Spring Boot with Spotless and Git Pre-commit Hooks
This guide walks you through setting up automated code formatting and pre-commit checks in a Spring Boot Maven project, ensuring consistent code style across your team. Table of Contents Introduction Prerequisites Step 1: Set Up a Spring Boot Project Step 2: Configure Spotless Maven Plugin Step 3: Install Git Hooks with Maven Step 4: Adding Git Commit ID Plugin Step 5: Full POM Example Step 6: Using the Configuration Step 7: Customization Options Troubleshooting Introduction Having consistent code style across a project improves readability and maintainability. This guide combines three powerful tools: Spotless: A code formatter that enforces style rules Git Pre-commit Hooks: Automatically checks or formats code before commits Maven: Integrates these tools into your build process Prerequisites Java 8 or higher Maven 3.6.0 or higher Git installed and initialized in your project A Spring Boot project (or willingness to create one) Step 1: Set Up a Spring Boot Project If you don't already have a Spring Boot project, create one using Spring Initializr or with the following Maven command: mvn archetype:generate -DgroupId=com.example -DartifactId=demo -DarchetypeArtifactId=maven-archetype-quickstart -DarchetypeVersion=1.4 -DinteractiveMode=false Make sure to initialize Git in your project directory: git init Step 2: Configure Spotless Maven Plugin Add the Spotless Maven plugin to your pom.xml file in the section: com.diffplug.spotless spotless-maven-plugin 2.40.0 src/main/java/**/*.java src/test/java/**/*.java 1.16.0 GOOGLE java,javax,org,com, check compile This configuration: Uses Google's Java code style Removes unused imports Organizes imports in a specific order Checks formatting during compilation Step 3: Install Git Hooks with Maven Add the Git Build Hook Maven plugin to manage Git hooks: com.rudikershaw.gitbuildhook git-build-hook-maven-plugin 3.4.1 mvn spotless:apply install initialize This plugin: Installs Git hooks during the initialize phase Sets up a pre-commit hook that runs mvn spotless:apply before each commit Step 4: Adding Git Commit ID Plugin Add the Git Commit ID plugin to include Git information in your build: io.github.git-commit-id git-commit-id-maven-plugin 6.0.0 get-the-git-infos revision initialize true ${project.build.outputDirectory}/git.properties ^git.branch$ ^git.commit.id$ ^git.commit.time$ This adds Git metadata to your build, which can be useful for tracking versions and build information. Step 5: Full POM Example Here's a complete pom.xml example for a Spring Boot project with all these configurations: 4.0.0 org.springframework.boot spring-boot-starter-parent 3.2.3 com.example demo 0.0.1-SNAPSHOT demo Demo Spring Boot project with Spotless and Git hooks 17 org.springframework.boot spring-boot-starter-web org.springframework.boot spring-boot-starter-test test org.springframework.boot spring-boot-maven-plugin com.diffplug.spotless spotless-maven-plugin 2.40.0 src/main/java/**/*.java src/test/java/**/*.java 1.16.0 GOOGLE java,javax,org,com, check compile io.github.git-commit-id git-commit-id-maven-plugin 6.0.0 get-the-git-infos revision
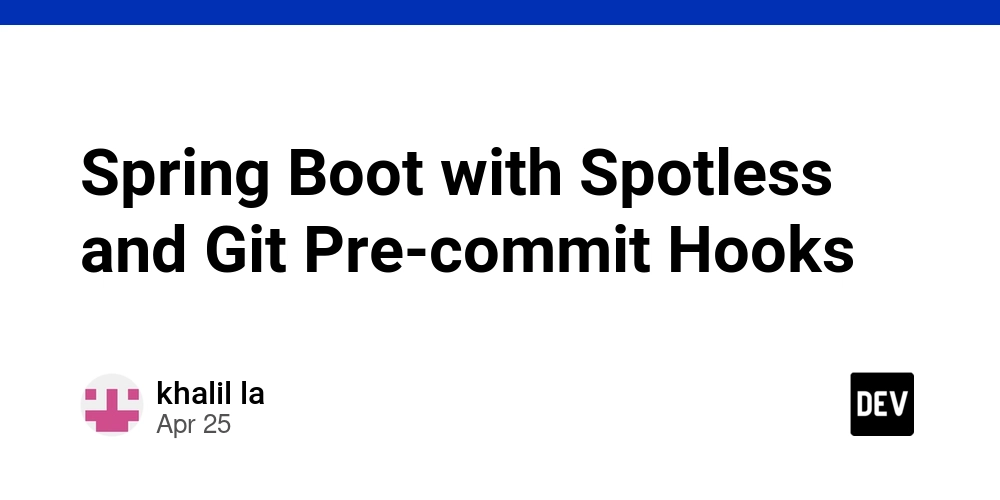
This guide walks you through setting up automated code formatting and pre-commit checks in a Spring Boot Maven project, ensuring consistent code style across your team.
Table of Contents
- Introduction
- Prerequisites
- Step 1: Set Up a Spring Boot Project
- Step 2: Configure Spotless Maven Plugin
- Step 3: Install Git Hooks with Maven
- Step 4: Adding Git Commit ID Plugin
- Step 5: Full POM Example
- Step 6: Using the Configuration
- Step 7: Customization Options
- Troubleshooting
Introduction
Having consistent code style across a project improves readability and maintainability. This guide combines three powerful tools:
- Spotless: A code formatter that enforces style rules
- Git Pre-commit Hooks: Automatically checks or formats code before commits
- Maven: Integrates these tools into your build process
Prerequisites
- Java 8 or higher
- Maven 3.6.0 or higher
- Git installed and initialized in your project
- A Spring Boot project (or willingness to create one)
Step 1: Set Up a Spring Boot Project
If you don't already have a Spring Boot project, create one using Spring Initializr or with the following Maven command:
mvn archetype:generate -DgroupId=com.example -DartifactId=demo -DarchetypeArtifactId=maven-archetype-quickstart -DarchetypeVersion=1.4 -DinteractiveMode=false
Make sure to initialize Git in your project directory:
git init
Step 2: Configure Spotless Maven Plugin
Add the Spotless Maven plugin to your pom.xml
file in the
section:
com.diffplug.spotless
spotless-maven-plugin
2.40.0
src/main/java/**/*.java
src/test/java/**/*.java
1.16.0
GOOGLE
/>
java,javax,org,com,
check
compile
This configuration:
- Uses Google's Java code style
- Removes unused imports
- Organizes imports in a specific order
- Checks formatting during compilation
Step 3: Install Git Hooks with Maven
Add the Git Build Hook Maven plugin to manage Git hooks:
com.rudikershaw.gitbuildhook
git-build-hook-maven-plugin
3.4.1
mvn spotless:apply
install
initialize
This plugin:
- Installs Git hooks during the initialize phase
- Sets up a pre-commit hook that runs
mvn spotless:apply
before each commit
Step 4: Adding Git Commit ID Plugin
Add the Git Commit ID plugin to include Git information in your build:
io.github.git-commit-id
git-commit-id-maven-plugin
6.0.0
get-the-git-infos
revision
initialize
true
${project.build.outputDirectory}/git.properties
^git.branch$
^git.commit.id$
^git.commit.time$
This adds Git metadata to your build, which can be useful for tracking versions and build information.
Step 5: Full POM Example
Here's a complete pom.xml
example for a Spring Boot project with all these configurations:
xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
4.0.0
org.springframework.boot
spring-boot-starter-parent
3.2.3
com.example
demo
0.0.1-SNAPSHOT
demo
Demo Spring Boot project with Spotless and Git hooks
17
org.springframework.boot
spring-boot-starter-web
org.springframework.boot
spring-boot-starter-test
test
org.springframework.boot
spring-boot-maven-plugin
com.diffplug.spotless
spotless-maven-plugin
2.40.0
src/main/java/**/*.java
src/test/java/**/*.java
1.16.0
GOOGLE
/>
java,javax,org,com,
check
compile
io.github.git-commit-id
git-commit-id-maven-plugin
6.0.0
get-the-git-infos
revision
initialize
true
${project.build.outputDirectory}/git.properties
^git.branch$
^git.commit.id$
^git.commit.time$
com.rudikershaw.gitbuildhook
git-build-hook-maven-plugin
3.4.1
mvn spotless:apply
install
initialize
Step 6: Using the Configuration
After adding these configurations to your pom.xml
:
- Install the Git hooks:
mvn initialize
- Verify the pre-commit hook is installed:
cat .git/hooks/pre-commit
You should see something that includes the command mvn spotless:apply
.
-
Now when you try to commit code:
- The pre-commit hook will automatically run
mvn spotless:apply
- This will format your code according to the configured style
- Then the commit will proceed
- The pre-commit hook will automatically run
-
Manual Spotless commands:
- Check for formatting issues without changing files:
mvn spotless:check
- Apply formatting to all files:
mvn spotless:apply
- Check for formatting issues without changing files:
Step 7: Customization Options
Alternative Formatting Styles
If you prefer the Eclipse formatter instead of Google's style:
${project.basedir}/eclipse-formatter.xml
You'll need to provide an Eclipse formatter configuration file.
Formatting Other File Types
Add formatters for additional file types:
src/main/**/*.xml
pom.xml
src/main/resources/**/*.yml
src/main/resources/**/*.yaml
Custom Import Order
Customize the import order to match your team's preferences:
com.yourcompany,com,org,javax,java,\#
Different Pre-commit Actions
To run both formatting check and tests in pre-commit:
mvn spotless:apply test
Troubleshooting
Pre-commit Hook Not Running
If the pre-commit hook doesn't run automatically:
- Check that the hook file has execution permissions:
chmod +x .git/hooks/pre-commit
- Verify Git hooks are not being ignored:
git config core.hooksPath
It should return .git/hooks
or be empty.
Spotless Fails to Apply Formatting
If Spotless fails with formatting errors:
- Run
mvn spotless:apply
manually to see detailed error messages - Check if your code has syntax errors that prevent formatting
- Ensure you're using a compatible Java version for the configured formatter
Git Commit ID Plugin Fails
If the Git Commit ID plugin fails:
- Ensure your project is a Git repository (
git init
) - Make at least one initial commit before running the plugin
- Check if Git is properly installed and accessible
For more advanced options and configurations, refer to the official documentation: