The problems I faced when coding a Pomodoro Timer with React.js
Introduction Recently, I was looking for web development ideas to kick off my new YouTube channel, Typing Zen, so I decided to code a simple Pomodoro timer app. After all, it shouldn't be too hard, right? Well, as it turns out, even a basic project can throw unexpected challenges your way. From handling circular progress bars without third-party libraries to dealing with JavaScript closures, I ran into some tricky problems that forced me to think outside the box. In this post, I'll share how I tackled these issues and what I learned along the way. The Circular Progress Bar My first challenge was the circular progress bar. Since I didn’t want to use any third-party libraries in my project—except for React and Tabler Icons (because nobody wants to handle SVG icons manually)—I quickly realized that implementing a circular progress bar wasn't as straightforward as I had thought. The solution I came up with was using an SVG path to manage the progress of the bar. Here’s the code I used: function CircularProgressBar({ progress, children }: CircularProgressBarProps) { const angle = progress * 2 * Math.PI; let path = ""; if (angle { // Some logic where we update the state // ... setActivity(newActivity); setLeftTime(newLeftTime); setSessionCount(newSessionCount); // Set a new timeout with the updated values setNewTimeout(newActivity, newLeftTime, newSessionCount); }; } useEffect(() => { return () => { clearTimeout(timeoutRef.current); }; }, []); function run() { setStatus("running"); setNewTimeout(activity, leftTime, sessionCount); } // ... } Conclusion Thank you for reading this far! If you're interested in the code, you can check out the GitHub repository: https://github.com/quibylix/pomodoro-timer. Additionally, if you enjoy mechanical keyboard sounds, relaxing videos, or just want to watch someone coding, feel free to check out my YouTube video where I build this app! Let me know in the comments if you've faced similar challenges or if you have suggestions for my future posts and projects. See you next time!
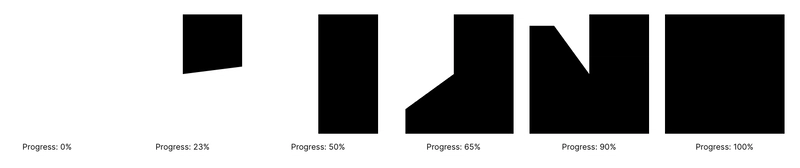
Introduction
Recently, I was looking for web development ideas to kick off my new YouTube channel, Typing Zen, so I decided to code a simple Pomodoro timer app. After all, it shouldn't be too hard, right? Well, as it turns out, even a basic project can throw unexpected challenges your way. From handling circular progress bars without third-party libraries to dealing with JavaScript closures, I ran into some tricky problems that forced me to think outside the box. In this post, I'll share how I tackled these issues and what I learned along the way.
The Circular Progress Bar
My first challenge was the circular progress bar. Since I didn’t want to use any third-party libraries in my project—except for React and Tabler Icons (because nobody wants to handle SVG icons manually)—I quickly realized that implementing a circular progress bar wasn't as straightforward as I had thought.
The solution I came up with was using an SVG path to manage the progress of the bar. Here’s the code I used:
function CircularProgressBar({ progress, children }: CircularProgressBarProps) {
const angle = progress * 2 * Math.PI;
let path = "";
if (angle <= Math.PI / 2) {
path = `M 50,50 V 0 H ${50 + 50 * Math.sin(angle)} V ${50 - 50 * Math.cos(angle)} Z`;
} else if (angle <= Math.PI) {
path = `M 50,50 V 0 H 100 V ${50 - 50 * Math.cos(angle)} H ${50 + 50 * Math.sin(angle)} Z`;
} else if (angle <= (3 * Math.PI) / 2) {
path = `M 50,50 V 0 H 100 V 100 H ${50 + 50 * Math.sin(angle)} V ${50 - 50 * Math.cos(angle)} Z`;
} else {
path = `M 50,50 V 0 H 100 V 100 H 0 V ${50 - 50 * Math.cos(angle)} H ${50 + 50 * Math.sin(angle)} Z`;
}
return (
<div className={styles.container}>
<svg className={styles.bar} viewBox="0 0 100 100">
<path d={path} />
svg>
<div className={styles.content}>{children}div>
div>
)
}
This solution creates a filled SVG path that dynamically colors the portion of the progress bar corresponding to a given progress level. Here are some examples:
After adding some styles:
.container {
display: flex;
justify-content: center;
align-items: center;
position: relative;
border-radius: 50%;
width: 15rem;
aspect-ratio: 1 / 1;
}
.bar {
fill: black;
position: absolute;
width: 100%;
height: 100%;
border-radius: 50%;
}
.content {
position: relative;
display: flex;
align-items: center;
justify-content: center;
width: calc(100% - 2rem);
aspect-ratio: 1 / 1;
border-radius: 50%;
background: #fff;
}
In my video, I added more colors and an animation for progress changes, but I consider this part to have been the hardest challenge.
JavaScript Closures and the Timer Issue
My second problem was dealing with JavaScript closures.
When I tried to program the timer using the setInterval function, I noticed that the global variables used inside the callback function remained unchanged, even when their values were updated outside the callback. This was especially problematic when working with useState.
After doing some research, I found that this issue is caused by how closures behave in JavaScript. To prevent issues with variables from outer scopes, closures only capture the initial value of a variable at the time of the first render.
To fix this, my solution was to use setTimeout instead of setInterval and generate a new callback function whenever the state was updated. Here’s how it works in code:
export function usePomodoroTimer() {
const [leftTime, setLeftTime] = useState(ACTIVITY_TIMES.working);
const [status, setStatus] = useState<PomodoroStatus>("stopped");
const [activity, setActivity] = useState<PomodoroActivity>("working");
const [sessionCount, setSessionCount] = useState(1);
const timeoutRef = useRef<number | undefined>(undefined);
// Instead of using global variables, we pass their values as parameters
function setNewTimeout(
activity: PomodoroActivity,
leftTime: number,
sessionCount: number,
) {
timeoutRef.current = setTimeout(
getTimeoutCallback(activity, leftTime, sessionCount),
1000,
);
}
function getTimeoutCallback(
activity: PomodoroActivity,
leftTime: number,
sessionCount: number,
) {
const initialTimestamp = Date.now();
return () => {
// Some logic where we update the state
// ...
setActivity(newActivity);
setLeftTime(newLeftTime);
setSessionCount(newSessionCount);
// Set a new timeout with the updated values
setNewTimeout(newActivity, newLeftTime, newSessionCount);
};
}
useEffect(() => {
return () => {
clearTimeout(timeoutRef.current);
};
}, []);
function run() {
setStatus("running");
setNewTimeout(activity, leftTime, sessionCount);
}
// ...
}
Conclusion
Thank you for reading this far!
If you're interested in the code, you can check out the GitHub repository:
https://github.com/quibylix/pomodoro-timer.
Additionally, if you enjoy mechanical keyboard sounds, relaxing videos, or just want to watch someone coding, feel free to check out my YouTube video where I build this app!
Let me know in the comments if you've faced similar challenges or if you have suggestions for my future posts and projects. See you next time!