 + 1 = '111'