"SensorClean: Step-by-Step Guide to IoT Hygiene Monitoring in Commercial Spaces"
Introduction Maintaining hygiene in commercial spaces has become more crucial than ever. Traditional cleaning methods often lack efficiency and real-time monitoring. Enter SensorClean, an innovative IoT-based solution designed to revolutionize commercial cleaning services. This system enables real-time tracking, automated alerts, and efficient hygiene management through smart sensors and cloud-based analytics. In this blog post, we'll explore how to implement SensorClean step by step, with relevant code snippets and best practices to ensure a seamless deployment. Why IoT for Cleaning Services? IoT technology allows businesses to optimize their cleaning processes, reduce costs, and maintain compliance with hygiene standards. Some key benefits include: Real-time monitoring: Track cleanliness levels in different areas. Automated scheduling: Ensure cleaning tasks are performed consistently. Data-driven insights: Optimize resources based on usage trends. Now, let's dive into building an IoT-powered hygiene monitoring system using SensorClean. Step 1: Setting Up IoT Sensors To get started, we'll use smart sensors that detect cleanliness levels through air quality, surface bacteria, and dust accumulation. These sensors can be integrated with a microcontroller such as Raspberry Pi or ESP8266. Required Hardware Raspberry Pi / ESP8266 Air quality sensor (MQ135) Optical dust sensor (GP2Y1010AU0F) Temperature and humidity sensor (DHT22) Wi-Fi module Connecting the Sensors import Adafruit_DHT import time dht_sensor = Adafruit_DHT.DHT22 sensor_pin = 4 while True: humidity, temperature = Adafruit_DHT.read_retry(dht_sensor, sensor_pin) if humidity and temperature: print(f"Temp: {temperature:.2f}C, Humidity: {humidity:.2f}%") time.sleep(5) This Python script reads temperature and humidity data, which helps assess environmental conditions and predict contamination risks. Sanitization Sanitization is a crucial step in maintaining hygiene in commercial spaces. By integrating automated IoT alerts, businesses can ensure timely sanitation, reducing contamination risks and improving customer experience. Implementing IoT-Based Sanitization Alerts const threshold = 70; // Humidity threshold for sanitization function checkHumidity(humidity) { if (humidity > threshold) { console.log("Triggering sanitization process..."); // Send alert to cleaning staff } } This JavaScript function triggers an alert when humidity exceeds a certain level, indicating the need for sanitización. Disinfection Disinfection goes beyond regular cleaning by eliminating harmful bacteria and viruses. IoT-powered UV-C sterilization can be scheduled automatically to enhance cleanliness levels. Integrating UV-C Disinfection with IoT import RPi.GPIO as GPIO import time uvc_pin = 18 # GPIO pin for UV-C light GPIO.setmode(GPIO.BCM) GPIO.setup(uvc_pin, GPIO.OUT) # Turn on UV-C light for disinfection GPIO.output(uvc_pin, GPIO.HIGH) time.sleep(30) # Run for 30 seconds GPIO.output(uvc_pin, GPIO.LOW) GPIO.cleanup() This script activates a UV-C light for desinfección, ensuring an automated and effective sterilization process. Maintenance Maintenance is key to keeping IoT devices operational. Regular sensor calibration and system updates improve accuracy and longevity. Automated Maintenance Notifications function scheduleMaintenance() { let now = new Date(); let nextMaintenance = new Date(); nextMaintenance.setDate(now.getDate() + 30); console.log(`Next maintenance scheduled on: ${nextMaintenance.toDateString()}`); } scheduleMaintenance(); This function schedules routine mantenimiento, ensuring that IoT devices operate efficiently. Dusting Dusting ensures that sensors and devices remain free from dust, which can impact their accuracy. IoT-based air filters and vacuum robots can be programmed to handle this task automatically. Smart Vacuum Integration class SmartVacuum: def __init__(self, room): self.room = room def start_cleaning(self): print(f"Starting dust removal in {self.room}...") vacuum = SmartVacuum("Office") vacuum.start_cleaning() This Python class represents an automated dust removal system that can be deployed in commercial spaces. Conclusion IoT-powered hygiene monitoring with SensorClean offers a game-changing solution for commercial spaces. By leveraging real-time data, automated alerts, and smart cleaning techniques, businesses can enhance hygiene levels efficiently. By implementing Sanitization, Disinfection, Maintenance, and Dusting, your commercial space can maintain optimal hygiene standards. Ready to take cleaning to the next level? Start building your IoT-powered hygiene monitoring system today!
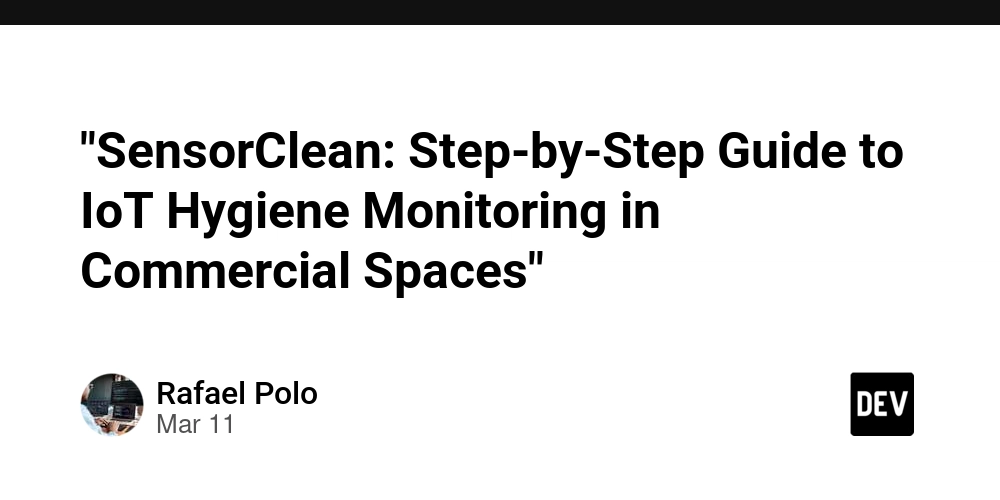
Introduction
Maintaining hygiene in commercial spaces has become more crucial than ever. Traditional cleaning methods often lack efficiency and real-time monitoring. Enter SensorClean, an innovative IoT-based solution designed to revolutionize commercial cleaning services. This system enables real-time tracking, automated alerts, and efficient hygiene management through smart sensors and cloud-based analytics.
In this blog post, we'll explore how to implement SensorClean step by step, with relevant code snippets and best practices to ensure a seamless deployment.
Why IoT for Cleaning Services?
IoT technology allows businesses to optimize their cleaning processes, reduce costs, and maintain compliance with hygiene standards. Some key benefits include:
- Real-time monitoring: Track cleanliness levels in different areas.
- Automated scheduling: Ensure cleaning tasks are performed consistently.
- Data-driven insights: Optimize resources based on usage trends.
Now, let's dive into building an IoT-powered hygiene monitoring system using SensorClean.
Step 1: Setting Up IoT Sensors
To get started, we'll use smart sensors that detect cleanliness levels through air quality, surface bacteria, and dust accumulation. These sensors can be integrated with a microcontroller such as Raspberry Pi or ESP8266.
Required Hardware
- Raspberry Pi / ESP8266
- Air quality sensor (MQ135)
- Optical dust sensor (GP2Y1010AU0F)
- Temperature and humidity sensor (DHT22)
- Wi-Fi module
Connecting the Sensors
import Adafruit_DHT
import time
dht_sensor = Adafruit_DHT.DHT22
sensor_pin = 4
while True:
humidity, temperature = Adafruit_DHT.read_retry(dht_sensor, sensor_pin)
if humidity and temperature:
print(f"Temp: {temperature:.2f}C, Humidity: {humidity:.2f}%")
time.sleep(5)
This Python script reads temperature and humidity data, which helps assess environmental conditions and predict contamination risks.
Sanitization
Sanitization is a crucial step in maintaining hygiene in commercial spaces. By integrating automated IoT alerts, businesses can ensure timely sanitation, reducing contamination risks and improving customer experience.
Implementing IoT-Based Sanitization Alerts
const threshold = 70; // Humidity threshold for sanitization
function checkHumidity(humidity) {
if (humidity > threshold) {
console.log("Triggering sanitization process...");
// Send alert to cleaning staff
}
}
This JavaScript function triggers an alert when humidity exceeds a certain level, indicating the need for sanitización.
Disinfection
Disinfection goes beyond regular cleaning by eliminating harmful bacteria and viruses. IoT-powered UV-C sterilization can be scheduled automatically to enhance cleanliness levels.
Integrating UV-C Disinfection with IoT
import RPi.GPIO as GPIO
import time
uvc_pin = 18 # GPIO pin for UV-C light
GPIO.setmode(GPIO.BCM)
GPIO.setup(uvc_pin, GPIO.OUT)
# Turn on UV-C light for disinfection
GPIO.output(uvc_pin, GPIO.HIGH)
time.sleep(30) # Run for 30 seconds
GPIO.output(uvc_pin, GPIO.LOW)
GPIO.cleanup()
This script activates a UV-C light for desinfección, ensuring an automated and effective sterilization process.
Maintenance
Maintenance is key to keeping IoT devices operational. Regular sensor calibration and system updates improve accuracy and longevity.
Automated Maintenance Notifications
function scheduleMaintenance() {
let now = new Date();
let nextMaintenance = new Date();
nextMaintenance.setDate(now.getDate() + 30);
console.log(`Next maintenance scheduled on: ${nextMaintenance.toDateString()}`);
}
scheduleMaintenance();
This function schedules routine mantenimiento, ensuring that IoT devices operate efficiently.
Dusting
Dusting ensures that sensors and devices remain free from dust, which can impact their accuracy. IoT-based air filters and vacuum robots can be programmed to handle this task automatically.
Smart Vacuum Integration
class SmartVacuum:
def __init__(self, room):
self.room = room
def start_cleaning(self):
print(f"Starting dust removal in {self.room}...")
vacuum = SmartVacuum("Office")
vacuum.start_cleaning()
This Python class represents an automated dust removal system that can be deployed in commercial spaces.
Conclusion
IoT-powered hygiene monitoring with SensorClean offers a game-changing solution for commercial spaces. By leveraging real-time data, automated alerts, and smart cleaning techniques, businesses can enhance hygiene levels efficiently.
By implementing Sanitization, Disinfection, Maintenance, and Dusting, your commercial space can maintain optimal hygiene standards. Ready to take cleaning to the next level? Start building your IoT-powered hygiene monitoring system today!