Route Declaration Order: Laravel's Hidden Routing Rule
Ever wondered why your custom Laravel routes suddenly disappeared? Trust me, you're not alone. I've spent countless hours debugging this exact issue – and now I'm sharing the solution that will save you major headaches. The Hidden Laravel Routing Rule That's Breaking Your Code Laravel's routing system is elegant and powerful, but there's one crucial detail that isn't immediately obvious in the documentation. When you're working with resource controllers alongside custom routes for the same resource, the order of declaration matters significantly. Let me break this down for you: // This works correctly ✅ Route::get('posts/featured', 'PostController@featured'); Route::resource('posts', 'PostController'); // This will fail ❌ Route::resource('posts', 'PostController'); Route::get('posts/featured', 'PostController@featured'); Why Does This Happen? When Laravel processes your routes, it registers them in the exact order they appear in your routes file. Resource routes are particularly special because they expand into multiple route definitions covering all the standard CRUD operations: GET /posts (index) GET /posts/create (create) POST /posts (store) GET /posts/{post} (show) GET /posts/{post}/edit (edit) PUT/PATCH /posts/{post} (update) DELETE /posts/{post} (destroy) The problem occurs with the show route - GET /posts/{post}. This route contains a wildcard parameter {post} that will match any segment after /posts/, including segments like "featured" that you might want to use for custom functionality. The Detailed Explanation Let's walk through exactly what happens in each scenario: Scenario 1: Custom Route Declared First (Correct Order) Route::get('posts/featured', 'PostController@featured'); Route::resource('posts', 'PostController'); Laravel registers the explicit route for posts/featured Then it registers all the resource routes When a request comes in for /posts/featured: Laravel checks registered routes in order It finds the explicit match first and routes to PostController@featured Scenario 2: Resource Route Declared First (Incorrect Order) Route::resource('posts', 'PostController'); Route::get('posts/featured', 'PostController@featured'); Laravel registers all the resource routes, including GET /posts/{post} Then it registers the explicit route for posts/featured When a request comes in for /posts/featured: Laravel checks registered routes in order It sees that /posts/{post} matches by treating "featured" as the {post} parameter It routes to PostController@show with "featured" as the post ID Your custom route never gets called!
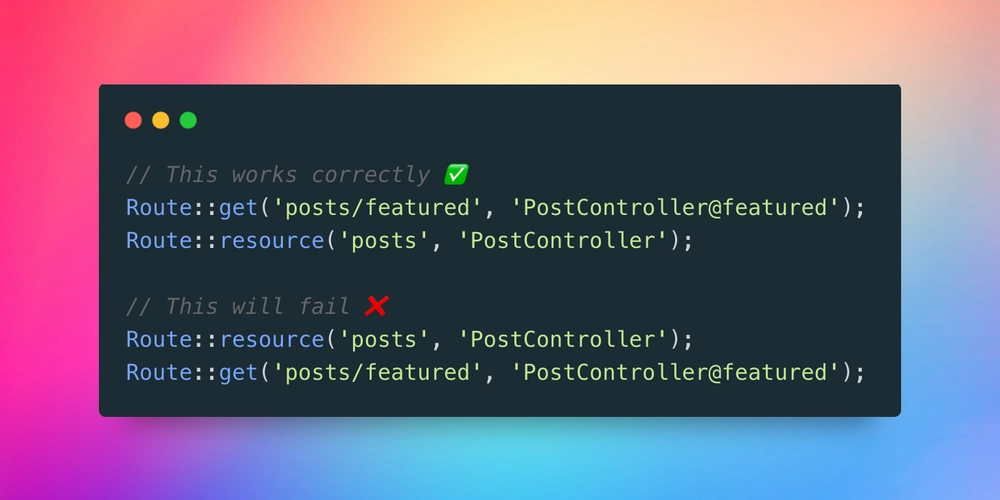
Ever wondered why your custom Laravel routes suddenly disappeared? Trust me, you're not alone. I've spent countless hours debugging this exact issue – and now I'm sharing the solution that will save you major headaches.
The Hidden Laravel Routing Rule That's Breaking Your Code
Laravel's routing system is elegant and powerful, but there's one crucial detail that isn't immediately obvious in the documentation. When you're working with resource controllers alongside custom routes for the same resource, the order of declaration matters significantly. Let me break this down for you:
// This works correctly ✅
Route::get('posts/featured', 'PostController@featured');
Route::resource('posts', 'PostController');
// This will fail ❌
Route::resource('posts', 'PostController');
Route::get('posts/featured', 'PostController@featured');
Why Does This Happen?
When Laravel processes your routes, it registers them in the exact order they appear in your routes file. Resource routes are particularly special because they expand into multiple route definitions covering all the standard CRUD operations:
GET /posts (index)
GET /posts/create (create)
POST /posts (store)
GET /posts/{post} (show)
GET /posts/{post}/edit (edit)
PUT/PATCH /posts/{post} (update)
DELETE /posts/{post} (destroy)
The problem occurs with the show
route - GET /posts/{post}
. This route contains a wildcard parameter {post}
that will match any segment after /posts/
, including segments like "featured" that you might want to use for custom functionality.
The Detailed Explanation
Let's walk through exactly what happens in each scenario:
Scenario 1: Custom Route Declared First (Correct Order)
Route::get('posts/featured', 'PostController@featured');
Route::resource('posts', 'PostController');
- Laravel registers the explicit route for
posts/featured
- Then it registers all the resource routes
- When a request comes in for
/posts/featured
:- Laravel checks registered routes in order
- It finds the explicit match first and routes to
PostController@featured
Scenario 2: Resource Route Declared First (Incorrect Order)
Route::resource('posts', 'PostController');
Route::get('posts/featured', 'PostController@featured');
- Laravel registers all the resource routes, including
GET /posts/{post}
- Then it registers the explicit route for
posts/featured
- When a request comes in for
/posts/featured
:- Laravel checks registered routes in order
- It sees that
/posts/{post}
matches by treating "featured" as the{post}
parameter - It routes to
PostController@show
with "featured" as the post ID - Your custom route never gets called!