Mutually Exclusive Checkbox Selection with Reactive Forms
I need some assistance with handling checkbox selection using angular. I have 4 checkboxes, ALL, FirstName, LastName, MiddleName. When the page loads ALL should be the only one selected. If I click any of the other boxes ALL should be deselected. Likewise if I click ALL again the other checkboxes should be deselected. Currently ALL is selected when the page loads. I then can click the other boxes. However I can't click ALL unless I manually uncheck the other boxes. Any assistance you can provide will be greatly appreciated. This is my first post so please excuse the formatting :). — parent html —parent ts this.reportListFG = this.fb.group( { Address: [[]], PhoneNumber: [[]], filterGroup: this.fb.group({ ALL: [true], FirstName: [false], LastName: [false], MiddleName: [false], }), ) //Listen to form changes and handle the logic for "All" and other checkboxes this.reportListFG.get('filterGroup').valueChanges.subscribe(values => { this.handleCheckboxSelection(values); }); } handleCheckboxSelection(values: any) { const allSelected = values.ALL; console.log('Inside handleCheckbox'); // If "All" is selected and another box is checked, uncheck "All" if (allSelected && (values.FirstName || values.LastName || values.MiddleName)) { this.reportListFG.get('filterGroup')?.patchValue( { ALL: false }, { emitEvent: false } // Prevent infinite loop ); } // If "All" is selected, ensure other checkboxes remain unchecked else if (allSelected) { console.log(allSelected, '********'); this.reportListFG.get('filterGroup')?.patchValue( { FirstName: false, LastName: false, MiddleName: false }, { emitEvent: false } // Prevent infinite loop ); } }
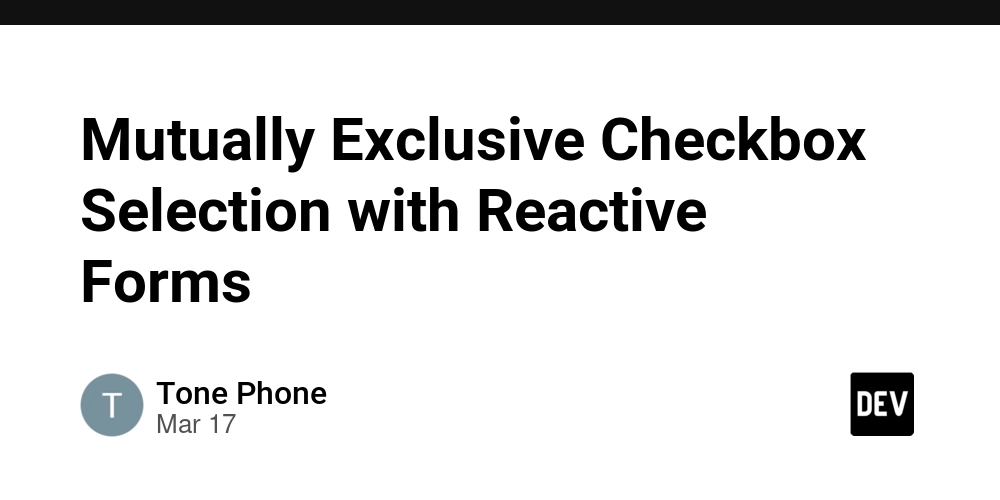
I need some assistance with handling checkbox selection using angular. I have 4 checkboxes, ALL, FirstName, LastName, MiddleName. When the page loads ALL should be the only one selected. If I click any of the other boxes ALL should be deselected. Likewise if I click ALL again the other checkboxes should be deselected. Currently ALL is selected when the page loads. I then can click the other boxes. However I can't click ALL unless I manually uncheck the other boxes. Any assistance you can provide will be greatly appreciated. This is my first post so please excuse the formatting :).
— parent html
—parent ts
this.reportListFG = this.fb.group(
{
Address: [[]],
PhoneNumber: [[]],
filterGroup: this.fb.group({
ALL: [true],
FirstName: [false],
LastName: [false],
MiddleName: [false],
}),
)
//Listen to form changes and handle the logic for "All" and other
checkboxes
this.reportListFG.get('filterGroup').valueChanges.subscribe(values =>
{
this.handleCheckboxSelection(values);
});
}
handleCheckboxSelection(values: any) {
const allSelected = values.ALL;
console.log('Inside handleCheckbox');
// If "All" is selected and another box is checked, uncheck "All"
if (allSelected && (values.FirstName || values.LastName ||
values.MiddleName)) {
this.reportListFG.get('filterGroup')?.patchValue(
{
ALL: false
},
{ emitEvent: false } // Prevent infinite loop
);
}
// If "All" is selected, ensure other checkboxes remain unchecked
else if (allSelected) {
console.log(allSelected, '********');
this.reportListFG.get('filterGroup')?.patchValue(
{
FirstName: false,
LastName: false,
MiddleName: false
},
{ emitEvent: false } // Prevent infinite loop
);
}
}