TS1394: Imported via {0} from file '{1}' with packageId '{2}'
TS1394: Imported via {0} from file '{1}' with packageId '{2}' TypeScript is a strongly-typed superset of JavaScript that adds optional static typing to the language. It enhances the developer experience by catching potential bugs at compile time and providing features like type definitions, interfaces, generics, and more. If you're new to TypeScript, it's worth noting that types (descriptions of data) allow you to define the structure of your code explicitly, making it more robust and maintainable. For example: let age: number = 25; // The variable `age` can only hold a number. let name: string = "Alice"; // The variable `name` is strictly a string. If you're interested in exploring TypeScript further, or learning how to code with AI-powered tools like GPT-based systems, make sure to visit GPTeach.us and subscribe to our blog to stay updated and sharpen your coding skills. Now, let's dive deeper into one key concept of TypeScript: Interfaces. What Are Interfaces? In TypeScript, interfaces define the structure of an object. They act as a contract for your data, ensuring that an object adheres to a specific shape. This allows you to enforce consistency across your codebase. Here's a simple example: interface Person { name: string; age: number; } const user: Person = { name: "John Doe", age: 30, }; In this example, the Person interface ensures that any object of type Person has both the name and age properties, and they must be of type string and number, respectively. Any violation of this contract will result in a compile-time error. Understanding the Error: TS1394: Imported via {0} from file '{1}' with packageId '{2}' This infamous error in TypeScript typically arises when there is some sort of type definition inconsistency or problem during module import. Let’s dissect this error in simpler, human-friendly terms and tackle how we can resolve it effectively. The error message might look intimidating at first, but it's a helpful indicator that something isn't allowing TypeScript's type checker to properly resolve the types being imported in a module. Example Scenario Imagine you’re working with a shared library that includes pre-defined types, but when you try to import these types, you see the error TS1394: Imported via {0} from file '{1}' with packageId '{2}'. Here is a basic example that could cause the error: // utils.d.ts export type User = { id: string; name: string; }; // app.ts import { User } from './utils'; // Error: TS1394 Possible reasons for the error: Type Mismatch: The definition of User in the file being imported may be inconsistent (e.g., misspelling or incorrect property types). Missing Definitions: TypeScript is unable to locate or resolve the type correctly due to incorrect pathing. Package Resolution Issue: If you're importing types from a library, it might be outdated or incorrectly set up. Fixing TS1394: Imported via {0} from file '{1}' with packageId '{2}' Here are step-by-step solutions to diagnose and fix this issue: 1. Ensure Correct Import Paths Check if the file path and type names in the import statement are accurate. // Fix if the file path is incorrect import { User } from './utils'; 2. Validate Type Definitions Verify that the type definitions are valid and align with expectations. // Correct definition export type User = { id: string; // Ensure `id` is a string, not anything else like number. name: string; }; 3. Install/Update Dependencies If the type comes from an external package, ensure the package is correctly installed and up-to-date. npm install package-name --save Also, ensure your tsconfig.json correctly includes type declarations: { "compilerOptions": { "typeRoots": ["node_modules/@types"] } } Important to Know! TypeScript resolves types based on the tsconfig.json file. Make sure that your configuration specifies proper module resolution. For external types from a package, always use the latest versions unless specified otherwise in your project. FAQs About TS1394 1. What does "packageId" mean in TS1394? The packageId refers to the package where the type definitions come from. If it's a custom module, make sure that it is exported properly. 2. Can TypeScript check types across multiple files? Yes, TypeScript can infer and enforce types across any number of files, as long as they're part of your project and linked through import/export statements. 3. How do I debug TS1394 errors? Start by checking your import paths, tsconfig.json, and the type definition files (often .d.ts files). Important to Know! TypeScript relies heavily on proper export and import mechanics. All custom types must be explicitly exported from their respective files. When developing libraries, use .d.ts files to define clear and shareable type def
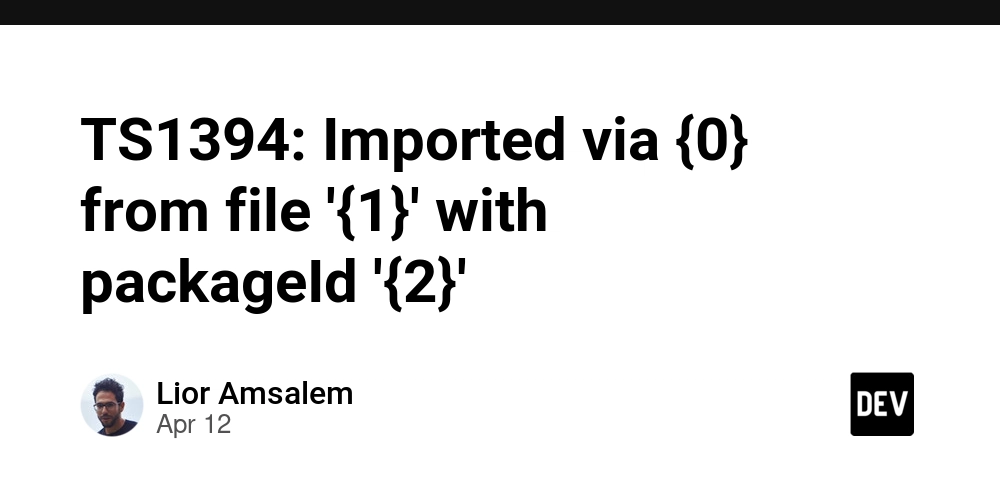
TS1394: Imported via {0} from file '{1}' with packageId '{2}'
TypeScript is a strongly-typed superset of JavaScript that adds optional static typing to the language. It enhances the developer experience by catching potential bugs at compile time and providing features like type definitions, interfaces, generics, and more. If you're new to TypeScript, it's worth noting that types (descriptions of data) allow you to define the structure of your code explicitly, making it more robust and maintainable. For example:
let age: number = 25; // The variable `age` can only hold a number.
let name: string = "Alice"; // The variable `name` is strictly a string.
If you're interested in exploring TypeScript further, or learning how to code with AI-powered tools like GPT-based systems, make sure to visit GPTeach.us and subscribe to our blog to stay updated and sharpen your coding skills.
Now, let's dive deeper into one key concept of TypeScript: Interfaces.
What Are Interfaces?
In TypeScript, interfaces
define the structure of an object. They act as a contract for your data, ensuring that an object adheres to a specific shape. This allows you to enforce consistency across your codebase.
Here's a simple example:
interface Person {
name: string;
age: number;
}
const user: Person = {
name: "John Doe",
age: 30,
};
In this example, the Person
interface ensures that any object of type Person
has both the name
and age
properties, and they must be of type string
and number
, respectively. Any violation of this contract will result in a compile-time error.
Understanding the Error: TS1394: Imported via {0} from file '{1}' with packageId '{2}'
This infamous error in TypeScript typically arises when there is some sort of type definition inconsistency or problem during module import. Let’s dissect this error in simpler, human-friendly terms and tackle how we can resolve it effectively.
The error message might look intimidating at first, but it's a helpful indicator that something isn't allowing TypeScript's type checker to properly resolve the types being imported in a module.
Example Scenario
Imagine you’re working with a shared library that includes pre-defined types, but when you try to import these types, you see the error TS1394: Imported via {0} from file '{1}' with packageId '{2}'.
Here is a basic example that could cause the error:
// utils.d.ts
export type User = {
id: string;
name: string;
};
// app.ts
import { User } from './utils'; // Error: TS1394
Possible reasons for the error:
-
Type Mismatch: The definition of
User
in the file being imported may be inconsistent (e.g., misspelling or incorrect property types). - Missing Definitions: TypeScript is unable to locate or resolve the type correctly due to incorrect pathing.
- Package Resolution Issue: If you're importing types from a library, it might be outdated or incorrectly set up.
Fixing TS1394: Imported via {0} from file '{1}' with packageId '{2}'
Here are step-by-step solutions to diagnose and fix this issue:
1. Ensure Correct Import Paths
Check if the file path and type names in the import statement are accurate.
// Fix if the file path is incorrect
import { User } from './utils';
2. Validate Type Definitions
Verify that the type definitions are valid and align with expectations.
// Correct definition
export type User = {
id: string; // Ensure `id` is a string, not anything else like number.
name: string;
};
3. Install/Update Dependencies
If the type comes from an external package, ensure the package is correctly installed and up-to-date.
npm install package-name --save
Also, ensure your tsconfig.json
correctly includes type declarations:
{
"compilerOptions": {
"typeRoots": ["node_modules/@types"]
}
}
Important to Know!
- TypeScript resolves types based on the
tsconfig.json
file. Make sure that your configuration specifies proper module resolution. - For external types from a package, always use the latest versions unless specified otherwise in your project.
FAQs About TS1394
1. What does "packageId" mean in TS1394?
The packageId
refers to the package where the type definitions come from. If it's a custom module, make sure that it is exported properly.
2. Can TypeScript check types across multiple files?
Yes, TypeScript can infer and enforce types across any number of files, as long as they're part of your project and linked through import/export
statements.
3. How do I debug TS1394 errors?
Start by checking your import paths, tsconfig.json
, and the type definition files (often .d.ts
files).
Important to Know!
- TypeScript relies heavily on proper export and import mechanics. All custom types must be explicitly exported from their respective files.
- When developing libraries, use
.d.ts
files to define clear and shareable type definitions.
Prerequisites for Fixing TS1394
- Basic understanding of TypeScript types and interfaces.
- Familiarity with the project structure (where files and dependencies are located).
- Knowledge of debugging in TypeScript (e.g., using
tsc
command for compilation).
By understanding and addressing the TS1394: Imported via {0} from file '{1}' with packageId '{2}' error, you can debug your TypeScript project efficiently while improving the overall quality of your codebase. Always remember that TypeScript errors, while sometimes verbose, are meant to guide you toward better practices.
If you'd like to delve deeper into TypeScript concepts like enums, type intersections, or how TypeScript works under the hood, don’t forget to subscribe to our blog. Also, explore GPTeach.us for an AI-assisted learning experience for mastering TypeScript today!