Solving the Two Sum Problem in Rust: Brute Force vs HashMap
I’ve been diving into Rust and decided to challenge myself with some LeetCode problems. One of the first ones I tackled was the classic Two Sum problem — and, it turned out to be a great way to practice Rust basics like iterating over vectors and using HashMap. In this post, I’ll walk you through how I approached the problem first with a brute-force solution, and then optimized it using a hash map. I’ll also break down what “time complexity” really means as we go. The Problem You're given an array of integers nums and a target integer target. Your task is to: Return the indices of the two numbers such that they add up to the target. Constraints: Each input has exactly one solution. You can’t use the same element twice. You can return the answer in any order.
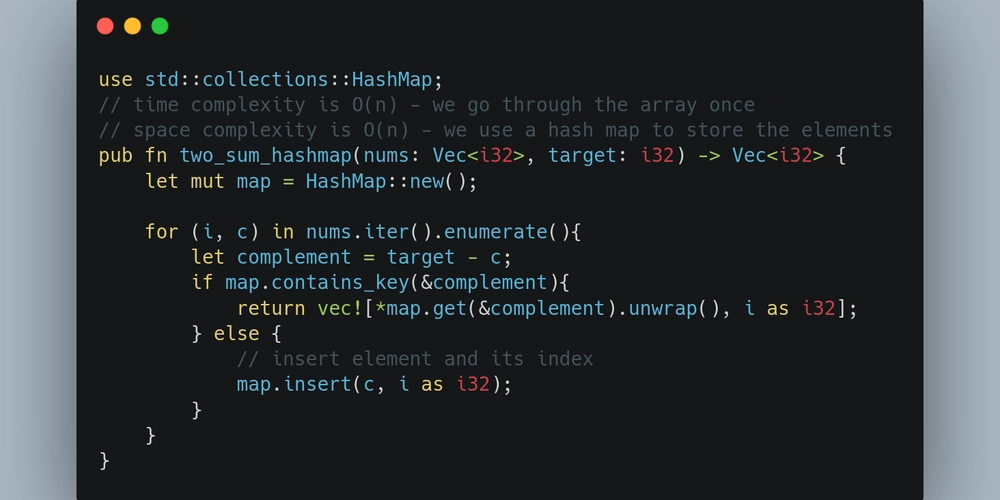
I’ve been diving into Rust and decided to challenge myself with some LeetCode problems. One of the first ones I tackled was the classic Two Sum problem — and, it turned out to be a great way to practice Rust basics like iterating over vectors and using HashMap.
In this post, I’ll walk you through how I approached the problem first with a brute-force solution, and then optimized it using a hash map. I’ll also break down what “time complexity” really means as we go.
The Problem
You're given an array of integers nums and a target integer target. Your task is to:
Return the indices of the two numbers such that they add up to the target.
Constraints:
- Each input has exactly one solution.
- You can’t use the same element twice.
- You can return the answer in any order.