The Dark Side of JavaScript: When the Magic Bites Back
JavaScript: the language we love to hate (and hate to love). It's the duct tape of the web — flexible, fast, forgiving… Too forgiving. Let’s take a walk down the alley where JavaScript goes from hero to horror: Type Coercion Madness [] + [] // '' [] + {} // '[object Object]' {} + [] // 0 (WHAT?) JavaScript will happily guess what you meant. Spoiler: it’s usually wrong. The "this" Trap const obj = { value: 10, getValue: function () { return this.value; } }; const get = obj.getValue; get(); // undefined (RIP 'this') One misstep with this and your logic collapses like a house of cards. Callback Hell Nested callbacks inside callbacks inside callbacks... Before async/await, JavaScript was basically a pasta dish. doSomething(function (res1) { doSomethingElse(res1, function (res2) { anotherThing(res2, function (res3) { //... }); }); }); Silent Errors const result = nonExistentFunction(); // JavaScript: “No worries, just crash and burn at runtime.” Implicit Globals function curseTheDay() { notDeclared = "oops"; // becomes global } Miss one let, and you've polluted the global scope. Equality Woes false == 0 // true '' == 0 // true null == undefined // true '0' == false // true Use == and JavaScript starts playing mind games. Monkey Patching Mayhem Array.prototype.toString = () => "I'm broken now."; Some devs just want to watch the world burn. Floating Point Fiascos 0.1 + 0.2 === 0.3 // false Thanks, IEEE 754. You had one job. Yet we keep coming back. Because despite the chaos, JavaScript is powerful, everywhere, and oddly… fun. But always remember: With great power comes great wOWs.
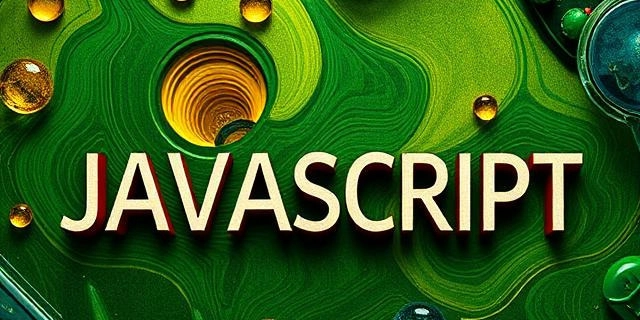
JavaScript: the language we love to hate (and hate to love).
It's the duct tape of the web — flexible, fast, forgiving…
Too forgiving.
Let’s take a walk down the alley where JavaScript goes from hero to horror:
Type Coercion Madness
[] + [] // ''
[] + {} // '[object Object]'
{} + [] // 0 (WHAT?)
JavaScript will happily guess what you meant.
Spoiler: it’s usually wrong.The "this" Trap
const obj = {
value: 10,
getValue: function () {
return this.value;
}
};
const get = obj.getValue;
get(); // undefined (RIP 'this')
One misstep with this and your logic collapses like a house of cards.
- Callback Hell Nested callbacks inside callbacks inside callbacks... Before async/await, JavaScript was basically a pasta dish.
doSomething(function (res1) {
doSomethingElse(res1, function (res2) {
anotherThing(res2, function (res3) {
//...
});
});
});
Silent Errors
const result = nonExistentFunction();
// JavaScript: “No worries, just crash and burn at runtime.”Implicit Globals
function curseTheDay() {
notDeclared = "oops"; // becomes global
}
Miss one let, and you've polluted the global scope.
- Equality Woes
false == 0 // true
'' == 0 // true
null == undefined // true
'0' == false // true
Use == and JavaScript starts playing mind games.
Monkey Patching Mayhem
Array.prototype.toString = () => "I'm broken now.";
Some devs just want to watch the world burn.Floating Point Fiascos
0.1 + 0.2 === 0.3 // false
Thanks, IEEE 754. You had one job.
Yet we keep coming back.
Because despite the chaos, JavaScript is powerful, everywhere, and oddly… fun.
But always remember:
With great power comes great wOWs.