Sign JPG Images with Text Signatures in C# Using .NET REST API
Developers aiming to incorporate text signatures into JPG images within .NET applications can utilize the GroupDocs.Signature Cloud .NET SDK, making it easy to add text-based e-signatures to JPG files. Whether building document management systems, automating business processes, or bolstering app security, this powerful Cloud API for .NET allows you to programmatically sign images without any complicated configuration. With its user-friendly API structure, you can create, modify, and apply text signatures to JPG files using only a few simple API requests. There’s no requirement for manual modifications or additional software; everything is efficiently executed within your C# codebase. Furthermore, the SDK’s cloud-native design guarantees reliability, scalability, and exceptional performance across all your .NET applications. Enhance your applications with secure text signing for JPGs, optimize your image processing workflows, and provide your users with a quicker, more professional experience. Discover how effortless it is to implement text signatures on JPG images in .NET by exploring this comprehensive tutorial that outlines a reliable REST API solution. Here is a C# code sample to help you begin with this capability: using GroupDocs.Signature.Cloud.Sdk.Api; using GroupDocs.Signature.Cloud.Sdk.Client; using GroupDocs.Signature.Cloud.Sdk.Model; using GroupDocs.Signature.Cloud.Sdk.Model.Requests; class Program { static void Main(string[] args) { // Configure API credentials string MyClientId = "your-client-id"; string MyClientSecret = "your-client-secret"; var configuration = new Configuration(MyClientId, MyClientSecret); // Initialize the SignApi class for adding e-signatures var signatureApi = new SignApi(configuration); // Set up text signature options var textOptions = new SignTextOptions { SignatureType = SignOptions.SignatureTypeEnum.Text, Text = "Image eSignature", Left = 100, Top = 200, Width = 150, Height = 100, Margin = new Padding { Top = 5, Bottom = 5 }, LocationMeasureType = SignTextOptions.LocationMeasureTypeEnum.Pixels, SizeMeasureType = SignTextOptions.SizeMeasureTypeEnum.Pixels, Font = new SignatureFont { FontFamily = "Times New Roman", FontSize = 12, Italic = true }, ForeColor = new Color { Web = "Blue" }, }; // Configure text e-signature settings var signSettings = new SignSettings { FileInfo = new GroupDocs.Signature.Cloud.Sdk.Model.FileInfo { // Source file path in cloud storage FilePath = "SampleFiles/source.jpg" }, SaveOptions = new SaveOptions { OutputFilePath = "signed/source-signed.jpg", }, Options = new List { textOptions } }; // Create and execute the text signature request var request = new CreateSignaturesRequest(signSettings); var response = signatureApi.CreateSignatures(request); } }
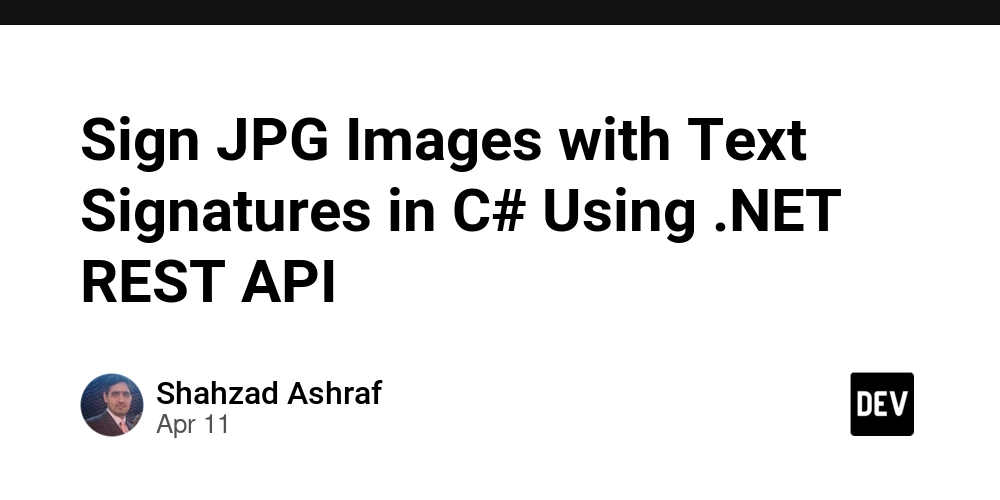
Developers aiming to incorporate text signatures into JPG images within .NET applications can utilize the GroupDocs.Signature Cloud .NET SDK, making it easy to add text-based e-signatures to JPG files. Whether building document management systems, automating business processes, or bolstering app security, this powerful Cloud API for .NET allows you to programmatically sign images without any complicated configuration.
With its user-friendly API structure, you can create, modify, and apply text signatures to JPG files using only a few simple API requests. There’s no requirement for manual modifications or additional software; everything is efficiently executed within your C# codebase. Furthermore, the SDK’s cloud-native design guarantees reliability, scalability, and exceptional performance across all your .NET applications.
Enhance your applications with secure text signing for JPGs, optimize your image processing workflows, and provide your users with a quicker, more professional experience. Discover how effortless it is to implement text signatures on JPG images in .NET by exploring this comprehensive tutorial that outlines a reliable REST API solution.
Here is a C# code sample to help you begin with this capability:
using GroupDocs.Signature.Cloud.Sdk.Api;
using GroupDocs.Signature.Cloud.Sdk.Client;
using GroupDocs.Signature.Cloud.Sdk.Model;
using GroupDocs.Signature.Cloud.Sdk.Model.Requests;
class Program
{
static void Main(string[] args)
{
// Configure API credentials
string MyClientId = "your-client-id";
string MyClientSecret = "your-client-secret";
var configuration = new Configuration(MyClientId, MyClientSecret);
// Initialize the SignApi class for adding e-signatures
var signatureApi = new SignApi(configuration);
// Set up text signature options
var textOptions = new SignTextOptions
{
SignatureType = SignOptions.SignatureTypeEnum.Text,
Text = "Image eSignature",
Left = 100,
Top = 200,
Width = 150,
Height = 100,
Margin = new Padding { Top = 5, Bottom = 5 },
LocationMeasureType = SignTextOptions.LocationMeasureTypeEnum.Pixels,
SizeMeasureType = SignTextOptions.SizeMeasureTypeEnum.Pixels,
Font = new SignatureFont
{
FontFamily = "Times New Roman",
FontSize = 12,
Italic = true
},
ForeColor = new Color { Web = "Blue" },
};
// Configure text e-signature settings
var signSettings = new SignSettings
{
FileInfo = new GroupDocs.Signature.Cloud.Sdk.Model.FileInfo
{
// Source file path in cloud storage
FilePath = "SampleFiles/source.jpg"
},
SaveOptions = new SaveOptions
{
OutputFilePath = "signed/source-signed.jpg",
},
Options = new List { textOptions }
};
// Create and execute the text signature request
var request = new CreateSignaturesRequest(signSettings);
var response = signatureApi.CreateSignatures(request);
}
}