Optimizing Docker Image Builds for Speed & Efficiency
The Problem: Slow Docker Builds are a Bottleneck Docker images form the backbone of modern containerized applications, but slow image builds can significantly impact developer productivity. Every second wasted waiting for a build to complete adds up, slowing down CI/CD pipelines and delaying deployments. Common reasons for slow builds include: Large base images bloating the final image size Unoptimized Dockerfile instructions leading to inefficient caching Unnecessary dependencies increasing build times Frequent rebuilds due to changes in lower Dockerfile layers The Solution: Optimize Your Dockerfile for Performance By applying a few best practices, we can dramatically speed up Docker builds while keeping images lightweight and efficient. 1. Use a Minimal Base Image Large base images slow down builds and increase attack surfaces. Instead, opt for lightweight images like Alpine Linux: # Avoid large images like ubuntu:latest FROM alpine:latest Why? Alpine is only ~5MB compared to Ubuntu (~29MB) or Debian (~22MB). This means smaller download sizes and faster builds. 2. Leverage Docker Build Caching Docker caches layers from top to bottom. To maximize cache efficiency: # BAD: Installing dependencies AFTER copying source code invalidates cache FROM node:18-alpine WORKDIR /app COPY . . RUN npm install # ❌ Re-runs on every change CMD ["node", "index.js"] **Fix: **Move unchanging layers before copying source files: # GOOD: Dependencies installed first, source copied later FROM node:18-alpine WORKDIR /app COPY package.json package-lock.json ./ RUN npm install # ✅ Cached until package.json changes COPY . . CMD ["node", "index.js"] Now, unless package.json changes, npm install is cached, reducing rebuild time. 3. Use Multi-Stage Builds Multi-stage builds keep final images clean by discarding unnecessary build dependencies. # Stage 1: Build dependencies FROM golang:1.20 AS builder WORKDIR /app COPY . . RUN go build -o myapp # Stage 2: Use a minimal runtime image FROM alpine:latest WORKDIR /app COPY --from=builder /app/myapp . CMD ["./myapp"] Benefit: The final image only contains the Go binary, making it smaller & faster. 4. Reduce Unnecessary Layers Each RUN instruction creates a new layer. Minimize layers by chaining commands: # BAD: Multiple RUN commands create extra layers RUN apt-get update RUN apt-get install -y curl RUN rm -rf /var/lib/apt/lists/* Fix: Combine them into a single RUN command: # GOOD: Reduces layer count RUN apt-get update && \ apt-get install -y curl && \ rm -rf /var/lib/apt/lists/* This minimizes image size and speeds up builds. Use .dockerignore to Exclude Unnecessary Files Docker builds everything in the build context. Exclude unnecessary files like logs, node_modules, and build artifacts: .dockerignore: node_modules/ .git/ *.log .env This reduces the build context size, leading to faster builds and reduced resource usage. Final Thoughts By following these best practices, you can significantly reduce Docker image size, improve build speed, and enhance CI/CD efficiency. Small optimizations can have big productivity gains!
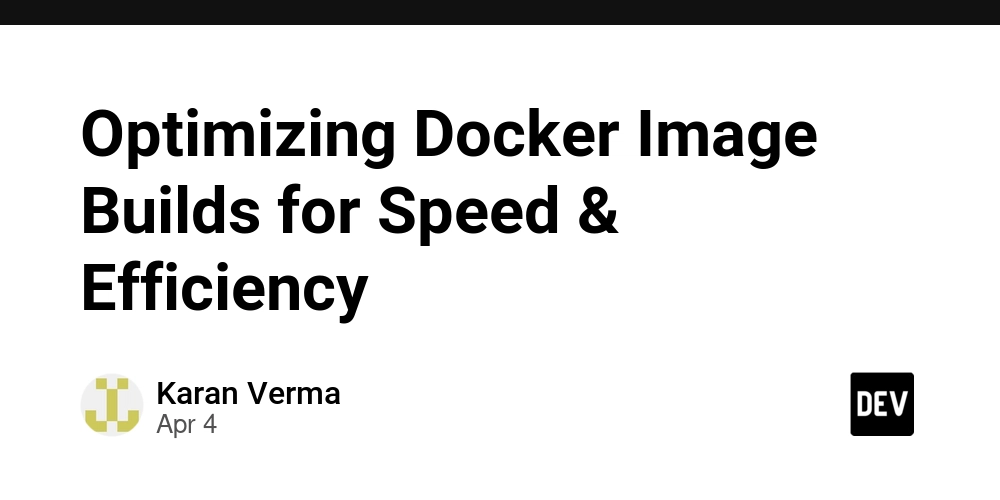
The Problem: Slow Docker Builds are a Bottleneck
Docker images form the backbone of modern containerized applications, but slow image builds can significantly impact developer productivity. Every second wasted waiting for a build to complete adds up, slowing down CI/CD pipelines and delaying deployments.
Common reasons for slow builds include:
- Large base images bloating the final image size
- Unoptimized Dockerfile instructions leading to inefficient caching
- Unnecessary dependencies increasing build times
- Frequent rebuilds due to changes in lower Dockerfile layers
The Solution: Optimize Your Dockerfile for Performance
By applying a few best practices, we can dramatically speed up Docker builds while keeping images lightweight and efficient.
1. Use a Minimal Base Image
Large base images slow down builds and increase attack surfaces. Instead, opt for lightweight images like Alpine Linux:
# Avoid large images like ubuntu:latest
FROM alpine:latest
Why? Alpine is only ~5MB compared to Ubuntu (~29MB) or Debian (~22MB). This means smaller download sizes and faster builds.
2. Leverage Docker Build Caching
Docker caches layers from top to bottom. To maximize cache efficiency:
# BAD: Installing dependencies AFTER copying source code invalidates cache
FROM node:18-alpine
WORKDIR /app
COPY . .
RUN npm install # ❌ Re-runs on every change
CMD ["node", "index.js"]
**Fix: **Move unchanging layers before copying source files:
# GOOD: Dependencies installed first, source copied later
FROM node:18-alpine
WORKDIR /app
COPY package.json package-lock.json ./
RUN npm install # ✅ Cached until package.json changes
COPY . .
CMD ["node", "index.js"]
Now, unless package.json
changes, npm install
is cached, reducing rebuild time.
3. Use Multi-Stage Builds
Multi-stage builds keep final images clean by discarding unnecessary build dependencies.
# Stage 1: Build dependencies
FROM golang:1.20 AS builder
WORKDIR /app
COPY . .
RUN go build -o myapp
# Stage 2: Use a minimal runtime image
FROM alpine:latest
WORKDIR /app
COPY --from=builder /app/myapp .
CMD ["./myapp"]
Benefit: The final image only contains the Go binary, making it smaller & faster.
4. Reduce Unnecessary Layers
Each RUN
instruction creates a new layer. Minimize layers by chaining commands:
# BAD: Multiple RUN commands create extra layers
RUN apt-get update
RUN apt-get install -y curl
RUN rm -rf /var/lib/apt/lists/*
Fix: Combine them into a single RUN
command:
# GOOD: Reduces layer count
RUN apt-get update && \
apt-get install -y curl && \
rm -rf /var/lib/apt/lists/*
This minimizes image size and speeds up builds.
Use .dockerignore to Exclude Unnecessary Files
Docker builds everything in the build context. Exclude unnecessary files like logs, node_modules
, and build artifacts:
.dockerignore:
node_modules/
.git/
*.log
.env
This reduces the build context size, leading to faster builds and reduced resource usage.
Final Thoughts
By following these best practices, you can significantly reduce Docker image size, improve build speed, and enhance CI/CD efficiency. Small optimizations can have big productivity gains!