Taming local git branches
On rare occasions, I write a blog post that's not about MiniScript. Today is one of those! It addresses a problem that I've often faced at work: keeping track of all my local git branches. The Problem At work, I have a couple of git repositories that my team works on extensively. We're a small team and have to wear a lot of hats, so I often have to switch from one task to another throughout the day. Often that means switching git branches, too. I try to remember to delete my local branches whenever I've finished a task, made a PR, and seen that PR merged to main, but sometimes I forget. Pretty soon, my git branch output looks like this: * joe/feat-remap-ids joe/feat-seg-lookup joe/feat_806 joe/feat_dacey_stuff joe/fix_syn_assignment joe/hotfix-synapses joe/mito-split-fix joe/pylint_timesink joe/seg-savvy-cc joe/seg-savvy-cc-clean joe/syn_neighbor joe/synapseflow main (Actually there's more than that; I've trimmed it down for the sake of this post!) And I can no longer remember what's going on with all of these: were they already merged to main, or does that need to happen? Or are they experimental code I never intended to merge at all? Making a mistake in either direction is very costly, so when I decide it's "clean up" time, I spend all day studying each branch and figuring out what's going on with it. The Solution Today I've finally found a solution that I think will help. You can associate a "description" with each local branch. And, by clever use of git aliases, you can make a command to list branches with these descriptions. I'll restrict my descriptions to these codes: EXPLORATION — trying something out that I may not want to keep PERSONAL — want to keep around but only for my own use WIP — real work, still in progress (may include ticket# if any) PR #123 — pull request submitted Add a git desc command You can edit the description of the current branch with git branch --edit-description, but I am lazy and that's too much to type and remember. So, do this just once: git config --global alias.desc "branch --edit-description" ...and now you can simply type git desc to edit this description. This pops up your preferred editor (the same one you use to type commit comments) and lets you enter or edit the description. This is where I would type WIP or whatever. Add a git branch-status command Similarly, we're going to add an alias to list branches — like the usual git branch command — but with the descriptions appended. And since I work on a standard terminal, we'll use terminal escape codes to even color it nicely. The command is a long one, so copy/paste carefully: git config --global alias.branch-status '!current=$(git symbolic-ref --short HEAD 2>/dev/null || echo "detached") && for branch in $(git branch --format="%(refname:short)"); do if [ "$branch" = "$current" ]; then printf "* \033[1;32m%s\033[0m - %s\n" "$branch" "$(git config branch.$branch.description || echo "No description")"; else printf " \033[1;33m%s\033[0m - %s\n" "$branch" "$(git config branch.$branch.description || echo "No description")"; fi; done' The result looks like this: As you can see, each branch is shown along with its current description, or "No description" if none has been entered. The branch names are in yellow, except for one you're currently in, which is green and also highlighted with an asterisk. These new commands are stored in your global git config file (~/.gitconfig), so you only need to do the above setup once. If you work on multiple machines, of course you can do them on each machine, or just copy your .gitconfig file over. Clarity at last! With these now git desc and git branch-status commands, I have a way to keep track of what's going on with each branch as it moves from vague idea, to real work, to pull request. No more will I have to look at a long list of branches and try to remember what's going on with each of them. It's important to note that these descriptions are only stored locally; they do not go to the server when you push. They're notes for your own use, which I think is just perfect. It means you don't need approval from your coworkers; you can start using this today, and let your coworkers wonder how you stay so organized!
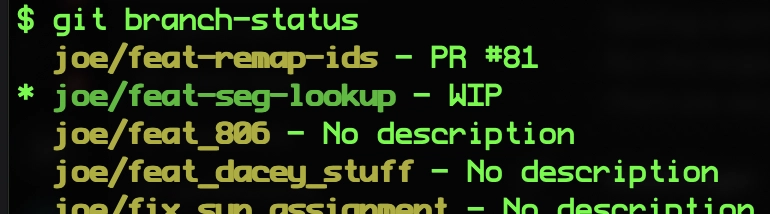
On rare occasions, I write a blog post that's not about MiniScript. Today is one of those! It addresses a problem that I've often faced at work: keeping track of all my local git branches.
The Problem
At work, I have a couple of git repositories that my team works on extensively. We're a small team and have to wear a lot of hats, so I often have to switch from one task to another throughout the day. Often that means switching git branches, too. I try to remember to delete my local branches whenever I've finished a task, made a PR, and seen that PR merged to main, but sometimes I forget. Pretty soon, my git branch
output looks like this:
* joe/feat-remap-ids
joe/feat-seg-lookup
joe/feat_806
joe/feat_dacey_stuff
joe/fix_syn_assignment
joe/hotfix-synapses
joe/mito-split-fix
joe/pylint_timesink
joe/seg-savvy-cc
joe/seg-savvy-cc-clean
joe/syn_neighbor
joe/synapseflow
main
(Actually there's more than that; I've trimmed it down for the sake of this post!) And I can no longer remember what's going on with all of these: were they already merged to main, or does that need to happen? Or are they experimental code I never intended to merge at all? Making a mistake in either direction is very costly, so when I decide it's "clean up" time, I spend all day studying each branch and figuring out what's going on with it.
The Solution
Today I've finally found a solution that I think will help. You can associate a "description" with each local branch. And, by clever use of git aliases, you can make a command to list branches with these descriptions. I'll restrict my descriptions to these codes:
- EXPLORATION — trying something out that I may not want to keep
- PERSONAL — want to keep around but only for my own use
- WIP — real work, still in progress (may include ticket# if any)
- PR #123 — pull request submitted
Add a git desc
command
You can edit the description of the current branch with git branch --edit-description
, but I am lazy and that's too much to type and remember. So, do this just once:
git config --global alias.desc "branch --edit-description"
...and now you can simply type git desc
to edit this description. This pops up your preferred editor (the same one you use to type commit comments) and lets you enter or edit the description. This is where I would type WIP
or whatever.
Add a git branch-status
command
Similarly, we're going to add an alias to list branches — like the usual git branch
command — but with the descriptions appended. And since I work on a standard terminal, we'll use terminal escape codes to even color it nicely. The command is a long one, so copy/paste carefully:
git config --global alias.branch-status '!current=$(git symbolic-ref --short HEAD 2>/dev/null || echo "detached") && for branch in $(git branch --format="%(refname:short)"); do if [ "$branch" = "$current" ]; then printf "* \033[1;32m%s\033[0m - %s\n" "$branch" "$(git config branch.$branch.description || echo "No description")"; else printf " \033[1;33m%s\033[0m - %s\n" "$branch" "$(git config branch.$branch.description || echo "No description")"; fi; done'
The result looks like this:
As you can see, each branch is shown along with its current description, or "No description" if none has been entered. The branch names are in yellow, except for one you're currently in, which is green and also highlighted with an asterisk.
These new commands are stored in your global git config file (~/.gitconfig
), so you only need to do the above setup once. If you work on multiple machines, of course you can do them on each machine, or just copy your .gitconfig
file over.
Clarity at last!
With these now git desc
and git branch-status
commands, I have a way to keep track of what's going on with each branch as it moves from vague idea, to real work, to pull request. No more will I have to look at a long list of branches and try to remember what's going on with each of them.
It's important to note that these descriptions are only stored locally; they do not go to the server when you push. They're notes for your own use, which I think is just perfect. It means you don't need approval from your coworkers; you can start using this today, and let your coworkers wonder how you stay so organized!