vs-code commands to create a dotnet project
Peace I will walk you through the steps to create a console application using Visual Studio Code (VS Code), manage projects within a solution, add packages like Entity Framework, and explore additional features that enhance your development experience. Prerequisites Before you begin, ensure you have the following installed: .NET SDK: Download and install the .NET SDK from the .NET website. Visual Studio Code: Download and install VS Code from the official website. C# Dev Kit extension: Install the C# Dev Kit extension from the Extensions Marketplace in VS Code. Step 1: List Available Project Templates To see a list of available project templates, you can use the following command: dotnet new --list This command will display all the templates available for creating different types of projects, including console applications, web applications, class libraries.. You will take the names that are in the short name column. Step 2: Create a New Console Application Open Visual Studio Code: Launch VS Code. Open the Integrated Terminal: You can open the terminal by selecting Terminal from the top menu and then New Terminal, or by using the shortcut Ctrl + ` (backtick). Create a New Console Application: In the terminal, navigate to the directory where you want to create your project. Use the following command to create a new console application: dotnet new console -n MyConsoleApp This command creates a new folder named MyConsoleApp containing the console application. Navigate to the Project Directory: cd MyConsoleApp Step 3: Create a Solution and Add the Project Create a New Solution: If you want to manage multiple projects, you can create a solution. Navigate to the parent directory where you want to create the solution and run: Navigate back to the parent Directory: cd .. Create the solution: dotnet new sln -n MySolution This creates a new solution file named MySolution.sln. Add the Console Application to the Solution: Navigate back to the project directory and add the console application to the solution: dotnet sln add MyConsoleApp/MyConsoleApp.csproj Step 4: Add a Package to the Project To add a package, such as Entity Framework Core, you can use the dotnet add package command. Here’s how to do it: Install Entity Framework Core: In the terminal, run the following command: dotnet add package Microsoft.EntityFrameworkCore This command will add the Entity Framework Core package to your project. Install Additional Packages: If you need to use a database provider (e.g., SQL Server), you can install the corresponding package: dotnet add package Microsoft.EntityFrameworkCore.SqlServer Step 5: Write Some Code Open the Program.cs file in the MyConsoleApp directory and modify it to include some basic functionality. For example, you can add code to connect to a database using Entity Framework Core: using System; using Microsoft.EntityFrameworkCore; namespace MyConsoleApp { public class MyDbContext : DbContext { public DbSet Products { get; set; } } public class Product { public int Id { get; set; } public string Name { get; set; } } class Program { static void Main(string[] args) { Console.WriteLine("Hello, World!"); // Example of using Entity Framework Core using (var context = new MyDbContext()) { // Database operations can be performed here } } } } Step 6: Run the Application To run your console application, use the following command in the terminal: dotnet run This command will build and execute your application, displaying the output in the terminal. For just building the project and not running it, just use: dotnet build Additional Features 1. Debugging You can debug your console application in VS Code: Set breakpoints by clicking in the left margin next to the line numbers in your code. Open the Debug panel by clicking on the Debug icon in the Activity Bar or pressing Ctrl + Shift + D. Click the green play button (Start Debugging) or press F5 to start debugging. Check this for more details about debugging setup: https://learn.microsoft.com/en-us/dotnet/core/tutorials/debugging-with-visual-studio-code 2. Managing Dependencies 3. Version Control If you are using Git for version control, you can easily initialize a Git repository for your console application and manage your code changes: Initialize a Git Repository: In the terminal, run the following command in your project directory: git init Add Files to the Repository: Stage your files for the initial commit: git add . Commit Your Changes: Commit the staged files with a message: git commit -m "Ini
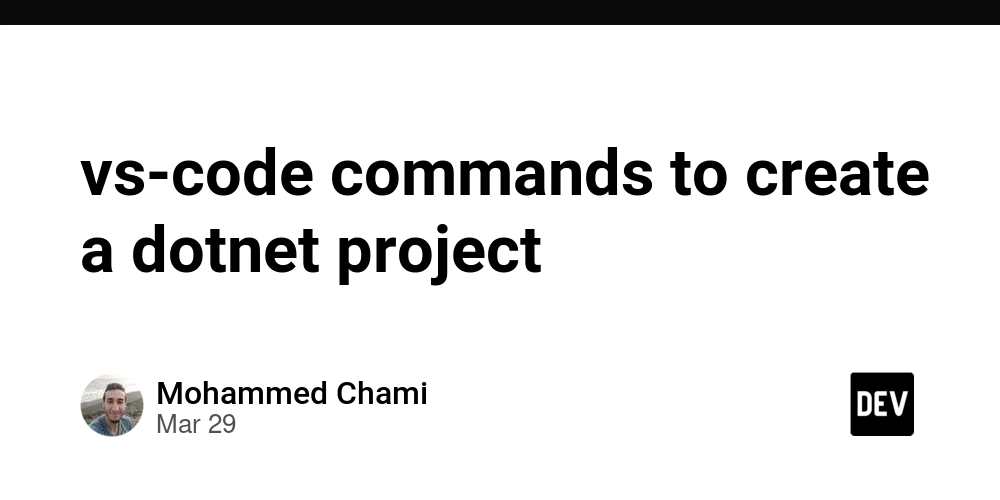
Peace
I will walk you through the steps to create a console application using Visual Studio Code (VS Code), manage projects within a solution, add packages like Entity Framework, and explore additional features that enhance your development experience.
Prerequisites
Before you begin, ensure you have the following installed:
- .NET SDK: Download and install the .NET SDK from the .NET website.
- Visual Studio Code: Download and install VS Code from the official website.
- C# Dev Kit extension: Install the C# Dev Kit extension from the Extensions Marketplace in VS Code.
Step 1: List Available Project Templates
To see a list of available project templates, you can use the following command:
dotnet new --list
This command will display all the templates available for creating different types of projects, including console applications, web applications, class libraries..
You will take the names that are in the short name column.
Step 2: Create a New Console Application
- Open Visual Studio Code: Launch VS Code.
-
Open the Integrated Terminal: You can open the terminal by selecting
Terminal
from the top menu and thenNew Terminal
, or by using the shortcutCtrl + `
(backtick). - Create a New Console Application: In the terminal, navigate to the directory where you want to create your project. Use the following command to create a new console application:
dotnet new console -n MyConsoleApp
This command creates a new folder named MyConsoleApp
containing the console application.
- Navigate to the Project Directory:
cd MyConsoleApp
Step 3: Create a Solution and Add the Project
- Create a New Solution: If you want to manage multiple projects, you can create a solution. Navigate to the parent directory where you want to create the solution and run:
Navigate back to the parent Directory:
cd ..
Create the solution:
dotnet new sln -n MySolution
This creates a new solution file named MySolution.sln
.
- Add the Console Application to the Solution: Navigate back to the project directory and add the console application to the solution:
dotnet sln add MyConsoleApp/MyConsoleApp.csproj
Step 4: Add a Package to the Project
To add a package, such as Entity Framework Core, you can use the dotnet add package
command. Here’s how to do it:
- Install Entity Framework Core: In the terminal, run the following command:
dotnet add package Microsoft.EntityFrameworkCore
This command will add the Entity Framework Core package to your project.
- Install Additional Packages: If you need to use a database provider (e.g., SQL Server), you can install the corresponding package:
dotnet add package Microsoft.EntityFrameworkCore.SqlServer
Step 5: Write Some Code
Open the Program.cs
file in the MyConsoleApp
directory and modify it to include some basic functionality. For example, you can add code to connect to a database using Entity Framework Core:
using System;
using Microsoft.EntityFrameworkCore;
namespace MyConsoleApp
{
public class MyDbContext : DbContext
{
public DbSet<Product> Products { get; set; }
}
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
}
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, World!");
// Example of using Entity Framework Core
using (var context = new MyDbContext())
{
// Database operations can be performed here
}
}
}
}
Step 6: Run the Application
To run your console application, use the following command in the terminal:
dotnet run
This command will build and execute your application, displaying the output in the terminal.
For just building the project and not running it, just use:
dotnet build
Additional Features
1. Debugging
You can debug your console application in VS Code:
- Set breakpoints by clicking in the left margin next to the line numbers in your code.
- Open the Debug panel by clicking on the Debug icon in the Activity Bar or pressing
Ctrl + Shift + D
. - Click the green play button (Start Debugging) or press
F5
to start debugging. Check this for more details about debugging setup: https://learn.microsoft.com/en-us/dotnet/core/tutorials/debugging-with-visual-studio-code
2. Managing Dependencies
3. Version Control
If you are using Git for version control, you can easily initialize a Git repository for your console application and manage your code changes:
- Initialize a Git Repository: In the terminal, run the following command in your project directory:
git init
- Add Files to the Repository: Stage your files for the initial commit:
git add .
- Commit Your Changes: Commit the staged files with a message:
git commit -m "Initial commit"
- Connect to a Remote Repository: If you want to push your code to a remote repository (e.g., GitHub), you can add a remote URL:
git remote add origin https://github.com/username/repository.git
- Push Your Changes: Push your local commits to the remote repository:
git push -u origin master
4. Using Configuration Files
You can manage application settings using configuration files. For example, you can create an appsettings.json
file to store configuration settings for your application.
-
Create
appsettings.json
: In the project directory, create a new file namedappsettings.json
and add your configuration settings:
{
"ConnectionStrings": {
"DefaultConnection": "Server=(localdb)\\mssqllocaldb;Database=MyDatabase;Trusted_Connection=True;"
}
}
-
Read Configuration in Your Application: You can read the configuration settings in your
Program.cs
file using theMicrosoft.Extensions.Configuration
package. First, add the package:
dotnet add package Microsoft.Extensions.Configuration
dotnet add package Microsoft.Extensions.Configuration.Json
Then, modify your Program.cs
to read the configuration:
using System;
using Microsoft.Extensions.Configuration;
namespace MyConsoleApp
{
class Program
{
static void Main(string[] args)
{
var config = new ConfigurationBuilder()
.SetBasePath(AppContext.BaseDirectory)
.AddJsonFile("appsettings.json")
.Build();
var connectionString = config.GetConnectionString("DefaultConnection");
Console.WriteLine($"Connection String: {connectionString}");
}
}
}
5. Testing Your Application
You can add unit tests to your console application to ensure that your code behaves as expected. Here’s how to create a test project:
- Create a Test Project: Navigate to the parent directory of your console application and run:
dotnet new nunit -n MyConsoleApp.Tests
- Add the Test Project to the Solution:
dotnet sln add MyConsoleApp.Tests/MyConsoleApp.Tests.csproj
- Add a Reference to the Console Application:
dotnet add MyConsoleApp.Tests/MyConsoleApp.Tests.csproj reference MyConsoleApp/MyConsoleApp.csproj
Write Tests: Open the
UnitTest1.cs
file in the test project and write your tests using the xUnit framework.Run Tests: You can run your tests using the following command:
dotnet test
check this article for more details: https://learn.microsoft.com/en-us/dotnet/core/testing/unit-testing-csharp-with-nunit
That's how we use vs-code to work with dotnet application.
resource for more dotnet using vs-code: https://code.visualstudio.com/docs/csharp/get-started