Understanding Core React Hooks with Simple Analogies
What You'll Learn in This Guide In this article, we'll break down the most important React Hooks using relatable, real-world analogies. Here's what we’re covering: useState — Understand how to manage component state like tracking mood swings. useEffect — Learn how to handle side effects with precision, just like setting a smart reminder. useRef — Discover how to store values or references without triggering rerenders. useMemo & useCallback — See how to optimize performance by caching values and functions. Custom Hooks — Learn how to create reusable logic to simplify your components. useState — Like a Mood Tracker in Your Brain Ever found yourself switching moods faster than your playlist? One minute you're relaxed, the next you're stressed, then suddenly you're starving. That’s basically useState. const [mood, setMood] = useState("relaxed"); You're saving your current "mood" (aka state) and updating it whenever something changes. And just like how your best friend notices your vibe shift instantly, React does the same and rerenders accordingly. Reality Check: Still holding on to this.state like it’s your first coding project? Let it go. useState is simpler, cleaner, and far less awkward than binding methods like it's still 2015. useEffect — The "Hey, Something Changed" Hook Imagine setting a reminder that says, "If it rains, grab your umbrella." That’s how useEffect works — it only kicks in when specific things change. useEffect(() => { console.log("Don't forget the umbrella!"); }, [weather]); Think of it as that friend who only texts you when something interesting happens. Not always around, but super efficient. Reality Check: Don’t treat useEffect like a junk drawer. Just because you can throw everything into it, doesn’t mean you should. Keep it clean — future you will thank you. useRef — The Hidden Compartment Need a quiet little corner to stash something where React won’t poke around? That’s what useRef is for. const inputRef = useRef(null); It’s like your app’s glove box — perfect for DOM elements, timers, or anything you want to store without triggering rerenders. Real Talk: If you don’t need React to react (pun intended), skip useState and use useRef. It’s your silent helper. useMemo & useCallback — Brain Caching for Your Code Say your app’s doing some heavy calculations on every render. Let’s not waste CPU cycles. const result = useMemo(() => expensiveCalculation(), []); useMemo stores values. useCallback stores functions. Think of them like mental bookmarks for your brain — ready when you need them, not recalculated every time. But Heads Up: Don't go wrapping everything in useMemo like it's bubble wrap. Use it when it actually makes a difference — not just to look smart in code reviews. Custom Hooks — Your Code’s Personal Assistant Tired of copy-pasting the same logic across components? Build a custom hook and reuse it like a pro. function useOnlineStatus() { const [isOnline, setIsOnline] = useState(navigator.onLine); useEffect(() => { const handleStatus = () => setIsOnline(navigator.onLine); window.addEventListener("online", handleStatus); window.addEventListener("offline", handleStatus); return () => { window.removeEventListener("online", handleStatus); window.removeEventListener("offline", handleStatus); }; }, []); return isOnline; } Now you've got a plug-and-play solution. No more clutter. Just clean, modular logic. Still Nervous About Hooks? Don’t be. Hooks might seem weird at first — so did async/await, and now you love those. Yes, they take getting used to. But once they click, your components will be cleaner, more powerful, and future-ready. Final Thoughts (A Gentle Roast) If you're still clinging to class components in 2025, you're basically the tech version of using dial-up. Nostalgic, but let's move on. React Hooks made code leaner, smarter, and more modular. If you haven’t jumped in yet — now’s the time. Try. Fail. Learn. Repeat. And hey — got your own wild analogy for useEffect? Or a horror story involving useRef? Drop it in the comments.
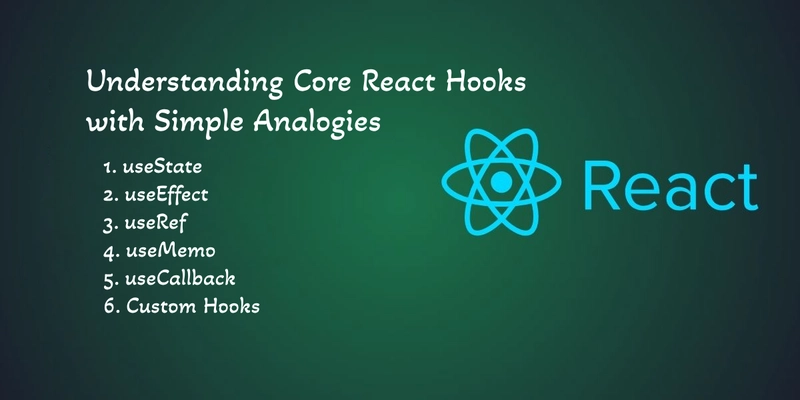
What You'll Learn in This Guide
In this article, we'll break down the most important React Hooks using relatable, real-world analogies. Here's what we’re covering:
useState
— Understand how to manage component state like tracking mood swings.
useEffect
— Learn how to handle side effects with precision, just like setting a smart reminder.
useRef
— Discover how to store values or references without triggering rerenders.
useMemo
& useCallback
— See how to optimize performance by caching values and functions.
Custom Hooks
— Learn how to create reusable logic to simplify your components.
useState
— Like a Mood Tracker in Your Brain
Ever found yourself switching moods faster than your playlist? One minute you're relaxed, the next you're stressed, then suddenly you're starving.
That’s basically useState
.
const [mood, setMood] = useState("relaxed");
You're saving your current "mood" (aka state) and updating it whenever something changes. And just like how your best friend notices your vibe shift instantly, React does the same and rerenders accordingly.
Reality Check:
Still holding on to this.state
like it’s your first coding project? Let it go. useState
is simpler, cleaner, and far less awkward than binding methods like it's still 2015.
useEffect
— The "Hey, Something Changed" Hook
Imagine setting a reminder that says, "If it rains, grab your umbrella." That’s how useEffect
works — it only kicks in when specific things change.
useEffect(() => {
console.log("Don't forget the umbrella!");
}, [weather]);
Think of it as that friend who only texts you when something interesting happens. Not always around, but super efficient.
Reality Check:
Don’t treat useEffect
like a junk drawer. Just because you can throw everything into it, doesn’t mean you should. Keep it clean — future you will thank you.
useRef
— The Hidden Compartment
Need a quiet little corner to stash something where React won’t poke around? That’s what useRef
is for.
const inputRef = useRef(null);
It’s like your app’s glove box — perfect for DOM elements, timers, or anything you want to store without triggering rerenders.
Real Talk:
If you don’t need React to react (pun intended), skip useState
and use useRef
. It’s your silent helper.
useMemo
& useCallback
— Brain Caching for Your Code
Say your app’s doing some heavy calculations on every render. Let’s not waste CPU cycles.
const result = useMemo(() => expensiveCalculation(), []);
useMemo
stores values. useCallback
stores functions. Think of them like mental bookmarks for your brain — ready when you need them, not recalculated every time.
But Heads Up:
Don't go wrapping everything in useMemo
like it's bubble wrap. Use it when it actually makes a difference — not just to look smart in code reviews.
Custom Hooks — Your Code’s Personal Assistant
Tired of copy-pasting the same logic across components? Build a custom hook and reuse it like a pro.
function useOnlineStatus() {
const [isOnline, setIsOnline] = useState(navigator.onLine);
useEffect(() => {
const handleStatus = () => setIsOnline(navigator.onLine);
window.addEventListener("online", handleStatus);
window.addEventListener("offline", handleStatus);
return () => {
window.removeEventListener("online", handleStatus);
window.removeEventListener("offline", handleStatus);
};
}, []);
return isOnline;
}
Now you've got a plug-and-play solution. No more clutter. Just clean, modular logic.
Still Nervous About Hooks?
Don’t be. Hooks might seem weird at first — so did async/await, and now you love those.
Yes, they take getting used to. But once they click, your components will be cleaner, more powerful, and future-ready.
Final Thoughts (A Gentle Roast)
If you're still clinging to class components in 2025, you're basically the tech version of using dial-up. Nostalgic, but let's move on.
React Hooks made code leaner, smarter, and more modular. If you haven’t jumped in yet — now’s the time. Try. Fail. Learn. Repeat.
And hey — got your own wild analogy for useEffect
? Or a horror story involving useRef
? Drop it in the comments.