TS1403: Type library referenced via '{0}' from file '{1}' with packageId '{2}'
TS1403: Type library referenced via '{0}' from file '{1}' with packageId '{2}' When diving into the world of web development, you may have heard of TypeScript, a strongly typed programming language that builds upon JavaScript (commonly referred to as its superset). If you're already working with JavaScript, TypeScript enhances your experience with features like static typing, interfaces, enums, and modern tools to avoid runtime issues long before they occur. TypeScript helps coders to catch potential bugs and improve code clarity by bringing types (specific definitions for variables, objects, and functions) into the equation. In this article, we’ll explore the error TS1403: Type library referenced via '{0}' from file '{1}' with packageId '{2}'. But before we dive into the details of this specific issue, let’s take a moment to understand what types mean in TypeScript and why they're essential. If you're keen to master TypeScript or learn to code like a pro, consider subscribing to my blog or exploring AI-powered tools like GPTeach to guide your journey. What is TypeScript, and What are Types? TypeScript is a programming language developed by Microsoft and seen as a superset of JavaScript. This means that every line of valid JavaScript is also valid TypeScript, but TypeScript introduces additional features not present in JavaScript. One of its key features is its support for static typing. Types are simply a way to define what kind of value a variable can hold. For example, in JavaScript, you can assign anything to a variable (let x = 5; x = "hello";). TypeScript prevents such ambiguity by enforcing types like string, number, and boolean. Here's an example of a type in TypeScript: let age: number = 30; // `age` must be a number age = "thirty"; // ERROR: Type 'string' is not assignable to type 'number' The primary advantage of defining types is catching errors at compile time (while compiling the code), way before it executes. About the Error: TS1403: Type library referenced via '{0}' from file '{1}' with packageId '{2}' The error TS1403: Type library referenced via '{0}' from file '{1}' with packageId '{2}' occurs when there’s an issue with a type library that TypeScript references. Type libraries are often used for type definitions in TypeScript projects to enable compatibility between various tools, packages, or dependencies. A classic example involves .d.ts files (declaration files). These files help TypeScript understand external JavaScript libraries' types. Common Causes of TS1403 Here are some potential reasons for encountering TS1403: A referenced type library file is missing or corrupted. The packageId referenced in the error message does not exist. There's a version mismatch between the referenced library and your project. The TypeScript configuration (tsconfig.json) does not properly resolve the type definitions. Code Example: When TS1403 Happens Here’s an example where the TS1403: Type library referenced via '{0}' from file '{1}' with packageId '{2}' error might occur: // tsconfig.json { "compilerOptions": { "types": ["nonExistentLibrary"] } } // TypeScript file let message: string = "Hello, TypeScript!"; message.toUpperCase(); In this case: The tsconfig.json specifies a type dependency nonExistentLibrary that doesn’t exist in node_modules. Running the TypeScript compiler (tsc) would throw TS1403 because it can't find the referenced type library. How to Fix TS1403 Prerequisites to Fix This Error: Ensure dependencies are correctly installed in your project. Understand and verify your tsconfig.json settings. Resolve any version mismatches between the type library and your project. Here’s how you can address the issue: Check and Install Missing Libraries If the library doesn’t exist, install the necessary types using npm: npm install --save-dev @types/nonExistentLibrary Modify tsconfig.json Ensure your tsconfig.json doesn’t reference libraries you’re not using. For the above code: { "compilerOptions": { "types": [] } } Remove or Verify Incorrect packageId If the error cites a package ID (packageId {2}), ensure the ID is valid or isn’t outdated. Update your dependencies with: npm install FAQ: Solving TS1403 and Understanding Types in TypeScript 1. What is a .d.ts file in TypeScript? .d.ts files are type declaration files that provide TypeScript with type information for external JavaScript libraries. These files bridge the gap between JavaScript and TypeScript. 2. What is the purpose of tsconfig.json? The tsconfig.json provides TypeScript's compiler options and settings for your project. For example, it specifies which libraries to include, the target ECMAScript version, and paths for resolving modules. 3. Can I disable type checking for a specific library?
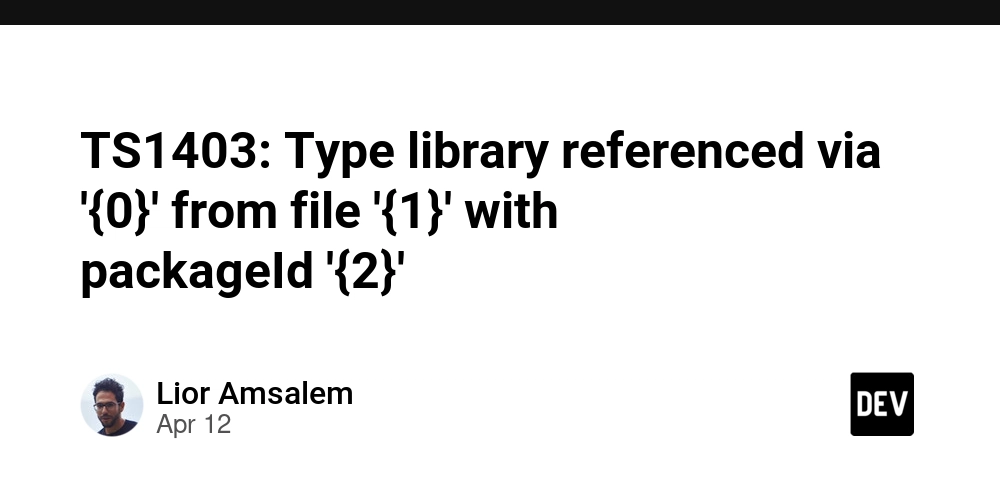
TS1403: Type library referenced via '{0}' from file '{1}' with packageId '{2}'
When diving into the world of web development, you may have heard of TypeScript, a strongly typed programming language that builds upon JavaScript (commonly referred to as its superset). If you're already working with JavaScript, TypeScript enhances your experience with features like static typing, interfaces, enums, and modern tools to avoid runtime issues long before they occur. TypeScript helps coders to catch potential bugs and improve code clarity by bringing types (specific definitions for variables, objects, and functions) into the equation.
In this article, we’ll explore the error TS1403: Type library referenced via '{0}' from file '{1}' with packageId '{2}'
. But before we dive into the details of this specific issue, let’s take a moment to understand what types mean in TypeScript and why they're essential. If you're keen to master TypeScript or learn to code like a pro, consider subscribing to my blog or exploring AI-powered tools like GPTeach to guide your journey.
What is TypeScript, and What are Types?
TypeScript is a programming language developed by Microsoft and seen as a superset of JavaScript. This means that every line of valid JavaScript is also valid TypeScript, but TypeScript introduces additional features not present in JavaScript. One of its key features is its support for static typing.
Types are simply a way to define what kind of value a variable can hold. For example, in JavaScript, you can assign anything to a variable (let x = 5; x = "hello";
). TypeScript prevents such ambiguity by enforcing types like string
, number
, and boolean
.
Here's an example of a type in TypeScript:
let age: number = 30; // `age` must be a number
age = "thirty"; // ERROR: Type 'string' is not assignable to type 'number'
The primary advantage of defining types is catching errors at compile time (while compiling the code), way before it executes.
About the Error: TS1403: Type library referenced via '{0}' from file '{1}' with packageId '{2}'
The error TS1403: Type library referenced via '{0}' from file '{1}' with packageId '{2}'
occurs when there’s an issue with a type library that TypeScript references. Type libraries are often used for type definitions in TypeScript projects to enable compatibility between various tools, packages, or dependencies. A classic example involves .d.ts
files (declaration files). These files help TypeScript understand external JavaScript libraries' types.
Common Causes of TS1403
Here are some potential reasons for encountering TS1403
:
- A referenced type library file is missing or corrupted.
- The
packageId
referenced in the error message does not exist. - There's a version mismatch between the referenced library and your project.
- The TypeScript configuration (
tsconfig.json
) does not properly resolve the type definitions.
Code Example: When TS1403 Happens
Here’s an example where the TS1403: Type library referenced via '{0}' from file '{1}' with packageId '{2}'
error might occur:
// tsconfig.json
{
"compilerOptions": {
"types": ["nonExistentLibrary"]
}
}
// TypeScript file
let message: string = "Hello, TypeScript!";
message.toUpperCase();
In this case:
- The
tsconfig.json
specifies a type dependencynonExistentLibrary
that doesn’t exist innode_modules
. - Running the TypeScript compiler (
tsc
) would throwTS1403
because it can't find the referenced type library.
How to Fix TS1403
Prerequisites to Fix This Error:
- Ensure dependencies are correctly installed in your project.
- Understand and verify your
tsconfig.json
settings. - Resolve any version mismatches between the type library and your project.
Here’s how you can address the issue:
- Check and Install Missing Libraries
If the library doesn’t exist, install the necessary types using npm:
npm install --save-dev @types/nonExistentLibrary
- Modify tsconfig.json
Ensure your tsconfig.json
doesn’t reference libraries you’re not using. For the above code:
{
"compilerOptions": {
"types": []
}
}
- Remove or Verify Incorrect packageId
If the error cites a package ID (packageId {2}
), ensure the ID is valid or isn’t outdated. Update your dependencies with:
npm install
FAQ: Solving TS1403 and Understanding Types in TypeScript
1. What is a .d.ts
file in TypeScript?
.d.ts
files are type declaration files that provide TypeScript with type information for external JavaScript libraries. These files bridge the gap between JavaScript and TypeScript.
2. What is the purpose of tsconfig.json
?
The tsconfig.json
provides TypeScript's compiler options and settings for your project. For example, it specifies which libraries to include, the target ECMAScript version, and paths for resolving modules.
3. Can I disable type checking for a specific library?
Yes! You can exclude a library by using the skipLibCheck
option in tsconfig.json
:
{
"compilerOptions": {
"skipLibCheck": true
}
}
However, use this cautiously, as it disables type checks for all libraries and may hide critical errors.
Important to Know!
- Always keep your dependencies updated. Many TS1403 issues arise from outdated or incompatible type libraries.
-
Understand your
tsconfig.json
file. Misconfiguration often leads to library errors like TS1403. - Intermediate Errors Can Cascade! Resolving one type error might reveal another downstream issue. Be patient and tackle them step by step.
Conclusion
Handling the TS1403: Type library referenced via '{0}' from file '{1}' with packageId '{2}'
error may seem complex at first, but understanding how TypeScript manages type definitions and libraries simplifies the process. With proper attention to your tsconfig.json
and an organized project setup, these issues can be resolved efficiently.
TypeScript is a powerful tool for modern web development. Whether you're learning about types, interfaces, or enums, taking the time to understand these core concepts will elevate your coding ability. And for those who want to dive further into TypeScript and solve more such errors, consider using GPTeach to learn interactively and grow your skills—one line of code at a time!
Happy coding!