Understanding Apex Data Types: The Building Blocks of Salesforce Development
Have you ever wondered how developers create powerful applications within Salesforce without starting from scratch each time? It's like having access to a robust set of pre-made Lego blocks that snap together perfectly, allowing you to build incredible structures quickly. These building blocks in the Salesforce ecosystem are called Apex data types—fundamental components that form the foundation of any Salesforce development project. Whether you're a business leader trying to understand your development team better or a developer looking to refresh your knowledge, understanding these essentials can transform how you approach Salesforce solutions. What Are Apex Data Types? Apex is Salesforce's proprietary programming language, designed specifically for the giant CRM platform. Like in any programming language, data types in Apex tell the system what kind of data a variable can hold and how it should be stored, manipulated, and displayed. Why does this matter? Because efficient data handling is the backbone of every successful Salesforce implementation. When your data is properly structured, your applications run faster, are easier to maintain, and deliver better user experiences. For businesses, this translates directly to improved productivity, better customer insights, and ultimately, increased ROI on your Salesforce investment. Apex Data Types: The Simple Version Apex data types can be categorized into: Primitive data types: These are the basic building blocks—like individual Lego pieces. They hold single values such as numbers, text, or true/false values. Collection data types: These are like organized toy boxes that hold multiple primitive pieces in different ways—some maintain order, some associate values with labels, and others ensure no duplicates. sObject data types: These are specialized containers designed specifically to hold Salesforce records, like contacts, opportunities, or custom objects your company has created. Enums: Think of these as preset dial positions—predefined values that a variable can be set to, like days of the week or status categories. Custom Apex Classes: These are your custom-built Lego kits, designed for specific purposes within your organization. Apex Data Types: Diving Deeper 1. Primitive data types Integer: Whole numbers without decimal points (e.g., 42, -7, 1000). Integer employeeCount = 157; Long: Similar to integer but provides a wider range of values than integers. Long l = 2147483648L; Decimal: Numbers that can have decimal points for precision (e.g., 3.14, 2.5). Decimal productPrice = 29.99; Double: Similar to Decimal but processed differently by the system, offering a trade-off between precision and performance. Double pi = 3.14159; String: Any set of characters surrounded by single quotes. String customerName = 'John Smith'; Boolean: True/false values, perfect for yes/no situations. Boolean isActive = true; Date: Represents a specific date without time information. Date dueDate = Date.today(); DateTime: Represents a specific date and time (such as a timestamp). DateTime meetingTime = DateTime.now(); ID: Salesforce's unique identifier format for records, always 18 characters. Id contactId = '00300000003T2PGAA0'; Blob: Short for Binary Large Object, is a data type used to store binary data such as files(PDF, images, docs, etc), encrypted data, content not plain text, etc. Blobs are not human-readable. Blob fileData = Blob.valueOf('File content here'); Blobs are super useful when working with files and APIs. 2. Collection data types: Organizing Multiple Values Collections are where things get interesting for developers because they allow for efficient data manipulation. Lists: Ordered collections that can contain duplicates. List elements can be of any data type; primitive types, collections, sObjects, user-defined types, and built-in Apex types. List teamMembers = new List{'Alex', 'Taylor', 'Jordan', 'Morgan'}; Sets: Unordered collections that cannot contain duplicates. Set elements can be of any data type—primitive types, collections, sObjects, user-defined types, and built-in Apex types. Set uniqueCategories = new Set{'Electronics', 'Apparel', 'Home Goods'}; Maps: Collections of key-value pairs where each key is unique. Map contactMap = new Map([SELECT Id, Name FROM Contact LIMIT 10]); 3. sObjects: Salesforce Records at Your Fingertips In Salesforce, data is stored in objects like Accounts, Contacts, and Opportunities. Apex provides sObject types to work with these records, making them essential for automation and customization. Account acc = new Account(Name = 'Tech Corp'); insert acc; 4. Enums: Defining Fixed Choices Enums allow you to create a set of predefined constants. public enum Status { NEW, IN_PROGRESS, COMPLETED } Status taskStatus=Status.NEW;
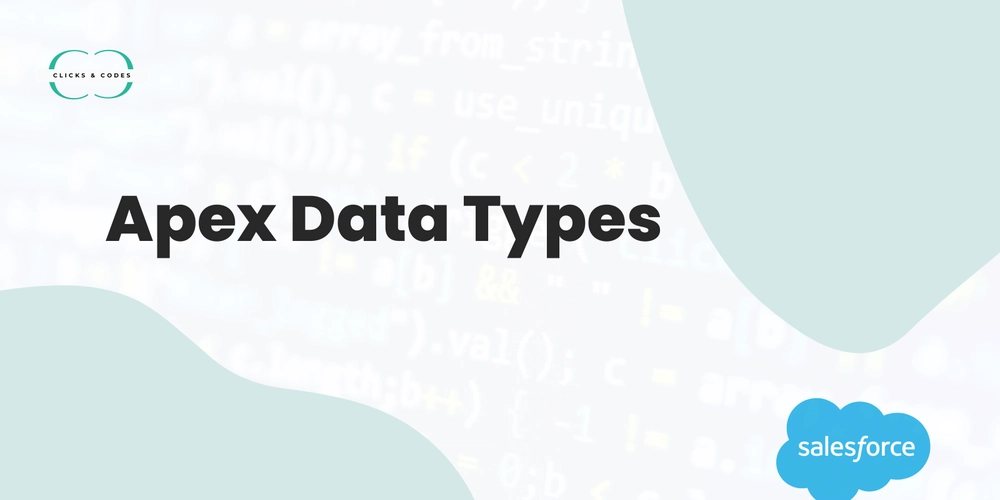
Have you ever wondered how developers create powerful applications within Salesforce without starting from scratch each time? It's like having access to a robust set of pre-made Lego blocks that snap together perfectly, allowing you to build incredible structures quickly. These building blocks in the Salesforce ecosystem are called Apex data types—fundamental components that form the foundation of any Salesforce development project. Whether you're a business leader trying to understand your development team better or a developer looking to refresh your knowledge, understanding these essentials can transform how you approach Salesforce solutions.
What Are Apex Data Types?
Apex is Salesforce's proprietary programming language, designed specifically for the giant CRM platform. Like in any programming language, data types in Apex tell the system what kind of data a variable can hold and how it should be stored, manipulated, and displayed.
Why does this matter?
Because efficient data handling is the backbone of every successful Salesforce implementation. When your data is properly structured, your applications run faster, are easier to maintain, and deliver better user experiences. For businesses, this translates directly to improved productivity, better customer insights, and ultimately, increased ROI on your Salesforce investment.
Apex Data Types: The Simple Version
Apex data types can be categorized into:
Primitive data types: These are the basic building blocks—like individual Lego pieces. They hold single values such as numbers, text, or true/false values.
Collection data types: These are like organized toy boxes that hold multiple primitive pieces in different ways—some maintain order, some associate values with labels, and others ensure no duplicates.
sObject data types: These are specialized containers designed specifically to hold Salesforce records, like contacts, opportunities, or custom objects your company has created.
Enums: Think of these as preset dial positions—predefined values that a variable can be set to, like days of the week or status categories.
Custom Apex Classes: These are your custom-built Lego kits, designed for specific purposes within your organization.
Apex Data Types: Diving Deeper
1. Primitive data types
- Integer: Whole numbers without decimal points (e.g., 42, -7, 1000).
Integer employeeCount = 157;
- Long: Similar to integer but provides a wider range of values than integers.
Long l = 2147483648L;
- Decimal: Numbers that can have decimal points for precision (e.g., 3.14, 2.5).
Decimal productPrice = 29.99;
- Double: Similar to Decimal but processed differently by the system, offering a trade-off between precision and performance.
Double pi = 3.14159;
- String: Any set of characters surrounded by single quotes.
String customerName = 'John Smith';
- Boolean: True/false values, perfect for yes/no situations.
Boolean isActive = true;
- Date: Represents a specific date without time information.
Date dueDate = Date.today();
- DateTime: Represents a specific date and time (such as a timestamp).
DateTime meetingTime = DateTime.now();
- ID: Salesforce's unique identifier format for records, always 18 characters.
Id contactId = '00300000003T2PGAA0';
- Blob: Short for Binary Large Object, is a data type used to store binary data such as files(PDF, images, docs, etc), encrypted data, content not plain text, etc. Blobs are not human-readable.
Blob fileData = Blob.valueOf('File content here');
Blobs are super useful when working with files and APIs.
2. Collection data types: Organizing Multiple Values
Collections are where things get interesting for developers because they allow for efficient data manipulation.
- Lists: Ordered collections that can contain duplicates. List elements can be of any data type; primitive types, collections, sObjects, user-defined types, and built-in Apex types.
List teamMembers = new List{'Alex', 'Taylor', 'Jordan', 'Morgan'};
- Sets: Unordered collections that cannot contain duplicates. Set elements can be of any data type—primitive types, collections, sObjects, user-defined types, and built-in Apex types.
Set uniqueCategories = new Set{'Electronics', 'Apparel', 'Home Goods'};
- Maps: Collections of key-value pairs where each key is unique.
Map contactMap = new Map([SELECT Id, Name FROM Contact LIMIT 10]);
3. sObjects: Salesforce Records at Your Fingertips
In Salesforce, data is stored in objects like Accounts, Contacts, and Opportunities. Apex provides sObject types to work with these records, making them essential for automation and customization.
Account acc = new Account(Name = 'Tech Corp');
insert acc;
4. Enums: Defining Fixed Choices
Enums allow you to create a set of predefined constants.
public enum Status { NEW, IN_PROGRESS, COMPLETED }
Status taskStatus=Status.NEW;
5. Objects & Classes: Custom Data Structures
When built-in types aren’t enough, Apex allows you to create your own objects and classes.
public class Car {
public String model;
public Integer year;
public void Car(String model, Integer year) {
this.model = model;
this.year = year;
}
}
Car myCar = new Car('Tesla', 2023);
Real-World Applications: Putting Data Types to Work
Imagine a retail company using Salesforce to manage its operations. Here's how different data types power various functions:
Customer Records: sObject data types store comprehensive customer profiles, including purchase history and preferences.
Inventory Management: Primitive types like Integer track quantities, while Decimal types handle pricing.
Sales Analytics: Collection types organize sales data by region (Maps), maintain unique product categories (Sets), and store ordered transaction histories (Lists).
Automated Marketing: Custom Apex classes combine multiple data types to create personalized marketing journeys based on customer behavior.
Best Practices and Common Pitfalls
For developers and architects, here are some useful insights and best practices:
Type Casting: Be careful when converting between data types. Apex is strongly typed, so conversions must be explicit.
Bulkification: When working with collections, design your code to handle bulk operations efficiently to respect Salesforce's governor limits.
Null Handling: Always check for null values when working with variables that might not contain data.
Using the Right Collection: Choose your collection type based on your specific needs:
Need order and possibly duplicates? Use a List.
Need uniqueness only? Use a Set.
Need to associate values with keys? Use a Map.
Bringing It All Together
Even at a high level, understanding Apex data types empowers business leaders to have more productive conversations with their development teams.
For developers, mastering these foundational elements is the key to writing efficient, scalable, and maintainable code. Whether you're optimising existing processes, troubleshooting performance issues, or designing entirely new solutions, the right application of data types can make all the difference. They may seem like abstract technical concepts, but their impact on your business applications is real and measurable.
What Apex data type challenges are you facing in your organization? Have you found creative ways to leverage these building blocks for business advantage? The conversation around Apex continues to evolve, and your experiences could help shape best practices for the entire Salesforce community.