Performance Budgeting with JavaScript
Performance Budgeting with JavaScript: A Comprehensive Exploration Introduction to Performance Budgeting Performance budgeting is an essential practice in modern web development that helps teams define and manage performance targets for web applications. By setting strict performance budgets, developers, designers, and project managers can ensure that their applications adhere to certain performance metrics, such as load times, rendering speed, and resource consumption. Historically, the term "performance budget" emerged alongside the increasing complexity of web applications in the early 2010s, particularly with the rise of Single Page Applications (SPAs) and heavy reliance on APIs. As applications grew in size and complexity, the need to measure and manage performance became paramount, especially given the increasing expectations of users for fast and responsive experiences. This article delves into the nuanced concepts of performance budgeting in JavaScript, providing a comprehensive guide for senior developers. We will explore technical, historical, and practical dimensions, using code examples, industry use cases, and performance optimization strategies. The Technical Foundation of Performance Budgeting Defining the Performance Budget Performance budgeting typically revolves around establishing limits on various performance metrics, including: Page load time: How long it takes for the primary content to be visible to users. First Input Delay (FID): The time from when a user first interacts with your page to the time when the browser responds to that interaction. Time to Interactive (TTI): The interval it takes for the page to become fully interactive. Resource Size Budgets: Limits on the total size of CSS, JavaScript, image, and font files. Example Definition For example, a team might decide that the total JavaScript bundle size should not exceed 150 KB minified and gzipped. This definition would serve as a key component of the performance budget. Tools and Metrics Several tools and libraries can aid in tracking performance budgets: Lighthouse: An automated tool for improving the quality of web pages. WebPageTest: A tool that provides detailed insights into various performance metrics. BundleAnalyzer: A tool for visualizing the size of Webpack output files. Chrome DevTools: Built-in browser tools for analyzing performance. Implementing Performance Budgets in JavaScript To implement performance budgets effectively, the budgets should be incorporated into the development process. Below is an implementation example using a build tool such as Webpack, enforcing budget limits directly within the build configuration. // webpack.config.js const { BundleAnalyzerPlugin } = require('webpack-bundle-analyzer'); module.exports = { // Other configurations... plugins: [ new BundleAnalyzerPlugin({ analyzerMode: 'static', openAnalyzer: false, reportFilename: 'bundle-report.html', }), ], performance: { hints: 'warning', // or 'error' to fail the build maxAssetSize: 150 * 1024, // 150 KB maxEntrypointSize: 300 * 1024, // 300 KB as an example }, }; This configuration will issue a warning whenever the asset sizes exceed specified limits, directly enforcing performance budgets. Advanced Build Configurations Example: Multi-Page Applications For larger applications, such as complex multi-page applications, teams may want different budgets for different pages. In this case, separate performance budgets could be defined in a more structured way: // budget.config.js const performanceBudgets = { homepage: { maxAssetSize: 100 * 1024, maxEntrypointSize: 150 * 1024, }, productPage: { maxAssetSize: 200 * 1024, maxEntrypointSize: 250 * 1024, }, }; // webpack.config.js const performance = performanceBudgets[process.env.NODE_ENV === 'production' ? 'homepage' : 'productPage']; module.exports = { performance: { hints: 'error', maxAssetSize: performance.maxAssetSize, maxEntrypointSize: performance.maxEntrypointSize, }, }; Code Splitting and Dynamic Imports An advanced technique for optimizing performance and staying within budget is to utilize code splitting. Code splitting allows you to create separate bundles for different parts of your application, ensuring that only the necessary code is loaded for each page. This can be efficiently implemented using dynamic imports. // Example of dynamic import in JavaScript const loadComponent = async () => { const component = await import('./MyComponent.js'); document.body.appendChild(component.default()); }; This implementation ensures that MyComponent is loaded only when required, reducing the initial bundle size. Advanced Edge Cases in Performance
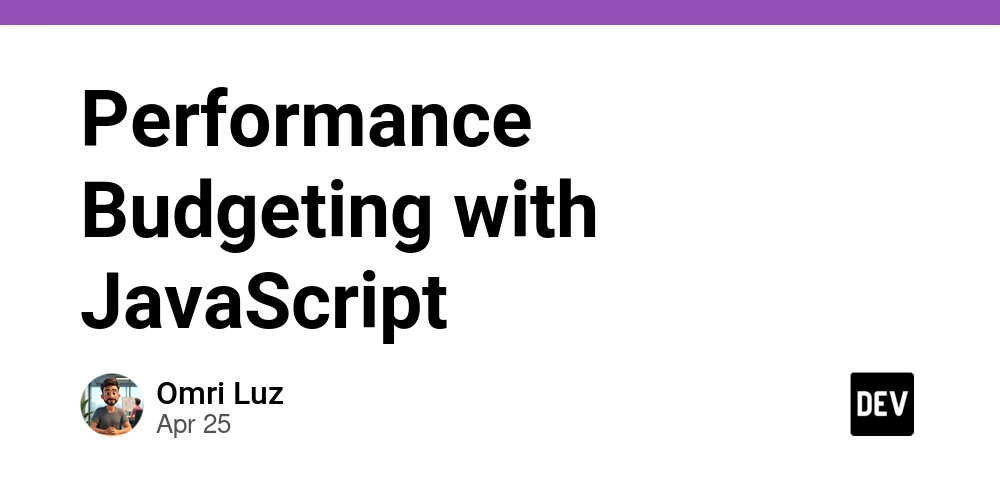
Performance Budgeting with JavaScript: A Comprehensive Exploration
Introduction to Performance Budgeting
Performance budgeting is an essential practice in modern web development that helps teams define and manage performance targets for web applications. By setting strict performance budgets, developers, designers, and project managers can ensure that their applications adhere to certain performance metrics, such as load times, rendering speed, and resource consumption.
Historically, the term "performance budget" emerged alongside the increasing complexity of web applications in the early 2010s, particularly with the rise of Single Page Applications (SPAs) and heavy reliance on APIs. As applications grew in size and complexity, the need to measure and manage performance became paramount, especially given the increasing expectations of users for fast and responsive experiences.
This article delves into the nuanced concepts of performance budgeting in JavaScript, providing a comprehensive guide for senior developers. We will explore technical, historical, and practical dimensions, using code examples, industry use cases, and performance optimization strategies.
The Technical Foundation of Performance Budgeting
Defining the Performance Budget
Performance budgeting typically revolves around establishing limits on various performance metrics, including:
- Page load time: How long it takes for the primary content to be visible to users.
- First Input Delay (FID): The time from when a user first interacts with your page to the time when the browser responds to that interaction.
- Time to Interactive (TTI): The interval it takes for the page to become fully interactive.
- Resource Size Budgets: Limits on the total size of CSS, JavaScript, image, and font files.
Example Definition
For example, a team might decide that the total JavaScript bundle size should not exceed 150 KB minified and gzipped. This definition would serve as a key component of the performance budget.
Tools and Metrics
Several tools and libraries can aid in tracking performance budgets:
- Lighthouse: An automated tool for improving the quality of web pages.
- WebPageTest: A tool that provides detailed insights into various performance metrics.
- BundleAnalyzer: A tool for visualizing the size of Webpack output files.
- Chrome DevTools: Built-in browser tools for analyzing performance.
Implementing Performance Budgets in JavaScript
To implement performance budgets effectively, the budgets should be incorporated into the development process. Below is an implementation example using a build tool such as Webpack, enforcing budget limits directly within the build configuration.
// webpack.config.js
const { BundleAnalyzerPlugin } = require('webpack-bundle-analyzer');
module.exports = {
// Other configurations...
plugins: [
new BundleAnalyzerPlugin({
analyzerMode: 'static',
openAnalyzer: false,
reportFilename: 'bundle-report.html',
}),
],
performance: {
hints: 'warning', // or 'error' to fail the build
maxAssetSize: 150 * 1024, // 150 KB
maxEntrypointSize: 300 * 1024, // 300 KB as an example
},
};
This configuration will issue a warning whenever the asset sizes exceed specified limits, directly enforcing performance budgets.
Advanced Build Configurations
Example: Multi-Page Applications
For larger applications, such as complex multi-page applications, teams may want different budgets for different pages. In this case, separate performance budgets could be defined in a more structured way:
// budget.config.js
const performanceBudgets = {
homepage: {
maxAssetSize: 100 * 1024,
maxEntrypointSize: 150 * 1024,
},
productPage: {
maxAssetSize: 200 * 1024,
maxEntrypointSize: 250 * 1024,
},
};
// webpack.config.js
const performance = performanceBudgets[process.env.NODE_ENV === 'production' ? 'homepage' : 'productPage'];
module.exports = {
performance: {
hints: 'error',
maxAssetSize: performance.maxAssetSize,
maxEntrypointSize: performance.maxEntrypointSize,
},
};
Code Splitting and Dynamic Imports
An advanced technique for optimizing performance and staying within budget is to utilize code splitting. Code splitting allows you to create separate bundles for different parts of your application, ensuring that only the necessary code is loaded for each page. This can be efficiently implemented using dynamic imports.
// Example of dynamic import in JavaScript
const loadComponent = async () => {
const component = await import('./MyComponent.js');
document.body.appendChild(component.default());
};
This implementation ensures that MyComponent
is loaded only when required, reducing the initial bundle size.
Advanced Edge Cases in Performance Budgeting
While performance budgeting can significantly enhance performance, there are edge cases to consider:
Third-party scripts: External scripts may unintentionally inflate your performance budget. For instance, social media widgets or ad scripts can add considerable load time and payload. Monitoring their size and impact is crucial.
Performance Variability: Local development environments may not reflect real-world performance. Developers should use tools that simulate various network conditions and device capabilities.
Selectors and DOM Access: Poorly constructed DOM access patterns (e.g., using
getElementById
vs.querySelectorAll
) could introduce performance bottlenecks even if a JavaScript bundle stays within size limits.
Example Code for Performance Monitoring
To regularly check the performance budget during development, consider the following script to log the bundle size:
import { performance } from 'perf_hooks';
const checkBundleSizes = (budget) => {
const sizes = getBundleSizes(); // Your method to obtain current bundle sizes
for (const [key, value] of Object.entries(sizes)) {
if (value > budget[key].maxSize) {
console.warn(`Warning: ${key} size exceeded the budget of ${budget[key].maxSize} bytes! Current size: ${value} bytes.`);
}
}
};
Performance Considerations
The significance of achieving performance targets cannot be understated, especially in the context of user experience and SEO. A slower site can lead to increased bounce rates and lower conversion rates. Here are various strategies to optimize JavaScript performance regarding budgets:
- Tree Shaking: Eliminate dead code with tree shaking during bundling.
- Lazy Loading: Load non-critical resources only when necessary.
- Minification and Compression: Use tools like Terser for minification, and enable Gzip or Brotli compression on the server-side.
Real-world Use Cases
Airbnb: Upon their transition to React, Airbnb implemented strict performance budgets, which resulted in improved page load times. They monitored the time-to-interaction metric in various environments, ensuring the effective use of dynamic imports for their key components.
Google Search: Google sets a performance budget implemented within their CI/CD pipeline. Each release undergoes performance scrutiny to adhere to defined metrics, resulting in consistently fast search experiences.
Potential Pitfalls
While performance budgeting is a robust strategy, pitfalls may arise:
- Underestimating the impact of third-party libraries: Including popular libraries can bloat your bundle. Regular audits for necessity and performance impact are critical.
- Overly strict budgets can be counterproductive: Setting unrealistic performance targets without understanding the trade-offs involved can lead to feature limitation.
Advanced Debugging Techniques
When budgets are exceeded, applying debugging techniques can clarify issues:
Chrome DevTools Performance Panel: Use this tool to record runtime performance, identify bottlenecks, and visualize JavaScript execution.
Lighthouse Audits: Conduct Lighthouse audits to provide insights into what contributes to load time and how your app adheres to its performance budget.
Performance Profiling: Leverage profiling tools from DevTools or the
performance
API to track significant resource usage.
Conclusion and Further Reading
Performance budgeting represents a critical component of seriously optimizing web applications. By establishing strict performance metrics and quantifying their impact on user experience, teams can significantly enhance the usability and responsiveness of their applications.
References and Resources
- Web Performance Budgets
- Google Lighthouse Documentation
- Understanding the Performance API
- Webpack Performance Optimization
By following this comprehensive guide, developers will be equipped to implement effective performance budgeting practices in their own projects, paving the way for cleaner, faster, and more efficient JavaScript applications. Thus, elevating both user experience and business outcomes.