Types of Databases: Relational, NoSQL, and Beyond
Introduction: Different Types of Databases Not all databases are created equal. The type of database you choose depends on your application’s needs, such as the structure of your data, the volume, and the speed of operations. In this post, we’ll explore the most common types of databases and their unique features. 1. Relational Databases Relational databases organize data into tables with rows and columns, where relationships between data are defined explicitly. Examples: MySQL, PostgreSQL, SQLite, Oracle Database. Best For: Structured data like financial records, customer databases, or inventory systems. Key Features: Data stored in tables. Supports SQL (Structured Query Language). Strong data integrity through primary and foreign keys. Code Example: Simple Relational Query -- Retrieve all orders for a specific customer SELECT * FROM orders WHERE customer_id = 101; 2. NoSQL Databases NoSQL databases are designed for flexibility and scalability. They are ideal for unstructured or semi-structured data and are not restricted by the tabular schema of relational databases. Types of NoSQL Databases: Document: Stores data as JSON or BSON (e.g., MongoDB). Key-Value: Data is stored as key-value pairs (e.g., Redis). Column-Family: Similar to tables but optimized for large-scale analytics (e.g., Cassandra). Graph: Designed for relationships between data (e.g., Neo4j). Example Query (MongoDB): // Find all users whose age is greater than 25 db.users.find({ age: { $gt: 25 } }); 3. Cloud Databases Cloud databases are hosted on cloud platforms and offer scalability, reliability, and global access. Examples: AWS RDS, Google Cloud Spanner, Azure SQL. Best For: Applications requiring dynamic scaling and minimal infrastructure management. 4. In-Memory Databases Data is stored directly in memory (RAM), making them extremely fast. Examples: Redis, Memcached. Best For: Caching, session storage, and real-time analytics. Challenges Scenario: You’re building a social media app. Which database would you choose to store millions of user profiles and why? Question: Can you think of scenarios where combining relational and NoSQL databases might be beneficial?
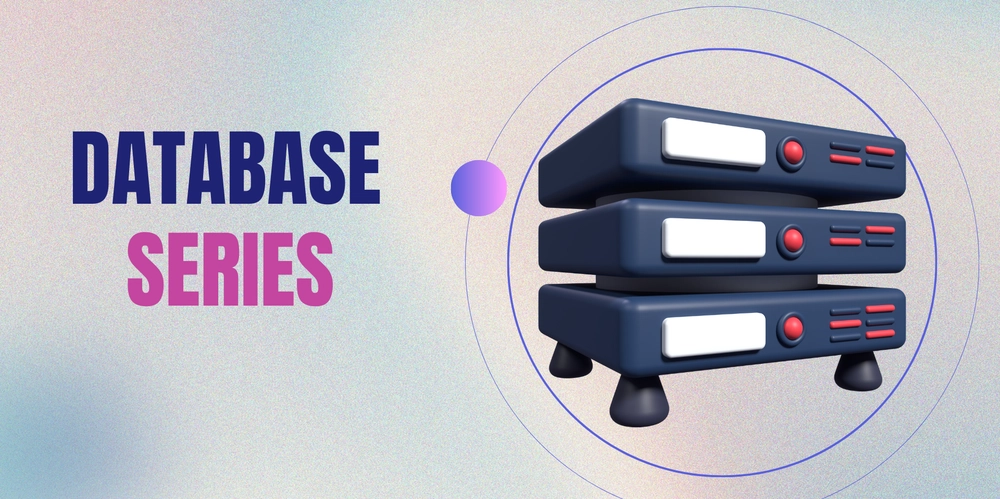
Introduction: Different Types of Databases
Not all databases are created equal. The type of database you choose depends on your application’s needs, such as the structure of your data, the volume, and the speed of operations. In this post, we’ll explore the most common types of databases and their unique features.
1. Relational Databases
Relational databases organize data into tables with rows and columns, where relationships between data are defined explicitly.
- Examples: MySQL, PostgreSQL, SQLite, Oracle Database.
- Best For: Structured data like financial records, customer databases, or inventory systems.
- Key Features:
- Data stored in tables.
- Supports SQL (Structured Query Language).
- Strong data integrity through primary and foreign keys.
Code Example: Simple Relational Query
-- Retrieve all orders for a specific customer
SELECT *
FROM orders
WHERE customer_id = 101;
2. NoSQL Databases
NoSQL databases are designed for flexibility and scalability. They are ideal for unstructured or semi-structured data and are not restricted by the tabular schema of relational databases.
- Types of NoSQL Databases:
- Document: Stores data as JSON or BSON (e.g., MongoDB).
- Key-Value: Data is stored as key-value pairs (e.g., Redis).
- Column-Family: Similar to tables but optimized for large-scale analytics (e.g., Cassandra).
- Graph: Designed for relationships between data (e.g., Neo4j).
Example Query (MongoDB):
// Find all users whose age is greater than 25
db.users.find({ age: { $gt: 25 } });
3. Cloud Databases
Cloud databases are hosted on cloud platforms and offer scalability, reliability, and global access.
- Examples: AWS RDS, Google Cloud Spanner, Azure SQL.
- Best For: Applications requiring dynamic scaling and minimal infrastructure management.
4. In-Memory Databases
Data is stored directly in memory (RAM), making them extremely fast.
- Examples: Redis, Memcached.
- Best For: Caching, session storage, and real-time analytics.
Challenges
Scenario: You’re building a social media app. Which database would you choose to store millions of user profiles and why?
Question: Can you think of scenarios where combining relational and NoSQL databases might be beneficial?