Taming the Concurrency Beast: A Guide to Go's Goroutines and Channels
Introduction Remember when adding concurrency to your code meant choosing between anxiety attacks and caffeine addiction? After 20+ years in the IT trenches, I can assure you that Go's approach to concurrency feels like finding an oasis in the desert of thread management, mutex locks, and race conditions. If you've ever tried to juggle chainsaws while riding a unicycle, you probably have a good sense of what traditional concurrency feels like. Welcome to Go, where the chainsaws are replaced with fluffy gophers and the unicycle has training wheels. Let's dive into the refreshingly practical world of goroutines and channels! 1. Goroutines: Lightweight Threads on Steroids Goroutines are to traditional threads what hummingbirds are to cargo planes - they're tiny, nimble, and you can have thousands of them without breaking a sweat. Fun fact: While a typical OS thread might consume about 1MB of memory, a goroutine starts at a mere 2KB. This isn't just a small difference; it's like comparing a mansion to a studio apartment! The Go runtime includes a sophisticated scheduler that manages goroutines across available OS threads. This scheduler has undergone major revisions (in versions 1.1, 1.5, and 1.14) to become increasingly efficient. The 1.14 update was particularly significant as it introduced asynchronous preemption to prevent long-running goroutines from hogging the scheduler. Creating a goroutine is delightfully simple: func main() { // Launch 10,000 goroutines (try this with OS threads, I dare you) for i := 0; i
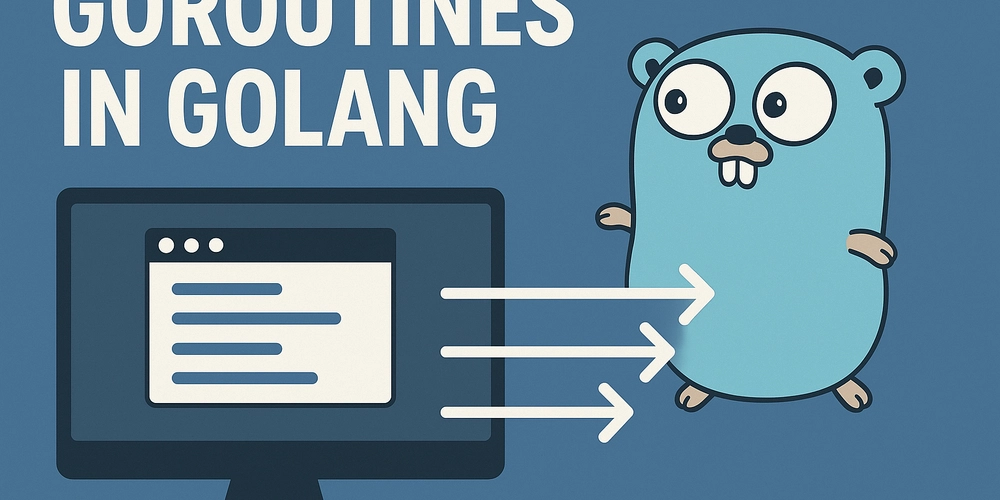
Introduction
Remember when adding concurrency to your code meant choosing between anxiety attacks and caffeine addiction? After 20+ years in the IT trenches, I can assure you that Go's approach to concurrency feels like finding an oasis in the desert of thread management, mutex locks, and race conditions.
If you've ever tried to juggle chainsaws while riding a unicycle, you probably have a good sense of what traditional concurrency feels like. Welcome to Go, where the chainsaws are replaced with fluffy gophers and the unicycle has training wheels. Let's dive into the refreshingly practical world of goroutines and channels!
1. Goroutines: Lightweight Threads on Steroids
Goroutines are to traditional threads what hummingbirds are to cargo planes - they're tiny, nimble, and you can have thousands of them without breaking a sweat.
Fun fact: While a typical OS thread might consume about 1MB of memory, a goroutine starts at a mere 2KB. This isn't just a small difference; it's like comparing a mansion to a studio apartment!
The Go runtime includes a sophisticated scheduler that manages goroutines across available OS threads. This scheduler has undergone major revisions (in versions 1.1, 1.5, and 1.14) to become increasingly efficient. The 1.14 update was particularly significant as it introduced asynchronous preemption to prevent long-running goroutines from hogging the scheduler.
Creating a goroutine is delightfully simple:
func main() {
// Launch 10,000 goroutines (try this with OS threads, I dare you)
for i := 0; i < 10000; i++ {
go func(id int) {
fmt.Printf("Hello from goroutine %d\n", id)
}(i)
}
// Wait to ensure goroutines have time to complete
// (We'll learn better synchronization methods shortly)
time.Sleep(time.Second)
}
The biggest rookie mistake? Forgetting that your main()
function doesn't wait for goroutines to finish before exiting. It's like being the parent who accidentally leaves the playground while their kids are still on the swings.