Random Quotes Generator in spring Boot
Quotes Generator 1.controller package com.example.demo.controller; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.GetMapping; import com.example.demo.service.quoteservice; @Controller public class QuotesController { @Autowired private quoteservice quoteService; @GetMapping("/") public String show_Quotes_Page(Model model) { String quote = quoteService.send_quote(); model.addAttribute("quo", quote); return "quotes"; } } 2.Service package com.example.demo.service; import java.util.List; import java.util.Random; import org.springframework.stereotype.Service; @Service public class quoteservice { public String send_quote() { List quotesList = List.of ("Failure is Stepping Stone of Success", "Hardwork never fails", "Love is Life", "I ride my soul", "Talent never fails"); Random random = new Random(); int random_no = random.nextInt(quotesList.size()); return quotesList.get(random_no); } } 3.html.file Random Quote Generator Random Quote Generator Next Quote
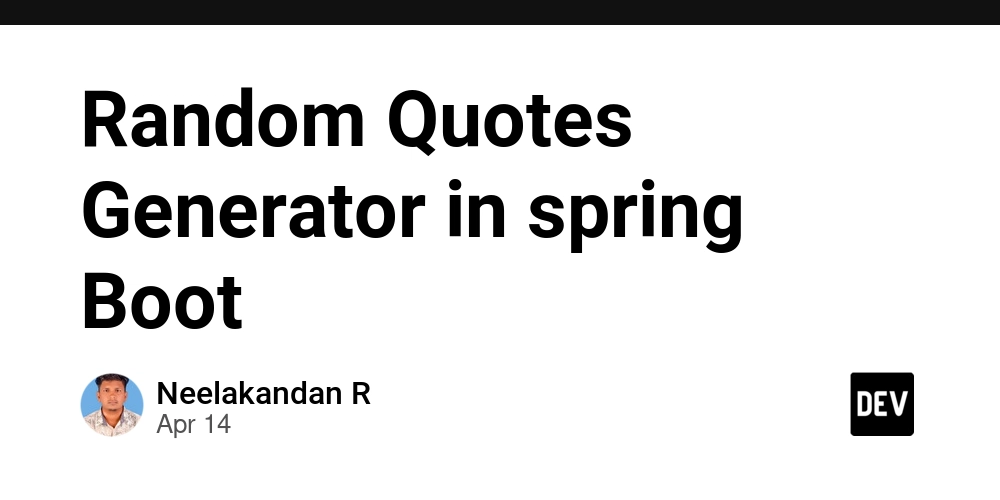
Quotes Generator
1.controller
package com.example.demo.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import com.example.demo.service.quoteservice;
@Controller
public class QuotesController
{
@Autowired
private quoteservice quoteService;
@GetMapping("/")
public String show_Quotes_Page(Model model)
{
String quote = quoteService.send_quote();
model.addAttribute("quo", quote);
return "quotes";
}
}
2.Service
package com.example.demo.service;
import java.util.List;
import java.util.Random;
import org.springframework.stereotype.Service;
@Service
public class quoteservice
{
public String send_quote()
{
List quotesList = List.of
("Failure is Stepping Stone of Success",
"Hardwork never fails",
"Love is Life",
"I ride my soul",
"Talent never fails");
Random random = new Random();
int random_no = random.nextInt(quotesList.size());
return quotesList.get(random_no);
}
}
3.html.file
Random Quote Generator