Necessary Python Concepts
Python is one of the most popular programming languages, known for its simplicity, readability, and versatility. Whether you're a beginner or an experienced developer, understanding these fundamental Python concepts will help you write better code and ace your interviews. Let’s dive in! 1. What is Python? Python is a high-level, interpreted programming language known for its simplicity and readability. It emphasizes clean and concise coding, making it a favorite among developers for web development, data analysis, scientific computing, and more. 2. Key Features of Python Easy-to-read syntax. Dynamic typing. Automatic memory management. Extensive standard library. Support for multiple programming paradigms (procedural, object-oriented, functional). 3. How is Python Different from Other Languages? Python stands out due to its simplicity, readability, and easy-to-understand syntax. It has a large and active community, extensive libraries, and is widely used across various domains like web development, data science, and automation. 4. Python Modules Python modules are files containing Python code that define functions, classes, and variables. They allow code reuse and organization, making it easier to manage and maintain larger projects. 5. Python Packages A Python package is a way to organize related modules into a directory hierarchy. It allows for logical grouping of modules, making it easier to manage and distribute code. 6. Comments in Python Comments in Python are denoted by the # character. Anything after # is considered a comment and is ignored by the interpreter. 7. Python Data Types Python supports various data types, including: Integers Floating-point numbers Strings Lists Tuples Dictionaries Booleans Each data type has its own characteristics and uses. 8. Type Conversion in Python Type conversion (or type casting) is the process of converting one data type into another. Python provides built-in functions like int(), float(), and str() for this purpose. 9. String Interpolation in Python String interpolation allows you to embed expressions or variables within a string. It can be done using f-strings or the format() method. 10. Conditional Statements in Python Conditional statements (if, elif, else) allow you to perform different actions based on certain conditions. They control the flow of the program based on the truthfulness of conditions. 11. Python Loops Python loops (for and while) enable you to execute a block of code repeatedly. They iterate over a sequence or execute until a specific condition is met. 12. Python Functions Functions are reusable blocks of code that perform a specific task. They help in code organization, reusability, and modularity. Functions can accept arguments and return values. 13. The __init__ Method The __init__ method is a special method in Python classes that is automatically called when an object is created. It is used to initialize the object's attributes and perform setup tasks. 14. Object-Oriented Programming (OOP) OOP is a programming paradigm that organizes code into objects, which are instances of classes. It emphasizes encapsulation, inheritance, and polymorphism. 15. Python Classes and Objects A class is a blueprint that defines the properties and behaviors of objects. An object is an instance of a class, representing a specific entity. 16. Creating an Object An object is created by calling the class as if it were a function. The class acts as a constructor, initializing the object and returning it. 17. Inheritance in Python Inheritance allows a class to inherit properties and methods from another class. It enables code reuse and supports hierarchical class structures. 18. Method Overriding Method overriding is the process of defining a method in a subclass that has the same name as a method in its superclass. The subclass method overrides the implementation of the superclass method. 19. Method Overloading Python does not directly support method overloading. However, you can achieve similar functionality using default argument values or variable-length arguments. 20. Generators in Python A generator is a function that returns an iterator. It allows you to generate a sequence of values on the fly, conserving memory and improving performance. 21. Decorators in Python Decorators are used to modify the behavior of a function or class without changing its source code. They are defined using the @decorator_name syntax and are commonly used for logging, timing, or modifying function arguments. 22. Lambda Functions A lambda function is an anonymous function defined using the lambda keyword. It is a shorthand way to create small, one-line
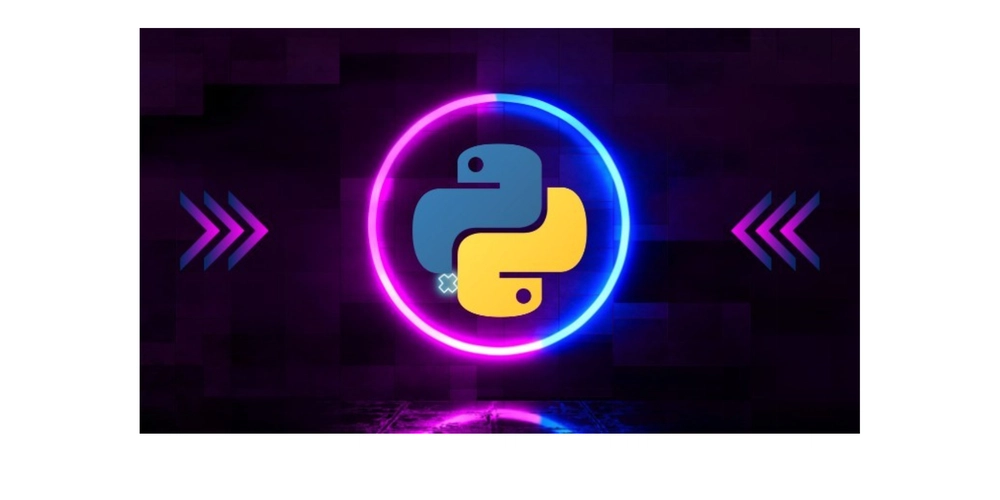
Python is one of the most popular programming languages, known for its simplicity, readability, and versatility. Whether you're a beginner or an experienced developer, understanding these fundamental Python concepts will help you write better code and ace your interviews. Let’s dive in!
1. What is Python?
Python is a high-level, interpreted programming language known for its simplicity and readability. It emphasizes clean and concise coding, making it a favorite among developers for web development, data analysis, scientific computing, and more.
2. Key Features of Python
- Easy-to-read syntax.
- Dynamic typing.
- Automatic memory management.
- Extensive standard library.
- Support for multiple programming paradigms (procedural, object-oriented, functional).
3. How is Python Different from Other Languages?
Python stands out due to its simplicity, readability, and easy-to-understand syntax. It has a large and active community, extensive libraries, and is widely used across various domains like web development, data science, and automation.
4. Python Modules
Python modules are files containing Python code that define functions, classes, and variables. They allow code reuse and organization, making it easier to manage and maintain larger projects.
5. Python Packages
A Python package is a way to organize related modules into a directory hierarchy. It allows for logical grouping of modules, making it easier to manage and distribute code.
6. Comments in Python
Comments in Python are denoted by the #
character. Anything after #
is considered a comment and is ignored by the interpreter.
7. Python Data Types
Python supports various data types, including:
- Integers
- Floating-point numbers
- Strings
- Lists
- Tuples
- Dictionaries
- Booleans
Each data type has its own characteristics and uses.
8. Type Conversion in Python
Type conversion (or type casting) is the process of converting one data type into another. Python provides built-in functions like int()
, float()
, and str()
for this purpose.
9. String Interpolation in Python
String interpolation allows you to embed expressions or variables within a string. It can be done using f-strings or the format()
method.
10. Conditional Statements in Python
Conditional statements (if
, elif
, else
) allow you to perform different actions based on certain conditions. They control the flow of the program based on the truthfulness of conditions.
11. Python Loops
Python loops (for
and while
) enable you to execute a block of code repeatedly. They iterate over a sequence or execute until a specific condition is met.
12. Python Functions
Functions are reusable blocks of code that perform a specific task. They help in code organization, reusability, and modularity. Functions can accept arguments and return values.
13. The __init__
Method
The __init__
method is a special method in Python classes that is automatically called when an object is created. It is used to initialize the object's attributes and perform setup tasks.
14. Object-Oriented Programming (OOP)
OOP is a programming paradigm that organizes code into objects, which are instances of classes. It emphasizes encapsulation, inheritance, and polymorphism.
15. Python Classes and Objects
- A class is a blueprint that defines the properties and behaviors of objects.
- An object is an instance of a class, representing a specific entity.
16. Creating an Object
An object is created by calling the class as if it were a function. The class acts as a constructor, initializing the object and returning it.
17. Inheritance in Python
Inheritance allows a class to inherit properties and methods from another class. It enables code reuse and supports hierarchical class structures.
18. Method Overriding
Method overriding is the process of defining a method in a subclass that has the same name as a method in its superclass. The subclass method overrides the implementation of the superclass method.
19. Method Overloading
Python does not directly support method overloading. However, you can achieve similar functionality using default argument values or variable-length arguments.
20. Generators in Python
A generator is a function that returns an iterator. It allows you to generate a sequence of values on the fly, conserving memory and improving performance.
21. Decorators in Python
Decorators are used to modify the behavior of a function or class without changing its source code. They are defined using the @decorator_name
syntax and are commonly used for logging, timing, or modifying function arguments.
22. Lambda Functions
A lambda function is an anonymous function defined using the lambda
keyword. It is a shorthand way to create small, one-line functions.
23. Virtual Environments
A virtual environment is a self-contained directory that contains a specific version of Python and installed packages. It isolates Python environments for different projects, making dependency management easier.
24. Error Handling in Python
Errors are events that disrupt the normal flow of a program. They can be handled using try-except
blocks to gracefully manage exceptions.
25. The try-except-else-finally
Block
-
try
: Contains code that may raise an exception. -
except
: Handles specific exceptions. -
else
: Executes if no exceptions occur. -
finally
: Always executes, regardless of exceptions.
26. List Comprehensions
List comprehensions provide a concise way to create lists based on existing lists or iterables. They combine looping and conditional logic in a single line.
27. The self
Parameter
The self
parameter is a reference to the current instance of a class. It is used to access class attributes and methods.
28. Deep Copy vs. Shallow Copy
- Shallow Copy: Creates a new object that references the original data.
- Deep Copy: Creates a new object with independent copies of the original data.
29. Global Interpreter Lock (GIL)
The GIL is a mechanism in CPython that allows only one thread to execute Python bytecode at a time. This can impact performance in multi-threaded programs.
30. Metaclasses in Python
A metaclass is a class that defines the behavior and structure of other classes. It allows you to customize class creation and add additional functionality.
31. The __name__
Variable
The __name__
variable represents the current module's name. It is used to determine whether a module is being run as the main script or imported.
32. Shallow vs. Deep Comparison
-
Shallow Comparison: Checks if two objects have the same memory address (
is
operator). -
Deep Comparison: Checks if two objects have the same values (
==
operator).
33. The if __name__ == "__main__"
Block
This block defines the entry point of a Python program. The code inside it executes only if the script is run directly, not when imported as a module.
34. The __str__
Method
The __str__
method returns a human-readable string representation of an object. It is called by the str()
function and print()
.
35. The __repr__
Method
The __repr__
method returns a string representation of an object that can be used to recreate it. It is called by the repr()
function.
36. The super()
Function
The super()
function is used to call a method in a superclass. It is often used in method overriding to invoke the superclass's implementation.
37. The __getitem__
Method
The __getitem__
method allows objects to define behavior for indexing and slicing operations.
38. The __setitem__
Method
The __setitem__
method allows objects to define behavior for assigning values to items using indexing.
39. The __iter__
Method
The __iter__
method returns an iterator object, making an object iterable.
40. The @property
Decorator
The @property
decorator defines a method as a getter for a class attribute, allowing it to be accessed like a normal attribute.
41. The @staticmethod
Decorator
The @staticmethod
decorator defines a static method in a class. Static methods do not require an instance and can be called directly from the class.
42. The @classmethod
Decorator
The @classmethod
decorator defines a method that operates on the class itself rather than an instance. It takes the class (cls
) as its first argument.
43. Context Managers
Context managers (with
statement) allow for resource allocation and deallocation, such as automatically closing a file after use.
44. The __enter__
and __exit__
Methods
These methods define the behavior of context managers. __enter__
is called when entering the with
block, and __exit__
is called when exiting.
45. The __call__
Method
The __call__
method allows an object to be called like a function.
46. The __slots__
Attribute
The __slots__
attribute is used to explicitly declare data members in a class, reducing memory usage.
47. The __dict__
Attribute
The __dict__
attribute stores the namespace of a class or instance as a dictionary.
48. The __annotations__
Attribute
The __annotations__
attribute stores type hints for variables, functions, and classes.
49. The __import__
Function
The __import__
function is used to dynamically import modules at runtime.
50. The __file__
Attribute
The __file__
attribute stores the path of the current module.
Python is a powerful and versatile language with a rich set of features. By mastering these concepts, you'll be well-equipped to tackle a wide range of programming challenges.