Powers of 2
Instructions: Complete the function that takes a non-negative integer n as input, and returns a list of all the powers of 2 with the exponent ranging from 0 to n ( inclusive ). Examples n = 0 ==> [1] # [2^0] n = 1 ==> [1, 2] # [2^0, 2^1] n = 2 ==> [1, 2, 4] # [2^0, 2^1, 2^2] Thoughts: I do create an array inside the function with n length. const arr = new Array(n); 2.Using a for loop it gives me the ability to loop until n (including n in this case) and raise 2 to the power of i until i=n. 3.In every iteration of the loop, I add the el to the newArr. newArr.push(el); Solution: function powersOfTwo(n){ const arr = new Array(n); let newArr = []; for (let i = 0; i
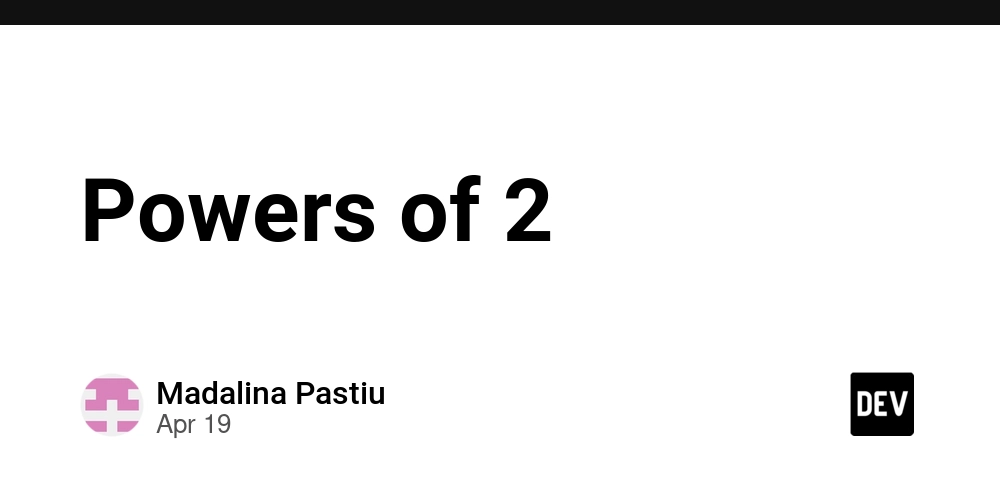
Instructions:
Complete the function that takes a non-negative integer n as input, and returns a list of all the powers of 2 with the exponent ranging from 0 to n ( inclusive ).
Examples
n = 0 ==> [1] # [2^0]
n = 1 ==> [1, 2] # [2^0, 2^1]
n = 2 ==> [1, 2, 4] # [2^0, 2^1, 2^2]
Thoughts:
- I do create an array inside the function with n length.
const arr = new Array(n);
2.Using a for loop it gives me the ability to loop until n (including n in this case) and raise 2 to the power of i until i=n. 3.In every iteration of the loop, I add the el to the newArr.newArr.push(el);
Solution:
function powersOfTwo(n){
const arr = new Array(n);
let newArr = [];
for (let i = 0; i <= n; i++) {
const el = Math.pow(2, i);
newArr.push(el);
}
return newArr;
}
This is a CodeWars Challenge of 8kyu Rank (https://www.codewars.com/kata/57a083a57cb1f31db7000028/train/javascript)