React Server Components in Practice: Building a fake E-commerce Site with Next.js 15 latest features
See the demo here - See the source code here For the last 18 months, in the react ecosystem, we have been witnessing the rise of server components. It's not the only new concept that has been introduced. Metaframeworks like Next.js have been relying for months on alpha/beta/RC versions of react 19, preparing for features like: RSC (React Server Components) Server Actions Streaming New caching strategies Partial Prerendering The stable version of react 19 shipped in December 2024 (see their blog post). Table of Contents Experiments Features of the project Tech stack Implementation Experimental flags Server components Layout QR Code Generation Header / UserIcon Header / SearchCombobox Category page Search Page / Product Page Mixing RSC and client components Server actions Progressive Enhancement Conclusion Experiments For the last year, multiple blog posts and videos have been published explaining how you would use those new features (some content even go deeper explaining some implementation details). Those are great content, however, they don't address the following questions: Should we now go all-in on RSCs and Server Actions? Drop all client components? How does this mix with client-side fetching? (react query, etc ...) When should you use either one of them? This is why I decided to build a real project to experiment on those new features. Features of the project See the demo here - See the source code here It's a fake e-commerce website, that allows you to: Access list of categories of products Access products by category Access product details Search for products Add/remove product to/from cart View cart Login/Logout (a fake identity is generated for you when you login) Checkout (a fake payment is made) Constraints: It should be SSR friendly (for performance and SEO reasons) It should also have a good user experience on client-side (fast navigation, interactivity, etc ...) It should take in account progressive enhancement Real api calls are made to an external API containing mock data Tech stack Next.js 15.2 - canary version (some features are not yet available in the stable version) React 19 Tailwind CSS TypeScript Shadcn UI Vercel (for hosting) No database, everything is stored on cookies for simplicity Implementation Experimental flags I turned on the following experimental flags (which needed the canary version of Next.js): dynamicIO The dynamicIO flag is an experimental feature in Next.js that causes data fetching operations in the App Router to be excluded from pre-renders unless they are explicitly cached. It is useful if your application requires fresh data fetching during runtime rather than serving from a pre-rendered cache. It is expected to be used in conjunction with use cache so that your data fetching happens at runtime by default unless you define specific parts of your application to be cached with use cache at the page, function, or component level. Link to documentation (dynamicIO) ppr Partial Prerendering (PPR) enables you to combine static and dynamic components together in the same route. Link to documentation (PPR) Here is the report of the build, you can see most of the routes are partially prerendered as static HTML with dynamic server-streamed content. ◐ Route (app) Size First Load JS ┌ ◐ / 214 B 195 kB ├ ○ /_not-found 140 B 120 kB ├ ◐ /account 671 B 196 kB ├ ○ /api/hello/world 140 B 120 kB ├ ƒ /api/og 353 B 121 kB ├ ◐ /category/[slug] 1.17 kB 196 kB ├ └ /category/[slug] ├ ◐ /checkout 207 B 195 kB ├ ◐ /login 214 B 195 kB ├ ◐ /product/[slug] 1.04 kB 196 kB ├ └ /product/[slug] └ ◐ /search 1.17 kB 196 kB + First Load JS shared by all 120 kB ├ chunks/520-047851854c706276.js 60.6 kB ├ chunks/f5e865f6-9abf91c0747daaa2.js 57.8 kB └ other shared chunks (total) 1.92 kB ƒ Middleware 33.1 kB ○ (Static) prerendered as static content ◐ (Partial Prerender) prerendered as static HTML with dynamic server-streamed content ƒ (Dynamic) server-rendered on demand Server components By default, all components are server components in Next.js App Router, unless you explicitly mark them as client components using the "use client" directive. In RSCs, you can: Fetch data, access backend resources directly (APIS, databases, file-system, etc ...) Keep large dependencies and sensitive data on the server Send only the HTML output to the client RSCs can be rendered on the server, they
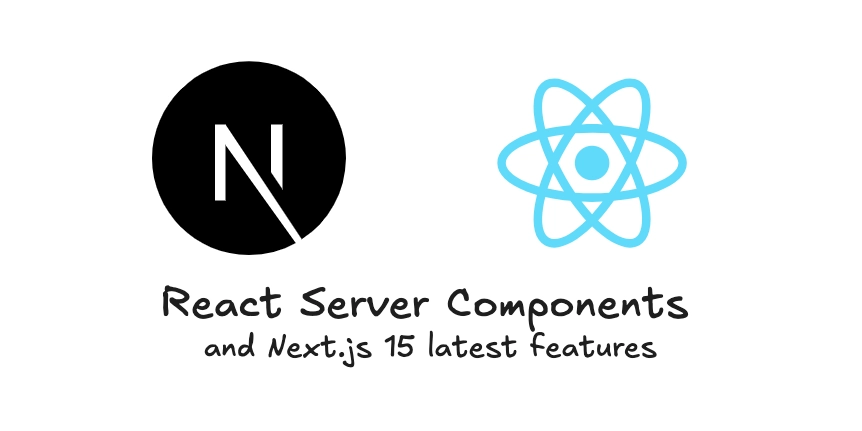
See the demo here - See the source code here
For the last 18 months, in the react ecosystem, we have been witnessing the rise of server components.
It's not the only new concept that has been introduced. Metaframeworks like Next.js have been relying for months on alpha/beta/RC versions of react 19, preparing for features like:
- RSC (React Server Components)
- Server Actions
- Streaming
- New caching strategies
- Partial Prerendering
The stable version of react 19 shipped in December 2024 (see their blog post).
Table of Contents
- Experiments
- Features of the project
- Tech stack
-
Implementation
- Experimental flags
-
Server components
- Layout
- QR Code Generation
- Header / UserIcon
- Header / SearchCombobox
- Category page
- Search Page / Product Page
- Mixing RSC and client components
- Server actions
- Progressive Enhancement
- Conclusion
Experiments
For the last year, multiple blog posts and videos have been published explaining how you would use those new features (some content even go deeper explaining some implementation details).
Those are great content, however, they don't address the following questions:
- Should we now go all-in on RSCs and Server Actions? Drop all client components?
- How does this mix with client-side fetching? (react query, etc ...)
- When should you use either one of them?
This is why I decided to build a real project to experiment on those new features.
Features of the project
See the demo here - See the source code here
It's a fake e-commerce website, that allows you to:
- Access list of categories of products
- Access products by category
- Access product details
- Search for products
- Add/remove product to/from cart
- View cart
- Login/Logout (a fake identity is generated for you when you login)
- Checkout (a fake payment is made)
Constraints:
- It should be SSR friendly (for performance and SEO reasons)
- It should also have a good user experience on client-side (fast navigation, interactivity, etc ...)
- It should take in account progressive enhancement
- Real api calls are made to an external API containing mock data
Tech stack
- Next.js 15.2 - canary version (some features are not yet available in the stable version)
- React 19
- Tailwind CSS
- TypeScript
- Shadcn UI
- Vercel (for hosting)
- No database, everything is stored on cookies for simplicity
Implementation
Experimental flags
I turned on the following experimental flags (which needed the canary version of Next.js):
dynamicIO
The
dynamicIO
flag is an experimental feature in Next.js that causes data fetching operations in the App Router to be excluded from pre-renders unless they are explicitly cached.It is useful if your application requires fresh data fetching during runtime rather than serving from a pre-rendered cache.
It is expected to be used in conjunction with
use cache
so that your data fetching happens at runtime by default unless you define specific parts of your application to be cached withuse cache
at the page, function, or component level.
Link to documentation (dynamicIO)
ppr
Partial Prerendering (PPR) enables you to combine static and dynamic components together in the same route.
Here is the report of the build, you can see most of the routes are partially prerendered as static HTML with dynamic server-streamed content. ◐
Route (app) Size First Load JS
┌ ◐ / 214 B 195 kB
├ ○ /_not-found 140 B 120 kB
├ ◐ /account 671 B 196 kB
├ ○ /api/hello/world 140 B 120 kB
├ ƒ /api/og 353 B 121 kB
├ ◐ /category/[slug] 1.17 kB 196 kB
├ └ /category/[slug]
├ ◐ /checkout 207 B 195 kB
├ ◐ /login 214 B 195 kB
├ ◐ /product/[slug] 1.04 kB 196 kB
├ └ /product/[slug]
└ ◐ /search 1.17 kB 196 kB
+ First Load JS shared by all 120 kB
├ chunks/520-047851854c706276.js 60.6 kB
├ chunks/f5e865f6-9abf91c0747daaa2.js 57.8 kB
└ other shared chunks (total) 1.92 kB
ƒ Middleware 33.1 kB
○ (Static) prerendered as static content
◐ (Partial Prerender) prerendered as static HTML with dynamic server-streamed content
ƒ (Dynamic) server-rendered on demand
Server components
By default, all components are server components in Next.js App Router, unless you explicitly mark them as client components using the "use client"
directive.
In RSCs, you can:
- Fetch data, access backend resources directly (APIS, databases, file-system, etc ...)
- Keep large dependencies and sensitive data on the server
- Send only the HTML output to the client
RSCs can be rendered on the server, they can also be rendered at build time - any runtime which doesn't have interactive content.
I will detail a few use cases I implemented on this project which leverages RSC features with the experimental flags I enabled.
The server actions part is detailed in the next section.
Layout - RSC + PPR = build time generation of static layout
A simplified version of the layout component:
<Providers>
<Header />
<Cart />
<main>
{children}
main>
<Footer />
Providers>
Since every components are server components by default, it will return a static HTML shell.
Since we enabled the ppr
flag, the layout will be rendered at build time, and the HTML will be cached (as it doesn't need to be rendered on each request).
You still can include client components, like the Cart
component, which will be rendered on the client.
Unrelated: on this project, I use the Route groups feature to have a specific layout for the checkout page and a generic one for the rest of the app.
QRCode - RSC + PPR + "use cache" = build time generation of static content
On the home page, I display a QRCode linking to the website, which is rendered by this react component:
.
CustomQRCode
being a server component, it lets us use the toDataURL
from the qrcode
package to generate the QRCode image. Since RSCs can be async, we can await toDataURL
directly in the component.
That way, we don't need to ship the qrcode
package to the client bundle, the RSC will return the HTML containing the QRCode image (an img tag with a data url).
Even better: since the url we want our QRCode is static (it won't change based on the request), we can tag the CustomQRCode
with the "use cache"
directive which, when used on a project with ppr
(Partial Prerendering) enabled, will prerender the QRCode image at build time.
Header / UserIcon - RSC + Suspense + PPR + DynamicIO = streaming
In the Header
component, I use a UserIcon
server component, either:
- shows a white user icon if the user is not logged in
- shows a green user icon (which links to the account page) if the user is logged in
// Header.tsx - simplified version
import { Suspense } from "react";
import { User } from "lucide-react";
import UserIcon from "./UserIcon";
<header>
{/* Rest of the header */}
<Suspense fallback={<User />}>
<UserIcon />
Suspense>
header>
// UserIcon.tsx - simplified version
import { User } from "lucide-react"; // The user svg icon
import Link from "next/link";
import { getUserInfos } from "@/actions/session";
export async function UserIcon() {
// Fetches the user infos server-side
const userInfos = await getUserInfos();
return (
<Link href="/account">
<User className={userInfos ? "text-green-300" : ""} />
Link>
);
}
- At build time, the
User
component (which is the fallback for ourSuspense
boundary wrappingUserIcon
) is rendered and cached. Thanks to theppr
flag, it will be rendered as static HTML. - At runtime, when the server starts streaming the HTML, it first returns this fallback HTML as part as the HTML response.
- While the HTML is being streamed to the client, the
UserIcon
server component is rendered on the server. - If the user is logged in, a fragment of HTML containing the green user icon is streamed to the client which will replace the fallback HTML.
All that in the same HTTP response. And since our "loading" state is just the default User
icon, it doesn't show at all.
Here is a simplified version of the raw HTML response with comments to explain the streaming part, follow the