WebSockets : Real-Time Magic Beyond REST
What are WebSockets? (We will cover everything in very short and but in detail.) WebSockets provide a persistent, full-duplex communication channel between client and server. Once the handshake is done over HTTP, the connection upgrades to WebSocket. Why WebSockets? Because REST is slow for certain use cases. Think: Real-time dashboards Chat apps Live collaboration tools (like Google Docs) Things You Might Not Know About WebSockets They’re Not Just for Chat Stock trading platforms Server log monitors WebSockets Need a Heartbeat Connections can silently die due to firewalls or network issues we have to send message in interval. Eg-: setInterval(() => { socket.send(JSON.stringify({ type: 'ping' })); }, 30000); No Built-In Auth After Handshake Unlike HTTP headers, you can't send custom headers once the WS connection is established. You’ll have to Include tokens in the query string during the handshake. Socket.io Or WebSocket Most devs assume Socket.IO is just a wrapper for WebSockets. But it’s more like a custom protocol that can use WebSocket under the hood. Big difference. Example websocket code -: Backend => const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 8080 }); wss.on('connection', (ws) => { console.log('A new client connected'); ws.on('message', (message) => { console.log('Received: %s', message); }); ws.send('Hello from server'); }); Frontend=> import React, { useState, useEffect } from 'react'; const WebSocketClient = () => { const [socket, setSocket] = useState(null); const [message, setMessage] = useState(''); const [messages, setMessages] = useState([]); useEffect(() => { const ws = new WebSocket('ws://localhost:8080'); // Connect to server setSocket(ws); ws.onmessage = (event) => { setMessages((prev) => [...prev, `Server: ${event.data}`]); }; return () => { ws.close(); }; }, []); const sendMessage = () => { if (socket && message) { socket.send(message); // Send message to server setMessages((prev) => [...prev, `Client: ${message}`]); setMessage(''); } }; return ( setMessage(e.target.value)} /> Send {messages.map((msg, idx) => {msg})} ); }; export default WebSocketClient; Easy code above let me know if you have any doubt or correction. Thank You and Stay real-time, devs. Just like webSocket. ⏱️
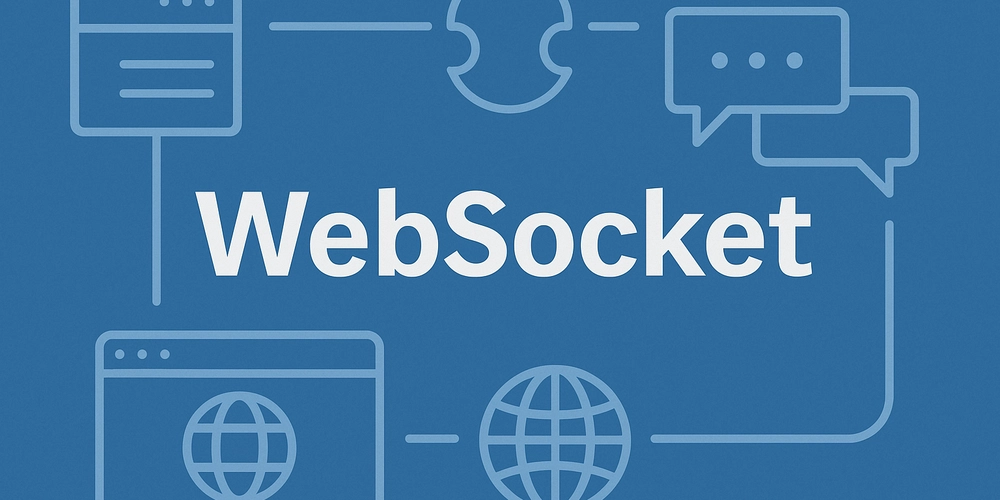
What are WebSockets?
(We will cover everything in very short and but in detail.)
WebSockets provide a persistent, full-duplex communication channel between client and server. Once the handshake is done over HTTP, the connection upgrades to WebSocket.
Why WebSockets?
Because REST is slow for certain use cases. Think:
- Real-time dashboards
- Chat apps
- Live collaboration tools (like Google Docs)
Things You Might Not Know About WebSockets
They’re Not Just for Chat
- Stock trading platforms
- Server log monitors
WebSockets Need a Heartbeat
- Connections can silently die due to firewalls or network issues we have to send message in interval. Eg-:
setInterval(() => {
socket.send(JSON.stringify({ type: 'ping' }));
}, 30000);
No Built-In Auth After Handshake
- Unlike HTTP headers, you can't send custom headers once the WS connection is established. You’ll have to Include tokens in the query string during the handshake.
Socket.io Or WebSocket
Most devs assume Socket.IO is just a wrapper for WebSockets. But it’s more like a custom protocol that can use WebSocket under the hood. Big difference.
Example websocket code -:
Backend =>
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', (ws) => {
console.log('A new client connected');
ws.on('message', (message) => {
console.log('Received: %s', message);
});
ws.send('Hello from server');
});
Frontend=>
import React, { useState, useEffect } from 'react';
const WebSocketClient = () => {
const [socket, setSocket] = useState(null);
const [message, setMessage] = useState('');
const [messages, setMessages] = useState([]);
useEffect(() => {
const ws = new WebSocket('ws://localhost:8080'); // Connect to server
setSocket(ws);
ws.onmessage = (event) => {
setMessages((prev) => [...prev, `Server: ${event.data}`]);
};
return () => {
ws.close();
};
}, []);
const sendMessage = () => {
if (socket && message) {
socket.send(message); // Send message to server
setMessages((prev) => [...prev, `Client: ${message}`]);
setMessage('');
}
};
return (
setMessage(e.target.value)} />
{messages.map((msg, idx) => - {msg}
)}
);
};
export default WebSocketClient;
Easy code above let me know if you have any doubt or correction.
Thank You and Stay real-time, devs. Just like webSocket. ⏱️