P 3- Looping
Looping in Java (In-Depth Guide) Looping is a fundamental concept in Java (and programming in general) that allows you to execute a block of code repeatedly based on a condition. Java provides several looping mechanisms, each suited for different scenarios. 1. for Loop The for loop is used when the number of iterations is known beforehand. Syntax for (initialization; condition; update) { // Code to execute } Example for (int i = 1; i
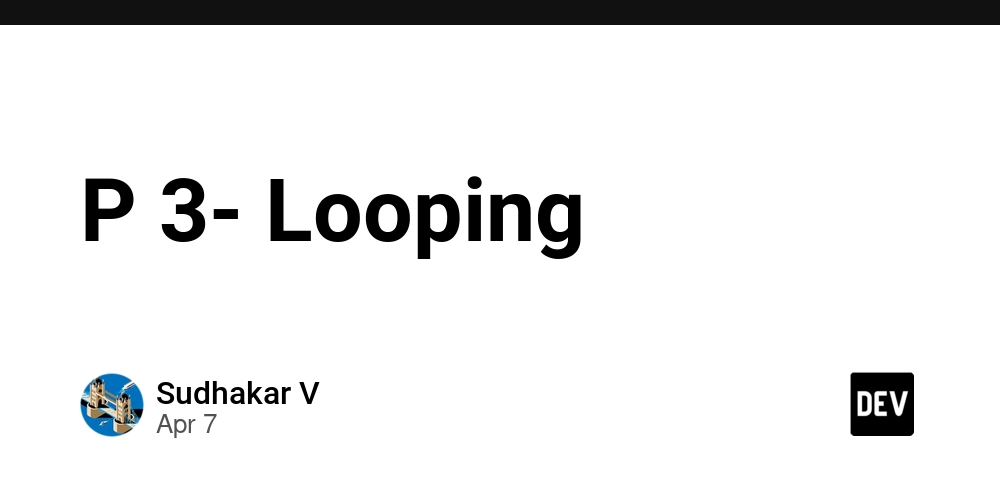
Looping in Java (In-Depth Guide)
Looping is a fundamental concept in Java (and programming in general) that allows you to execute a block of code repeatedly based on a condition. Java provides several looping mechanisms, each suited for different scenarios.
1. for
Loop
The for
loop is used when the number of iterations is known beforehand.
Syntax
for (initialization; condition; update) {
// Code to execute
}
Example
for (int i = 1; i <= 5; i++) {
System.out.println("Iteration: " + i);
}
Output:
Iteration: 1
Iteration: 2
Iteration: 3
Iteration: 4
Iteration: 5
Variations
a) Enhanced for
Loop (for-each)
Used for iterating over arrays and collections.
int[] numbers = {10, 20, 30, 40};
for (int num : numbers) {
System.out.println(num);
}
Output:
10
20
30
40
b) Infinite for
Loop
for (;;) {
System.out.println("This runs forever!");
}
2. while
Loop
The while
loop executes as long as the condition is true. It is used when the number of iterations is not known in advance.
Syntax
while (condition) {
// Code to execute
}
Example
int count = 1;
while (count <= 5) {
System.out.println("Count: " + count);
count++;
}
Output:
Count: 1
Count: 2
Count: 3
Count: 4
Count: 5
Infinite while
Loop
while (true) {
System.out.println("Infinite loop!");
}
3. do-while
Loop
Similar to while
, but guarantees at least one execution before checking the condition.
Syntax
do {
// Code to execute
} while (condition);
Example
int x = 5;
do {
System.out.println("x = " + x);
x--;
} while (x > 0);
Output:
x = 5
x = 4
x = 3
x = 2
x = 1
4. Nested Loops
A loop inside another loop is called a nested loop.
Example (Printing a Pyramid)
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= i; j++) {
System.out.print("* ");
}
System.out.println();
}
Output:
*
* *
* * *
* * * *
* * * * *
5. Loop Control Statements
Java provides keywords to alter loop execution:
Keyword | Description |
---|---|
break |
Exits the loop immediately |
continue |
Skips the current iteration and moves to the next |
return |
Exits the entire method |
Example: break
for (int i = 1; i <= 10; i++) {
if (i == 6) {
break; // Exit loop when i=6
}
System.out.println(i);
}
Output:
1
2
3
4
5
Example: continue
for (int i = 1; i <= 5; i++) {
if (i == 3) {
continue; // Skip iteration when i=3
}
System.out.println(i);
}
Output:
1
2
4
5
6. Best Practices for Loops
- Avoid infinite loops unless intentional (e.g., in servers).
-
Use
for
when the number of iterations is known. -
Use
while
when the condition is dynamic. -
Prefer
for-each
for collections (cleaner syntax). - Keep loop bodies small for readability.
-
Use
break
andcontinue
sparingly (can make code harder to debug).
Conclusion
-
for
loop → Best for fixed iterations. -
while
loop → Best for unknown iterations. -
do-while
loop → Ensures at least one execution. - Nested loops → Useful for multi-dimensional data.
-
break
&continue
→ Control loop flow.
Mastering loops is essential for efficient iteration in Java programs.