Single Quotes vs Double Quotes in YAML: How They Differ From JavaScript and TypeScript
Note: AI was used to help me out to clean up the article When working with YAML files for configuration, one of the most common sources of confusion is understanding how string quoting works. If you're coming from JavaScript or TypeScript, you might assume quotes work the same way, but there are some important differences that can trip you up. Let's look at how string quoting differs between these languages and how to avoid common pitfalls. String Quoting in YAML In YAML, both single quotes (') and double quotes (") can be used to define strings, but they behave differently: Double Quotes in YAML Double-quoted strings in YAML support escape sequences, which means you can use special characters like: message: "Hello\nWorld" # Includes a line break path: "C:\\Program Files\\App" # Escapes backslashes special: "Quote \"inside\" a string" # Escapes quotes Single Quotes in YAML Single-quoted strings treat characters literally, with the only exception being that you can escape a single quote by doubling it: message: 'Hello\nWorld' # \n appears literally as "\n", not a line break path: 'C:\Program Files\App' # Backslashes are preserved as-is special: 'Quote ''inside'' a string' # Escape single quotes by doubling them How This Differs From JavaScript/TypeScript In JavaScript and TypeScript, both single and double quotes behave almost identically: const doubleQuoted = "Hello\nWorld"; // Contains a line break const singleQuoted = 'Hello\nWorld'; // Also contains a line break! // Both allow escape sequences const doubleBackslash = "C:\\Program Files\\App"; const singleBackslash = 'C:\\Program Files\\App'; // But you need to escape the quote you're using const doubleQuoteEscape = "Quote \"inside\" a string"; const singleQuoteEscape = 'Quote \'inside\' a string'; The key difference is that in JavaScript/TypeScript, both quote types support the same escape sequences while in YAML, only double quotes do! Common Gotchas This difference often causes issues when: Working with paths: Windows file paths with backslashes can cause problems # Wrong - in double quotes you need to escape backslashes windows_path: "C:\Program Files\My App" # Will be interpreted as "C:Program FilesMy App" # Correct approaches windows_path1: "C:\\Program Files\\My App" # Double each backslash windows_path2: 'C:\Program Files\My App' # Use single quotes (safer for paths) windows_path3: C:\Program Files\My App # Unquoted (works for simple paths) Multiline strings: Newlines are treated differently # In double quotes, \n creates an actual line break description: "First line\nSecond line" # In single quotes, \n is just the characters \ and n description: 'First line\nSecond line' # Will be "First line\nSecond line" Special characters: Some YAML values need quotes to avoid being interpreted as other types # Without quotes, these might be parsed as boolean or numeric values value1: "yes" # String "yes" (not boolean true) value2: "no" # String "no" (not boolean false) value3: "1.0" # String "1.0" (not float 1.0) Practical Recommendations Based on these differences, here are some practical tips: Use single quotes for paths (especially Windows paths) to avoid escaping backslashes Use double quotes when you need escape sequences like \n, \t, etc. When in doubt, quote your strings to prevent YAML from interpreting them as non-string values Be consistent within your project - pick a quoting style and stick with it In Summary Unlike JavaScript and TypeScript, where single and double quotes work almost identically, YAML treats them quite differently: Feature YAML Single Quotes YAML Double Quotes JS/TS Single Quotes JS/TS Double Quotes Escape sequences No (literal) Yes Yes Yes Escaping quotes Double it ('') Backslash (\") Backslash (\') Backslash (\") Multiline support Yes (special syntax) Yes (with \n) Yes (with \n) Yes (with \n) Understanding these differences is crucial when working with applications that use both JavaScript/TypeScript code and YAML configuration files, such as Kubernetes, Docker Compose, or GitHub Actions workflows. Happy coding!
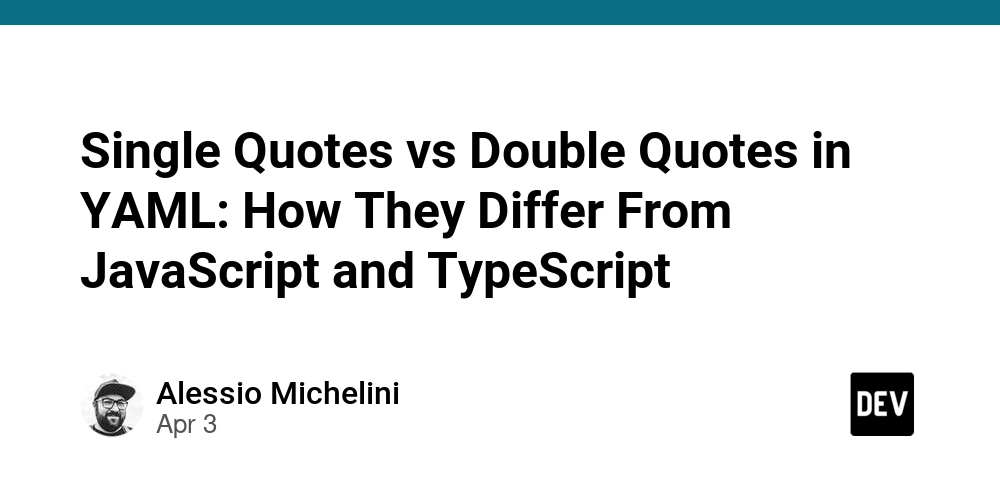
Note: AI was used to help me out to clean up the article
When working with YAML files for configuration, one of the most common sources of confusion is understanding how string quoting works. If you're coming from JavaScript or TypeScript, you might assume quotes work the same way, but there are some important differences that can trip you up. Let's look at how string quoting differs between these languages and how to avoid common pitfalls.
String Quoting in YAML
In YAML, both single quotes ('
) and double quotes ("
) can be used to define strings, but they behave differently:
Double Quotes in YAML
Double-quoted strings in YAML support escape sequences, which means you can use special characters like:
message: "Hello\nWorld" # Includes a line break
path: "C:\\Program Files\\App" # Escapes backslashes
special: "Quote \"inside\" a string" # Escapes quotes
Single Quotes in YAML
Single-quoted strings treat characters literally, with the only exception being that you can escape a single quote by doubling it:
message: 'Hello\nWorld' # \n appears literally as "\n", not a line break
path: 'C:\Program Files\App' # Backslashes are preserved as-is
special: 'Quote ''inside'' a string' # Escape single quotes by doubling them
How This Differs From JavaScript/TypeScript
In JavaScript and TypeScript, both single and double quotes behave almost identically:
const doubleQuoted = "Hello\nWorld"; // Contains a line break
const singleQuoted = 'Hello\nWorld'; // Also contains a line break!
// Both allow escape sequences
const doubleBackslash = "C:\\Program Files\\App";
const singleBackslash = 'C:\\Program Files\\App';
// But you need to escape the quote you're using
const doubleQuoteEscape = "Quote \"inside\" a string";
const singleQuoteEscape = 'Quote \'inside\' a string';
The key difference is that in JavaScript/TypeScript, both quote types support the same escape sequences while in YAML, only double quotes do!
Common Gotchas
This difference often causes issues when:
- Working with paths: Windows file paths with backslashes can cause problems
# Wrong - in double quotes you need to escape backslashes
windows_path: "C:\Program Files\My App" # Will be interpreted as "C:Program FilesMy App"
# Correct approaches
windows_path1: "C:\\Program Files\\My App" # Double each backslash
windows_path2: 'C:\Program Files\My App' # Use single quotes (safer for paths)
windows_path3: C:\Program Files\My App # Unquoted (works for simple paths)
- Multiline strings: Newlines are treated differently
# In double quotes, \n creates an actual line break
description: "First line\nSecond line"
# In single quotes, \n is just the characters \ and n
description: 'First line\nSecond line' # Will be "First line\nSecond line"
- Special characters: Some YAML values need quotes to avoid being interpreted as other types
# Without quotes, these might be parsed as boolean or numeric values
value1: "yes" # String "yes" (not boolean true)
value2: "no" # String "no" (not boolean false)
value3: "1.0" # String "1.0" (not float 1.0)
Practical Recommendations
Based on these differences, here are some practical tips:
- Use single quotes for paths (especially Windows paths) to avoid escaping backslashes
-
Use double quotes when you need escape sequences like
\n
,\t
, etc. - When in doubt, quote your strings to prevent YAML from interpreting them as non-string values
- Be consistent within your project - pick a quoting style and stick with it
In Summary
Unlike JavaScript and TypeScript, where single and double quotes work almost identically, YAML treats them quite differently:
Feature | YAML Single Quotes | YAML Double Quotes | JS/TS Single Quotes | JS/TS Double Quotes |
---|---|---|---|---|
Escape sequences | No (literal) | Yes | Yes | Yes |
Escaping quotes | Double it ('' ) |
Backslash (\" ) |
Backslash (\' ) |
Backslash (\" ) |
Multiline support | Yes (special syntax) | Yes (with \n ) |
Yes (with \n ) |
Yes (with \n ) |
Understanding these differences is crucial when working with applications that use both JavaScript/TypeScript code and YAML configuration files, such as Kubernetes, Docker Compose, or GitHub Actions workflows.
Happy coding!