Optimistically updating the UI with the useOptimistic hook
In summary, useOptimistic is a React hook that displays a different state only while some asynchronous action is in progress. Most basic use case is to make asynchronous data visible on UI while it's still on it's way to the database. It's a great tool to build highly reactive UI's and increase the user experience. It's the most underrated React hook in my opinion. SIMPLE USE CASE In my little example app i implemented useOptimistic and Tanstack Query together to add users' comments on the UI before it reaches the database. In my simple Invoice app i used useOptimistic hook for updating the status of the invoice. First you have to import the hook: import { useOptimistic } from 'react'; Use the useOptimistic hook with 2 arguments in it. const [currentStatus, setCurrentStatus] = useOptimistic( invoice.status, (state, newStatus) => { return String(newStatus); } ); Then, write a simple function to handle a form action: async function handleOnUpdateStatus(formData: FormData) { const originalStatus = currentStatus; setCurrentStatus(formData.get('status')); try { await updateStatusAction(formData); } catch (error) { setCurrentStatus(originalStatus); } } Then add that function to your form using "onSubmit" event. {AVAILABLE_STATUSES.map((status) => ( {status.label} The result: Thank you for reading. Have fun.
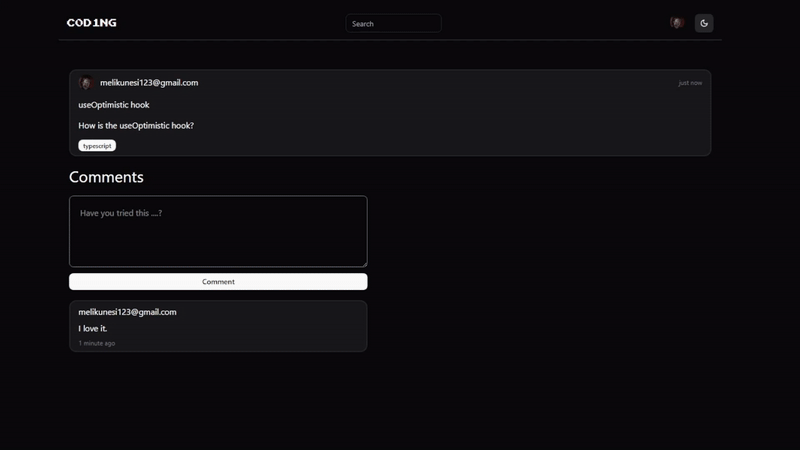
In summary, useOptimistic is a React hook that displays a different state only while some asynchronous action is in progress. Most basic use case is to make asynchronous data visible on UI while it's still on it's way to the database. It's a great tool to build highly reactive UI's and increase the user experience. It's the most underrated React hook in my opinion.
SIMPLE USE CASE
In my little example app i implemented useOptimistic and Tanstack Query together to add users' comments on the UI before it reaches the database.
In my simple Invoice app i used useOptimistic hook for updating the status of the invoice.
First you have to import the hook:
import { useOptimistic } from 'react';
Use the useOptimistic hook with 2 arguments in it.
const [currentStatus, setCurrentStatus] = useOptimistic(
invoice.status,
(state, newStatus) => {
return String(newStatus);
}
);
Then, write a simple function to handle a form action:
async function handleOnUpdateStatus(formData: FormData) {
const originalStatus = currentStatus;
setCurrentStatus(formData.get('status'));
try {
await updateStatusAction(formData);
} catch (error) {
setCurrentStatus(originalStatus);
}
}
Then add that function to your form using "onSubmit" event.
{AVAILABLE_STATUSES.map((status) => (
The result:
Thank you for reading. Have fun.