Understanding Caching in Next.js
~ Index Introduction What is Caching in Next.js? Request Memoization Full Route Cache Data Cache Turbopack Cache Bonus Tips Conclusion ~ Introduction Caching is like the espresso shot of performance in web apps, small and capable of turning your app from "aehh" to "WoW" in milliseconds. If you're building with Next.js, especially the App Router, you already get some seriously clever caching for free. But what’s actually happening behind the scenes? In this post, we’ll break down the four major caching mechanisms used in Next.js: Request Memoization Full Route Cache Data Cache Turbopack Cache Let’s dive in!!! ~ What is Caching in Next.js? In short: Next.js caches things so it doesn’t have to re-fetch or re-render stuff unnecessarily. Depending on the type of data and request, it uses different strategies. The goal? Speed. Predictability. Scalability. Let’s break them down. ~ Request Memoization Ever call the same fetch() or DB query multiple times during a single render? Next.js is like: “Yo bro! I already did that.” Under the hood, it deduplicates fetches within the same request context. Example: app/page.tsx const users = await getUsers(); // fetches from DB const usersAgain = await getUsers(); // uses cached result, not another DB call Works automatically No config needed Reduces server load ~ Full Route Cache Static routes (like app/about/page.tsx) are fully cached. That means: The HTML is pre-rendered Served instantly from an edge cache Never hits your server (unless revalidated) If you use export const revalidate = 3600, it’ll refresh every hour. Or revalidate = 0 for dynamic routes. This is the bread and butter of Incremental Static Regeneration (ISR). // app/blog/[slug]/page.tsx export const revalidate = 86400; // cache page for 1 day Fast Edge-cached Smart refresh ~ Data Cache When you fetch() inside a Server Component, Next.js caches the response. This is especially useful when fetching from APIs or databases, it's smart enough to store and reuse those results across requests until you tell it otherwise. // app/page.tsx const res = await fetch('https://api.example.com/posts', { next: { revalidate: 60 } }); Options: revalidate: false = never cache revalidate: 0 = fetch fresh every time revalidate: 3600 = cache for 1 hour Works only in Server Components Cached globally ~ Turbopack Cache This one’s for the devs who hit ctrl + S every 3 seconds. Turbopack (Next.js's new bundler) caches your modules, transforms, and dependencies during development. Why? So hot reloads are instant. If Webpack was a bicycle, Turbopack is a Tesla. It remembers: Which files changed Which parts of the tree need rebuilding And skips everything else No config needed. Just enjoy the speed. You write code → it updates in
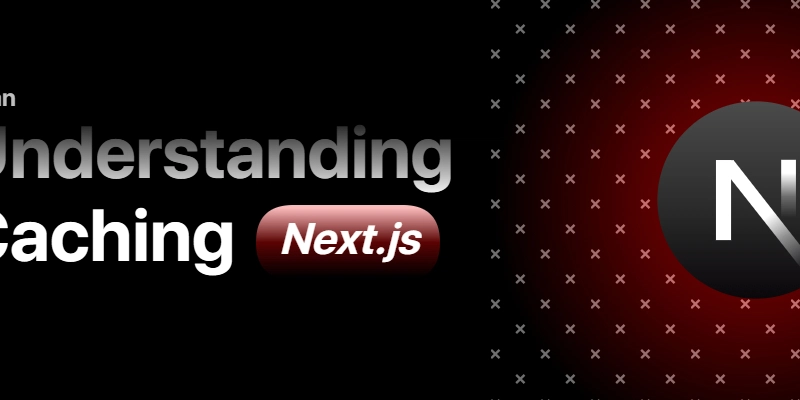
~ Index
- Introduction
- What is Caching in Next.js?
- Request Memoization
- Full Route Cache
- Data Cache
- Turbopack Cache
- Bonus Tips
- Conclusion
~ Introduction
Caching is like the espresso shot of performance in web apps, small and capable of turning your app from "aehh" to "WoW" in milliseconds. If you're building with Next.js, especially the App Router, you already get some seriously clever caching for free.
But what’s actually happening behind the scenes?
In this post, we’ll break down the four major caching mechanisms used in Next.js:
- Request Memoization
- Full Route Cache
- Data Cache
- Turbopack Cache
Let’s dive in!!!
~ What is Caching in Next.js?
In short: Next.js caches things so it doesn’t have to re-fetch or re-render stuff unnecessarily. Depending on the type of data and request, it uses different strategies. The goal?
Speed. Predictability. Scalability.
Let’s break them down.
~ Request Memoization
Ever call the same fetch()
or DB query multiple times during a single render?
Next.js is like: “Yo bro! I already did that.”
Under the hood, it deduplicates fetches within the same request context.
Example:
app/page.tsx
const users = await getUsers(); // fetches from DB
const usersAgain = await getUsers(); // uses cached result, not another DB call
- Works automatically
- No config needed
- Reduces server load
~ Full Route Cache
Static routes (like app/about/page.tsx
) are fully cached.
That means:
- The HTML is pre-rendered
- Served instantly from an edge cache
- Never hits your server (unless revalidated)
If you use export const revalidate = 3600
, it’ll refresh every hour.
Or revalidate = 0
for dynamic routes.
This is the bread and butter of Incremental Static Regeneration (ISR).
// app/blog/[slug]/page.tsx
export const revalidate = 86400; // cache page for 1 day
- Fast
- Edge-cached
- Smart refresh
~ Data Cache
When you fetch()
inside a Server Component, Next.js caches the response.
This is especially useful when fetching from APIs or databases, it's smart enough to store and reuse those results across requests until you tell it otherwise.
// app/page.tsx
const res = await fetch('https://api.example.com/posts', {
next: { revalidate: 60 }
});
Options:
-
revalidate: false
= never cache -
revalidate: 0
= fetch fresh every time revalidate: 3600
= cache for 1 hourWorks only in Server Components
Cached globally
~ Turbopack Cache
This one’s for the devs who hit ctrl + S every 3 seconds.
Turbopack (Next.js's new bundler) caches your modules, transforms, and dependencies during development.
Why? So hot reloads are instant.
If Webpack was a bicycle, Turbopack is a Tesla.
It remembers:
- Which files changed
- Which parts of the tree need rebuilding
- And skips everything else
No config needed. Just enjoy the speed.
- You write code → it updates in <200ms
- Love at first refresh
~ Bonus Tips
Let’s level up your caching game:
1. Use revalidateTag()
and unstable_cache()
Want to manually bust cache from a route or action?
import { revalidateTag } from 'next/cache';
revalidateTag('posts');
Tag your fetches:
await fetch('/api/posts', { next: { tags: ['posts'] } });
Boom. Granular control.
2. Edge Functions = Edge Cache
Want truly global performance?
Deploy with Vercel and use edge functions — they cache and serve from closest regions to the user.
Latency = who?
3. Opt out of caching (when needed)
Not all data should be cached.
await fetch(url, { cache: 'no-store' });
Useful for dashboards, user-specific content, or live updates.
~ Conclusion
Here's what we covered:
- Request Memoization
- Full Route Cache
- Data Cache
- Turbopack Cache
Caching is one of those things where knowing the defaults = superpowers. Next.js does a lot for you, but now, you know how to control it.
Need help with revalidation strategies? Confused about dynamic content?
You know what to do.
Questions? Ideas? Cache bugs?
Drop them below