React JS Classes: The Essential Guide for Today’s Front‑End Developers
Fusion Software Institute invites you to dive into the world of React JS class components — a technology every modern front‑end engineer should still keep in their toolbox, even in the era of Hooks and Server Components. Read More: React JS Classes: The Essential Guide for Today’s Front‑End Developers What Are React JS Class Components? A class component is an ES6 class that extends React.Component (or PureComponent). It introduces: Local state with this.state Lifecycle methods (componentDidMount, shouldComponentUpdate, etc.) Error boundaries via componentDidCatch A familiar OOP paradigm for developers coming from Java, C#, or C++ class Greeting extends React.Component { state = { timeOfDay: 'morning' }; componentDidMount() { const hour = new Date().getHours(); this.setState({ timeOfDay: hour < 12 ? 'morning' : 'evening' }); } render() { return Good {this.state.timeOfDay}, {this.props.name}!; } } Why Class Components Still Matter in 2025 Reason Real‑World Impact Legacy Codebases Thousands of production apps rely on classes; maintenance demands fluency. Explicit Lifecycles Fine‑grained control over mount, update, and unmount phases helps diagnose performance issues. Design Patterns Higher‑Order Components (HOC) and render props were born in the class era and remain widely used. TypeScript Strength Generics for props and state (class MyComp ) provide robust type safety at scale. Lifecycle Methods Demystified Phase Method Common Use Cases Mount constructor Initialize state; bind handlers componentDidMount Fetch data; set up subscriptions Update shouldComponentUpdate Prevent unnecessary re‑renders componentDidUpdate Act on prop/state changes Unmount componentWillUnmount Clean up timers, sockets, observers Error componentDidCatch Render fallback UI; log errors to monitoring Classes vs. Hooks: Choosing the Right Tool Feature Class Components Functional Components (Hooks) Learning Curve Intuitive for OOP developers Functional mindset required Granular Lifecycle Individual methods per phase Unified but potentially verbose useEffect Performance Tuning shouldComponentUpdate, PureComponent React.memo, custom comparison Library Compatibility Works out‑of‑the‑box with older React libraries Many libraries are Hook‑first Takeaway: Master both paradigms — use Hooks for green‑field features, but keep classes handy for legacy integration or precise lifecycle control. Best Practices for Class Components Leverage PureComponent for automatic shallow prop/state comparison. Compose, Don’t Inherit — wrap components instead of extending them. Document Side‑Effects clearly to prevent memory leaks and race conditions. Gradually Introduce Hooks — convert small, stateless classes first to modernize without big‑bang rewrites. Migration Blueprint: From Classes to Hooks Audit the Codebase — identify low‑complexity class components. Automate Refactors using codemods like react‑codemod. Replace State with useState, then move side‑effects to useEffect. Extract Shared Logic into custom hooks for reuse. Keep Complex Giants for Last — only refactor when parity exists. Fusion Software Institute believes that mastering both React JS classes and Hooks makes you a full‑spectrum front‑end developer, equipped to handle legacy maintenance and cutting‑edge innovation alike. Visit Here: https://www.fusion-institute.com/courses/react-js Contact Us Ready to level‑up your React skills? Reach out today: Phone: 9890647273, 7498992609 Email: enquiry@fusion-institute.com Website: www.fusionsoftwareinstitute.com Address: Office 101, 104 1st Floor, Stellar Spaces, Kharadi South Main Road, opp. Zensar, IT Park, Kharadi, Pune, Maharashtra 411014 Join Fusion Software Institute for hands‑on courses, expert mentorship, and career‑focused learning paths that keep you ahead of the curve.
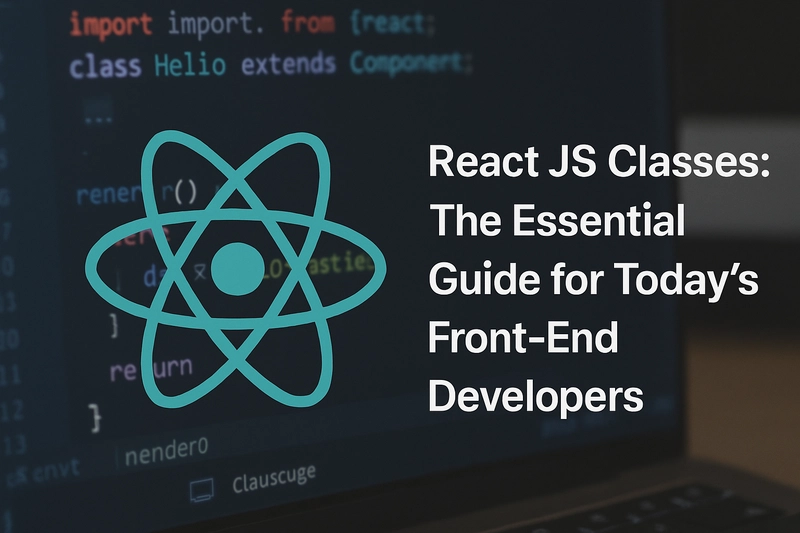
Fusion Software Institute invites you to dive into the world of React JS class components — a technology every modern front‑end engineer should still keep in their toolbox, even in the era of Hooks and Server Components.
Read More: React JS Classes: The Essential Guide for Today’s Front‑End Developers
- What Are React JS Class Components? A class component is an ES6 class that extends React.Component (or PureComponent). It introduces:
Local state with this.state
Lifecycle methods (componentDidMount, shouldComponentUpdate, etc.)
Error boundaries via componentDidCatch
A familiar OOP paradigm for developers coming from Java, C#, or C++
class Greeting extends React.Component {
state = { timeOfDay: 'morning' };
componentDidMount() {
const hour = new Date().getHours();
this.setState({ timeOfDay: hour < 12 ? 'morning' : 'evening' });
}
render() {
return
Good {this.state.timeOfDay}, {this.props.name}!
;}
}
-
Why Class Components Still Matter in 2025
Reason Real‑World Impact Legacy Codebases Thousands of production apps rely on classes; maintenance demands fluency. Explicit Lifecycles Fine‑grained control over mount, update, and unmount phases helps diagnose performance issues. Design Patterns Higher‑Order Components (HOC) and render props were born in the class era and remain widely used. TypeScript Strength Generics for props and state (class MyComp) provide robust type safety at scale.
Lifecycle Methods Demystified
Phase Method Common Use Cases Mount constructor Initialize state; bind handlers componentDidMount Fetch data; set up subscriptions Update shouldComponentUpdate Prevent unnecessary re‑renders componentDidUpdate Act on prop/state changes Unmount componentWillUnmount Clean up timers, sockets, observers Error componentDidCatch Render fallback UI; log errors to monitoringClasses vs. Hooks: Choosing the Right Tool
Feature Class Components Functional Components (Hooks) Learning Curve Intuitive for OOP developers Functional mindset required Granular Lifecycle Individual methods per phase Unified but potentially verbose useEffect Performance Tuning shouldComponentUpdate, PureComponent React.memo, custom comparison Library Compatibility Works out‑of‑the‑box with older React libraries Many libraries are Hook‑first
Takeaway: Master both paradigms — use Hooks for green‑field features, but keep classes handy for legacy integration or precise lifecycle control.
- Best Practices for Class Components Leverage PureComponent for automatic shallow prop/state comparison. Compose, Don’t Inherit — wrap components instead of extending them. Document Side‑Effects clearly to prevent memory leaks and race conditions. Gradually Introduce Hooks — convert small, stateless classes first to modernize without big‑bang rewrites.
- Migration Blueprint: From Classes to Hooks Audit the Codebase — identify low‑complexity class components. Automate Refactors using codemods like react‑codemod. Replace State with useState, then move side‑effects to useEffect. Extract Shared Logic into custom hooks for reuse. Keep Complex Giants for Last — only refactor when parity exists. Fusion Software Institute believes that mastering both React JS classes and Hooks makes you a full‑spectrum front‑end developer, equipped to handle legacy maintenance and cutting‑edge innovation alike.
Visit Here: https://www.fusion-institute.com/courses/react-js
Contact Us
Ready to level‑up your React skills? Reach out today:
Phone: 9890647273, 7498992609
Email: enquiry@fusion-institute.com
Website: www.fusionsoftwareinstitute.com
Address: Office 101, 104 1st Floor, Stellar Spaces, Kharadi South Main Road, opp. Zensar, IT Park, Kharadi, Pune, Maharashtra 411014
Join Fusion Software Institute for hands‑on courses, expert mentorship, and career‑focused learning paths that keep you ahead of the curve.