Understanding Strings, Object Memory, Static vs. Non-Static, and 'this' Keyword in Java Day 7 ...
Java Day 7 : Java is a powerful programming language that provides developers with various features to handle data and memory efficiently. In this blog, we will explore Strings, object memory locations, static vs. non-static variables, and the this keyword, specifically focusing on Java 7. 1. What is a String in Java? A String in Java is an immutable sequence of characters. Unlike primitive data types, strings are objects in Java, meaning they are instances of the String class. How to Create a String? Java provides multiple ways to create a string: Using String Literal (Stored in String Pool) String str1 = "Hello Java"; Using new Keyword (Stored in Heap Memory) String str2 = new String("Hello Java"); Both methods create a string, but the first method stores it in the String Pool, which helps in memory optimization. 2. Printing Object Memory Location In Java, objects are stored in heap memory, and we can print their memory reference using the System.identityHashCode() method. public class MemoryExample { public static void main(String[] args) { String str1 = new String("Java"); String str2 = "Java"; System.out.println(System.identityHashCode(str1)); // Heap memory System.out.println(System.identityHashCode(str2)); // String Pool memory } } Here, str1 is stored in heap memory, while str2 is stored in the String Pool. 3. Declaring a String Without Assigning a Value Java allows us to declare a string variable without assigning a value: String str; // Declaration without assignment However, if you try to print str before initializing, Java will throw a compilation error. So, always initialize it before use: str = "Hello"; 4. When to Use static and When to Use Non-Static? Static Variables and Methods: Belong to the class rather than an instance. Shared across all instances of the class. Can be accessed without creating an object. Example: class Example { static int counter = 0; static void showCounter() { System.out.println("Counter: " + counter); } } public class Main { public static void main(String[] args) { Example.counter = 10; Example.showCounter(); // Accessing without creating an object } } Non-Static Variables and Methods: Belong to individual objects. Require an object to access them. Example: class Example { int number = 5; // Non-static variable void display() { System.out.println("Number: " + number); } } public class Main { public static void main(String[] args) { Example obj = new Example(); obj.display(); // Requires object creation } } When to Use static? When a variable or method should be shared across all instances. When a method does not depend on instance variables. When to Use Non-Static? When different objects should have different values for a variable. When a method needs to access instance variables. 5. What is this Keyword in Java? The this keyword refers to the current instance of the class. It helps in differentiating between instance variables and method parameters when they have the same name. Example Without this (Ambiguity Issue) class Student { String name; void setName(String name) { name = name; // Here, both refer to the method parameter, so the instance variable remains unchanged } void display() { System.out.println("Student Name: " + name); } } In the above example, name = name; does not update the instance variable because both refer to the method parameter. Example With this (Corrected Version) class Student { String name; void setName(String name) { this.name = name; // Now, this.name refers to the instance variable } void display() { System.out.println("Student Name: " + this.name); } } 6. When to Use this and Why? Use Case Why Use this? To differentiate between instance and local variables Avoids variable name conflicts To call another constructor in the same class Reduces code duplication To pass the current object as an argument Helps when passing an object reference Using this to Call Another Constructor class Student { String name; Student() { this("Unknown"); // Calls the parameterized constructor } Student(String name) { this.name = name; } void display() { System.out.println("Student Name: " + name); } } Conclusion In this blog, we covered: ✔ Strings and how they are stored in Java. ✔ Printing memory locations of objects. ✔ Declaring a string without assigning a value. ✔ When to use static vs. non-static methods and variables. ✔ Understanding the this keyw
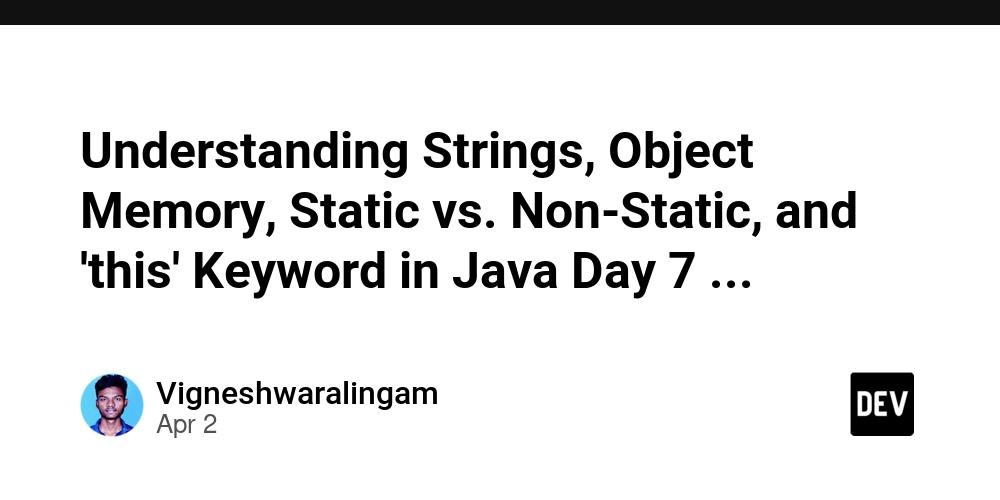
Java Day 7 :
Java is a powerful programming language that provides developers with various features to handle data and memory efficiently. In this blog, we will explore Strings, object memory locations, static vs. non-static variables, and the this
keyword, specifically focusing on Java 7.
1. What is a String in Java?
A String
in Java is an immutable sequence of characters. Unlike primitive data types, strings are objects in Java, meaning they are instances of the String
class.
How to Create a String?
Java provides multiple ways to create a string:
- Using String Literal (Stored in String Pool)
String str1 = "Hello Java";
-
Using
new
Keyword (Stored in Heap Memory)
String str2 = new String("Hello Java");
Both methods create a string, but the first method stores it in the String Pool, which helps in memory optimization.
2. Printing Object Memory Location
In Java, objects are stored in heap memory, and we can print their memory reference using the System.identityHashCode()
method.
public class MemoryExample {
public static void main(String[] args) {
String str1 = new String("Java");
String str2 = "Java";
System.out.println(System.identityHashCode(str1)); // Heap memory
System.out.println(System.identityHashCode(str2)); // String Pool memory
}
}
Here, str1
is stored in heap memory, while str2
is stored in the String Pool.
3. Declaring a String Without Assigning a Value
Java allows us to declare a string variable without assigning a value:
String str; // Declaration without assignment
However, if you try to print str
before initializing, Java will throw a compilation error. So, always initialize it before use:
str = "Hello";
4. When to Use static
and When to Use Non-Static?
Static Variables and Methods:
- Belong to the class rather than an instance.
- Shared across all instances of the class.
- Can be accessed without creating an object.
Example:
class Example {
static int counter = 0;
static void showCounter() {
System.out.println("Counter: " + counter);
}
}
public class Main {
public static void main(String[] args) {
Example.counter = 10;
Example.showCounter(); // Accessing without creating an object
}
}
Non-Static Variables and Methods:
- Belong to individual objects.
- Require an object to access them.
Example:
class Example {
int number = 5; // Non-static variable
void display() {
System.out.println("Number: " + number);
}
}
public class Main {
public static void main(String[] args) {
Example obj = new Example();
obj.display(); // Requires object creation
}
}
When to Use static
?
- When a variable or method should be shared across all instances.
- When a method does not depend on instance variables.
When to Use Non-Static?
- When different objects should have different values for a variable.
- When a method needs to access instance variables.
5. What is this
Keyword in Java?
The this
keyword refers to the current instance of the class. It helps in differentiating between instance variables and method parameters when they have the same name.
Example Without this
(Ambiguity Issue)
class Student {
String name;
void setName(String name) {
name = name; // Here, both refer to the method parameter, so the instance variable remains unchanged
}
void display() {
System.out.println("Student Name: " + name);
}
}
In the above example, name = name;
does not update the instance variable because both refer to the method parameter.
Example With this
(Corrected Version)
class Student {
String name;
void setName(String name) {
this.name = name; // Now, this.name refers to the instance variable
}
void display() {
System.out.println("Student Name: " + this.name);
}
}
6. When to Use this
and Why?
Use Case | Why Use this ? |
---|---|
To differentiate between instance and local variables | Avoids variable name conflicts |
To call another constructor in the same class | Reduces code duplication |
To pass the current object as an argument | Helps when passing an object reference |
Using this
to Call Another Constructor
class Student {
String name;
Student() {
this("Unknown"); // Calls the parameterized constructor
}
Student(String name) {
this.name = name;
}
void display() {
System.out.println("Student Name: " + name);
}
}
Conclusion
In this blog, we covered:
✔ Strings and how they are stored in Java.
✔ Printing memory locations of objects.
✔ Declaring a string without assigning a value.
✔ When to use static vs. non-static methods and variables.
✔ Understanding the this
keyword and its use cases.