Using Streams with Fetch for Efficient Data Processing
Using Streams with Fetch for Efficient Data Processing Table of Contents Introduction Historical Background Understanding Streams What are Streams? Types of Streams in JavaScript The Fetch API Explained How Fetch Works Handling Responses Integrating Streams with Fetch Basic Implementation Handling Large Data Sets Advanced Scenarios and Use Cases Transforming Data on-the-fly Error Handling in Streams Comparative Analysis: Streams vs. Alternatives XHR vs. Fetch with Streams WebSocket vs. Fetch Streams Performance Considerations Streaming vs. Buffering Memory Management Potential Pitfalls Advanced Debugging Techniques Conclusion References and Further Reading Introduction In modern web development, handling large volumes of data efficiently is a challenge many developers face. The advent of the Fetch API introduced an elegant way to handle HTTP requests, but when combined with the concept of streams, it becomes a powerhouse for managing data flows in real-time. This article delves into utilizing streams in conjunction with the Fetch API for efficient data processing, equipping seasoned developers with advanced techniques and nuanced insights. Historical Background The evolution of web technologies has continuously aimed to optimize the interaction between servers and clients. Traditionally, using the XMLHttpRequest (XHR) method was the primary way to make network requests in JavaScript. However, XHR was cumbersome and lacked a promise-based structure, leading to complexities in managing asynchronous requests. With the introduction of the Fetch API in 2015, life became easier for developers. It allows for a more intuitive syntax and promise-based handling of requests. However, as web applications began to handle more significant amounts of data—especially in the context of streaming media, real-time data feeds, and large file transfers—there was a growing need for improved mechanisms to process that data in a more efficient and performant way. This led to the implementation of the Streams API, which can be seamlessly integrated into Fetch operations. Understanding Streams What are Streams? Streams are a set of data processing APIs in JavaScript that allow developers to read and write data in a continuous flow rather than loading it entirely into memory before processing. This allows applications to handle massive datasets more efficiently by processing chunks of data as they are received. Types of Streams in JavaScript Readable Streams: Allow data to be read from a source. Writable Streams: Allow data to be written to a destination. Duplex Streams: Enable both reading and writing. Transform Streams: Modify the data as it is read or written. In the context of Fetch, we primarily work with Readable Streams, which are especially advantageous when streaming responses from a network request. The Fetch API Explained How Fetch Works The Fetch API provides a global fetch() function that starts a network request to a specified resource. In its simplest form, it looks like this: fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error fetching data:', error)); Handling Responses When a Fetch request is made, it returns a promise that resolves to the Response object representing the response to the request. The Response object allows developers to access the status, headers, and body of the response, making it suitable for various use cases. One of the most powerful features of the Response object is its ability to return a ReadableStream via the body property. This is where we can leverage streams to process data efficiently. Integrating Streams with Fetch Basic Implementation Here's how you can integrate streams with Fetch to process data incrementally. This is particularly useful for large datasets. async function fetchAndProcessData(url) { const response = await fetch(url); const reader = response.body.getReader(); let result; while (!(result = await reader.read()).done) { const chunk = result.value; // Uint8Array // Process the chunk, e.g. converting to text const textChunk = new TextDecoder().decode(chunk); console.log(textChunk); } } fetchAndProcessData('https://api.example.com/large-data'); This basic example demonstrates how to read a response body in chunks. As each chunk is received, you can process it immediately without waiting for the entire dataset to download. Handling Large Data Sets Fetching large datasets can lead to excessive memory use if the entire dataset is buffered in memory. Instead, we can take advantage of the streaming capability. async function fetchAndAggregateData(url) { const response =
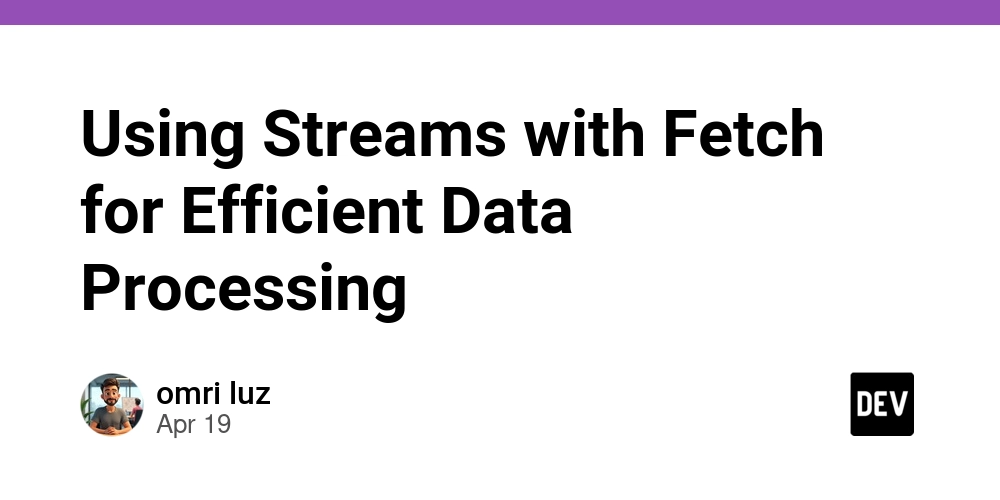
Using Streams with Fetch for Efficient Data Processing
Table of Contents
- Introduction
- Historical Background
-
Understanding Streams
- What are Streams?
- Types of Streams in JavaScript
-
The Fetch API Explained
- How Fetch Works
- Handling Responses
-
Integrating Streams with Fetch
- Basic Implementation
- Handling Large Data Sets
-
Advanced Scenarios and Use Cases
- Transforming Data on-the-fly
- Error Handling in Streams
-
Comparative Analysis: Streams vs. Alternatives
- XHR vs. Fetch with Streams
- WebSocket vs. Fetch Streams
-
Performance Considerations
- Streaming vs. Buffering
- Memory Management
- Potential Pitfalls
- Advanced Debugging Techniques
- Conclusion
- References and Further Reading
Introduction
In modern web development, handling large volumes of data efficiently is a challenge many developers face. The advent of the Fetch API introduced an elegant way to handle HTTP requests, but when combined with the concept of streams, it becomes a powerhouse for managing data flows in real-time. This article delves into utilizing streams in conjunction with the Fetch API for efficient data processing, equipping seasoned developers with advanced techniques and nuanced insights.
Historical Background
The evolution of web technologies has continuously aimed to optimize the interaction between servers and clients. Traditionally, using the XMLHttpRequest (XHR) method was the primary way to make network requests in JavaScript. However, XHR was cumbersome and lacked a promise-based structure, leading to complexities in managing asynchronous requests.
With the introduction of the Fetch API in 2015, life became easier for developers. It allows for a more intuitive syntax and promise-based handling of requests. However, as web applications began to handle more significant amounts of data—especially in the context of streaming media, real-time data feeds, and large file transfers—there was a growing need for improved mechanisms to process that data in a more efficient and performant way. This led to the implementation of the Streams API, which can be seamlessly integrated into Fetch operations.
Understanding Streams
What are Streams?
Streams are a set of data processing APIs in JavaScript that allow developers to read and write data in a continuous flow rather than loading it entirely into memory before processing. This allows applications to handle massive datasets more efficiently by processing chunks of data as they are received.
Types of Streams in JavaScript
- Readable Streams: Allow data to be read from a source.
- Writable Streams: Allow data to be written to a destination.
- Duplex Streams: Enable both reading and writing.
- Transform Streams: Modify the data as it is read or written.
In the context of Fetch, we primarily work with Readable Streams, which are especially advantageous when streaming responses from a network request.
The Fetch API Explained
How Fetch Works
The Fetch API provides a global fetch()
function that starts a network request to a specified resource. In its simplest form, it looks like this:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error fetching data:', error));
Handling Responses
When a Fetch request is made, it returns a promise that resolves to the Response object representing the response to the request. The Response object allows developers to access the status, headers, and body of the response, making it suitable for various use cases.
One of the most powerful features of the Response object is its ability to return a ReadableStream via the body
property. This is where we can leverage streams to process data efficiently.
Integrating Streams with Fetch
Basic Implementation
Here's how you can integrate streams with Fetch to process data incrementally. This is particularly useful for large datasets.
async function fetchAndProcessData(url) {
const response = await fetch(url);
const reader = response.body.getReader();
let result;
while (!(result = await reader.read()).done) {
const chunk = result.value; // Uint8Array
// Process the chunk, e.g. converting to text
const textChunk = new TextDecoder().decode(chunk);
console.log(textChunk);
}
}
fetchAndProcessData('https://api.example.com/large-data');
This basic example demonstrates how to read a response body in chunks. As each chunk is received, you can process it immediately without waiting for the entire dataset to download.
Handling Large Data Sets
Fetching large datasets can lead to excessive memory use if the entire dataset is buffered in memory. Instead, we can take advantage of the streaming capability.
async function fetchAndAggregateData(url) {
const response = await fetch(url);
const reader = response.body.getReader();
let result;
let aggregatedData = '';
while (!(result = await reader.read()).done) {
const chunk = new TextDecoder().decode(result.value);
aggregatedData += chunk;
}
// Parse aggregated data (assuming JSON)
const finalData = JSON.parse(aggregatedData);
console.log(finalData);
}
fetchAndAggregateData('https://api.example.com/large-json-data');
In this example, we read in chunks and aggregate them into a single string before parsing it as JSON. This method ensures that we only keep the necessary data in memory.
Advanced Scenarios and Use Cases
Transforming Data on-the-fly
To read and transform a stream simultaneously, you can utilize TransformStream
. This allows processing data as it is received, which can be crucial for applications that require immediate processing of incoming data.
const { ReadableStream, WritableStream, TransformStream } = require('stream/web');
const transformStream = new TransformStream({
transform(chunk, controller) {
const transformedChunk = new TextDecoder().decode(chunk).toUpperCase();
controller.enqueue(new TextEncoder().encode(transformedChunk));
}
});
async function fetchAndTransformData(url) {
const response = await fetch(url);
const reader = response.body.pipeThrough(transformStream).getReader();
let result;
while (!(result = await reader.read()).done) {
const transformedChunk = result.value; // Process as needed
console.log(new TextDecoder().decode(transformedChunk));
}
}
fetchAndTransformData('https://api.example.com/data');
Error Handling in Streams
Error scenarios are critical when dealing with streams, and handling them appropriately ensures that your application remains robust.
async function fetchWithErrorHandling(url) {
try {
const response = await fetch(url);
if (!response.ok) throw new Error(`HTTP error! status: ${response.status}`);
const reader = response.body.getReader();
let result;
while (!(result = await reader.read()).done) {
// Process your data
}
} catch (error) {
console.error('Stream processing error:', error);
}
}
This implementation catches errors either from the network request itself or during the data streaming process.
Comparative Analysis: Streams vs. Alternatives
XHR vs. Fetch with Streams
While XHR provided a way to handle network requests, it does not support modern promise-based patterns or streams. Here are the significant differences:
- Ease of Use: Fetch has a simpler API that supports promises and async/await, enhancing readability and maintainability.
- Stream Support: Fetch supports streams natively, allowing for efficient data processing, whereas XHR requires full data loading before processing.
WebSocket vs. Fetch Streams
WebSockets offer a full-duplex communication channel over a single, long-lived connection. The choice between using Fetch with streams versus WebSockets depends heavily on your use case:
- Fetch with Streams: Best for one-off requests or APIs where large datasets need to be processed.
- WebSockets: Ideal for scenarios that require continuous data feeds or when keeping a persistent connection is beneficial.
Performance Considerations
Streaming vs. Buffering
When using streams, you avoid buffering large responses entirely before processing. This leads to reduced memory usage and a more responsive user experience, allowing their application to continue functioning while waiting for data.
Memory Management
With large datasets, memory management becomes paramount. Streaming allows early disposal of processed chunks to free up memory. Always ensure that streams are correctly closed or canceled once you are done.
const controller = new AbortController();
const signal = controller.signal;
const response = await fetch(url, { signal });
reader.cancel();
controller.abort(); // to terminate the request
Potential Pitfalls
- Unhandled Errors: Not handling rejected promises can lead to unpredicted application states. Always ensure to have appropriate error handling when processing streams.
- Memory Leaks: Improperly handled streams or retaining references can lead to memory bloat. Use diagnostic tools and review your memory usage regularly.
- Network Issues: Consider implementing retry logic for potentially unstable network conditions.
Advanced Debugging Techniques
- Check Network Logs: Use browser developer tools to observe network requests and ensure data is being streamed as expected.
- Console Logging: Log the state of streams at different stages to monitor progress and identify potential issues.
- Performance Profiling: Use Chrome's Performance panel to profile your application's memory usage and CPU usage during streaming operations.
Conclusion
Incorporating streams into Fetch API enables developers to handle data more efficiently than ever before. This comprehensive guide has illustrated the power of streams for processing data incrementally, thereby optimizing performance, reducing memory overhead, and improving user experience. By understanding and leveraging these advanced techniques, seasoned developers can significantly enhance their web applications' capabilities.
References and Further Reading
- MDN Web Docs: Fetch API
- MDN Web Docs: Streams API
- Whatcom: The Streams API
- Node.js Documentation on Streams
This detailed exploration of using streams with Fetch should provide both theoretical understanding and practical implementation strategies to bolster any senior web developer’s approach to efficient data processing.