Python: Types of Data
Types of data Numeric Integer Float Sequence Complex number Dictionary string list tuples Boolean Set Integers: Integers, as you know in mathematics are numbers without any fraction part. They can be 0, positive or negative. x = 42 print(type(x)) print(x - 10) Floating Point number: Floating point numbers (float) are real numbers with floating point representation, they are specified by a decimal point. y = 5.75 print(type(y)) print(y + 2.25) Complex numbers: Complex numbers in python are specified as (real part) + (imaginary part)j. They are represented by the complex class. z = 3+4j print(type(z)) print(z.real) print(z.imag) NOTE: In Python, the type() function is used to determine the data type of a value or variable. String: A string can be defined as a sequence of characters enclosed in single, double, or triple quotation marks text = "Python" print("Type:", type(text)) print("Value:", text.lower()) Lists: Lists are an ordered sequence of one or more types of values. They are mulable, their items can be modified. They are created by enclosing items (separated by commas) inside square brackets []. colors = ["red", "green", "blue"] print("Type:", type(colors) print("Value:", colors[2]) Tuples: Like lists, Tuples are also an ordered sequence of one or more types of values except for they are immutable, their values can't be modified. dimensions = (1920, 1080) print("Type:", type(dimensions)) print("Value:", dimensipns[]) Dictionaries: In Python, Dictionaries are an unordered collection of pair values (key: value). Dictionaries are of the type dict. The elements of a dictionary are enclosed in curly brackets {}. student = ("name": "liam", "score": 88) print("Type:", type(student)) print("Value:", student["score"]) Booleans: Boolean is a data type that has one of two possible values, True or False. They are mostly used in creating the control flow of a program using conditional statements. is_valid = False print("Type:", type(is_valid)) print("Value:", is_valid == True) Sets: Sets in python are an unordered collection of elements. They contain only unique elements. They are mutable, their values can be modified. They are created by elements separated by commas enclosed in curly brackets {}. numbers = {1, 2, 2, 3, 4} print("Type:", type(numbers)) print("Value:", numbers)
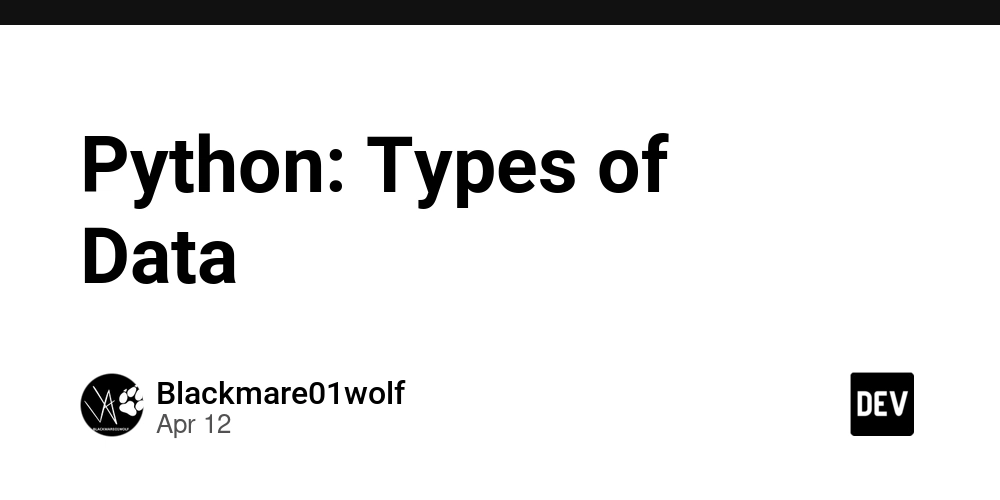
Types of data
- Numeric
- Integer
- Float
- Sequence
- Complex number
- Dictionary
- string
- list
- tuples
Boolean
Set
Integers:
Integers, as you know in mathematics are numbers without any fraction part. They can be 0, positive or negative.
x = 42
print(type(x))
print(x - 10)
Floating Point number:
Floating point numbers (float) are real numbers with floating point representation, they are specified by a decimal point.
y = 5.75
print(type(y))
print(y + 2.25)
Complex numbers:
Complex numbers in python are specified as (real part) + (imaginary part)j. They are represented by the complex class.
z = 3+4j
print(type(z))
print(z.real)
print(z.imag)
NOTE: In Python, the type() function is used to determine the data type of a value or variable.
String:
A string can be defined as a sequence of characters enclosed in single, double, or triple quotation marks
text = "Python"
print("Type:", type(text))
print("Value:", text.lower())
Lists:
Lists are an ordered sequence of one or more types of values. They are mulable, their items can be modified. They are created by enclosing items (separated by commas) inside square brackets []
.
colors = ["red", "green", "blue"]
print("Type:", type(colors)
print("Value:", colors[2])
Tuples:
Like lists, Tuples are also an ordered sequence of one or more types of values except for they are immutable, their values can't be modified.
dimensions = (1920, 1080)
print("Type:", type(dimensions))
print("Value:", dimensipns[])
Dictionaries:
In Python, Dictionaries are an unordered collection of pair values (key: value)
. Dictionaries are of the type dict. The elements of a dictionary are enclosed in curly brackets {}
.
student = ("name": "liam", "score": 88)
print("Type:", type(student))
print("Value:", student["score"])
Booleans:
Boolean is a data type that has one of two possible values, True
or False
. They are mostly used in creating the control flow of a program using conditional statements.
is_valid = False
print("Type:", type(is_valid))
print("Value:", is_valid == True)
Sets:
Sets in python are an unordered collection of elements. They contain only unique elements. They are mutable, their values can be modified. They are created by elements separated by commas enclosed in curly brackets {}
.
numbers = {1, 2, 2, 3, 4}
print("Type:", type(numbers))
print("Value:", numbers)