Quick & Easy Intro to Data Structures & Algorithms for Beginners
In programming, data structures and algorithms (DSA) are the foundational building blocks that dictate how software applications handle data and perform tasks efficiently. Understanding these concepts is crucial for any software developer, as they directly impact programs' performance, scalability, and efficiency. Definition of Data Structures A data structure is a specialized way to organize, manage, and store data for efficient access and modification. Different data structures are designed to suit specific types of operations, enabling developers to store and manipulate data effectively. What is an Algorithm? An algorithm is a set of well-defined instructions for solving a particular problem or performing a specific task. Algorithms take data as input and produce an output, often through a series of logical steps. The efficiency of an algorithm is measured in terms of its time complexity (how fast it runs) and space complexity (how much memory it uses). Real-Life Example of Data Structures and Algorithms Imagine you are organizing a bookshelf. How you arrange your books and the method you use to find a particular book are akin to data structures and algorithms. Data Structure: The bookshelf is a data structure. If you arrange books alphabetically by author, you are using an array-like structure where each position corresponds to a specific book. Algorithm: When you want to find a specific book, you might start from one end and check each book one by one until you find the one you need. Basic Data Structures Here are some of the most commonly used data structures: Arrays: A collection of elements, identified by index or key. Arrays are fixed in size and allow constant access to elements. Linked Lists: A sequence of nodes where each node points to the next. Linked lists allow for dynamic memory allocation and efficient insertions and deletions. Stacks: A linear data structure that follows the Last-In-First-Out (LIFO) principle. It's used in scenarios like undo functionality in text editors. Queues: A linear structure that follows the First-In-First-Out (FIFO) principle, often used in task scheduling and handling requests. Trees: A hierarchical structure with a root node and child nodes, commonly used for storing hierarchical data like file systems or databases (e.g., Binary Trees, AVL Trees). Graphs: A collection of nodes (vertices) connected by edges, used to represent networks, such as social media connections or city maps. Hash Tables: A data structure that maps keys to values, providing fast access to elements based on their keys. Basic Algorithms Some of the most commonly used algorithms include: Sorting Algorithms: Methods to arrange data in a particular order. Examples include QuickSort, MergeSort, and BubbleSort. Searching Algorithms: Techniques to find an element within a data structure. Examples include Binary Search and Linear Search. Traversal Algorithms: Ways to navigate through data structures, especially trees and graphs. Examples include Depth-First Search (DFS) and Breadth-First Search (BFS). Dynamic Programming: A method to solve complex problems by breaking them down into simpler subproblems. This technique is often used in optimization problems. Greedy Algorithms: Algorithms that make locally optimal choices at each step to find a global optimum, are often used in tasks like scheduling and resource allocation. Divide and Conquer: A technique that divides a problem into smaller subproblems, solves each one independently, and combines their results. QuickSort and MergeSort are classic examples. Importance of Data Structures and Algorithms Mastering data structures and algorithms is essential for several reasons: Efficiency: Well-designed data structures and algorithms improve the efficiency of software by reducing time and space complexity. This is particularly important for applications handling large amounts of data or requiring fast response times. Problem Solving: Many real-world problems can be modeled and solved using data structures and algorithms. Understanding these concepts allows developers to design solutions that are both correct and efficient. Scalability: Efficient algorithms ensure that programs can handle growth in data size or user base without significant degradation in performance. Competitive Advantage: In coding interviews, knowledge of DSA is often tested as it reflects a candidate's ability to think logically and solve complex problems. Conclusion Understanding data structures and algorithms is crucial for writing efficient code and solving complex problems. From everyday tasks like organizing a bookshelf to building scalable software systems, DSA plays a vital role in how data is managed and processed. Whether you're sorting a list, searching for a particular item, or managing complex hierarchical data, the right choice of data structure and algorith
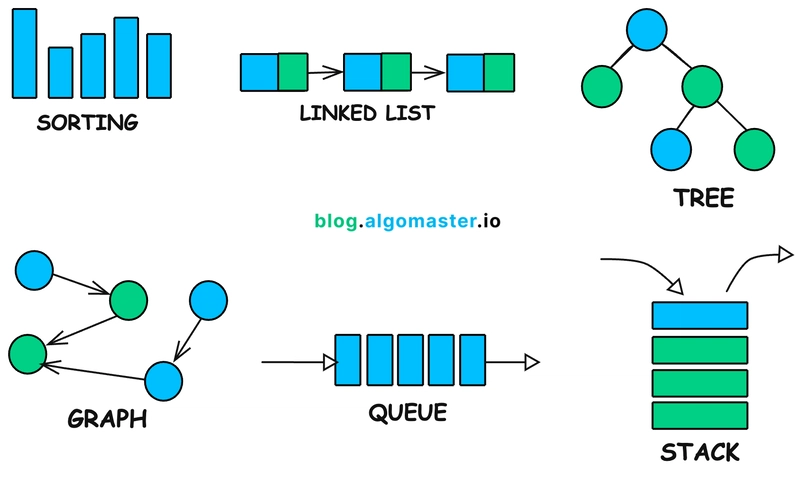
In programming, data structures and algorithms (DSA) are the foundational building blocks that dictate how software applications handle data and perform tasks efficiently. Understanding these concepts is crucial for any software developer, as they directly impact programs' performance, scalability, and efficiency.
Definition of Data Structures
A data structure is a specialized way to organize, manage, and store data for efficient access and modification. Different data structures are designed to suit specific types of operations, enabling developers to store and manipulate data effectively.
What is an Algorithm?
An algorithm is a set of well-defined instructions for solving a particular problem or performing a specific task. Algorithms take data as input and produce an output, often through a series of logical steps. The efficiency of an algorithm is measured in terms of its time complexity (how fast it runs) and space complexity (how much memory it uses).
Real-Life Example of Data Structures and Algorithms
Imagine you are organizing a bookshelf. How you arrange your books and the method you use to find a particular book are akin to data structures and algorithms.
- Data Structure: The bookshelf is a data structure. If you arrange books alphabetically by author, you are using an array-like structure where each position corresponds to a specific book.
- Algorithm: When you want to find a specific book, you might start from one end and check each book one by one until you find the one you need.
Basic Data Structures
Here are some of the most commonly used data structures:
Arrays: A collection of elements, identified by index or key. Arrays are fixed in size and allow constant access to elements.
Linked Lists: A sequence of nodes where each node points to the next. Linked lists allow for dynamic memory allocation and efficient insertions and deletions.
Stacks: A linear data structure that follows the Last-In-First-Out (LIFO) principle. It's used in scenarios like undo functionality in text editors.
Queues: A linear structure that follows the First-In-First-Out (FIFO) principle, often used in task scheduling and handling requests.
Trees: A hierarchical structure with a root node and child nodes, commonly used for storing hierarchical data like file systems or databases (e.g., Binary Trees, AVL Trees).
Graphs: A collection of nodes (vertices) connected by edges, used to represent networks, such as social media connections or city maps.
Hash Tables: A data structure that maps keys to values, providing fast access to elements based on their keys.
Basic Algorithms
Some of the most commonly used algorithms include:
Sorting Algorithms: Methods to arrange data in a particular order. Examples include QuickSort, MergeSort, and BubbleSort.
Searching Algorithms: Techniques to find an element within a data structure. Examples include Binary Search and Linear Search.
Traversal Algorithms: Ways to navigate through data structures, especially trees and graphs. Examples include Depth-First Search (DFS) and Breadth-First Search (BFS).
Dynamic Programming: A method to solve complex problems by breaking them down into simpler subproblems. This technique is often used in optimization problems.
Greedy Algorithms: Algorithms that make locally optimal choices at each step to find a global optimum, are often used in tasks like scheduling and resource allocation.
Divide and Conquer: A technique that divides a problem into smaller subproblems, solves each one independently, and combines their results. QuickSort and MergeSort are classic examples.
Importance of Data Structures and Algorithms
Mastering data structures and algorithms is essential for several reasons:
- Efficiency: Well-designed data structures and algorithms improve the efficiency of software by reducing time and space complexity. This is particularly important for applications handling large amounts of data or requiring fast response times.
- Problem Solving: Many real-world problems can be modeled and solved using data structures and algorithms. Understanding these concepts allows developers to design solutions that are both correct and efficient.
- Scalability: Efficient algorithms ensure that programs can handle growth in data size or user base without significant degradation in performance.
- Competitive Advantage: In coding interviews, knowledge of DSA is often tested as it reflects a candidate's ability to think logically and solve complex problems.
Conclusion
Understanding data structures and algorithms is crucial for writing efficient code and solving complex problems. From everyday tasks like organizing a bookshelf to building scalable software systems, DSA plays a vital role in how data is managed and processed. Whether you're sorting a list, searching for a particular item, or managing complex hierarchical data, the right choice of data structure and algorithm can significantly impact the performance and scalability of your solution.
By mastering these concepts, developers can design systems that are not only correct but also optimized for efficiency, making DSA a cornerstone of computer science education and practice.
Additional:
This blog post has been transformed into a video on our AlgoSync YouTube channel.
Link: Video