Multi-Environment Portfolio Website Pipeline: My Journey with Pulumi and GitHub
This is a submission for the Pulumi Deploy and Document Challenge under both the "Fast Static Website Deployment" and "Get Creative with Pulumi and GitHub" categories. What I Built I built a multi-environment portfolio website pipeline using Pulumi's Infrastructure as Code capabilities. The solution deploys static websites to AWS with separate environments (dev, staging, production), automated CI/CD through GitHub Actions, and comprehensive monitoring. It showcases how Pulumi can orchestrate AWS services (S3, CloudFront, ACM, CloudWatch), Cloudflare DNS management, and GitHub repository configuration from a single codebase. ✨ You can see the live sites here: Production: https://jacksonkasi.xyz/ Development/Preview: https://dev.jacksonkasi.xyz/ Project Repositories Infrastructure Code: portfolio-website-pipeline This repo contains the Pulumi infrastructure code that provisions all resources. Website Repository (created by Pulumi): portfolio-website-pipeline-new This repo is automatically created and configured by Pulumi with CI/CD workflows. Technical Core Components The infrastructure consists of several key components defined as Pulumi resources: S3 Website Hosting: export const siteBucket = new aws.s3.Bucket(`${namePrefix}-bucket`, { bucket: environmentDomain, website: { indexDocument: "index.html", errorDocument: "error.html", }, tags, }); CloudFront Distribution: export const distribution = new aws.cloudfront.Distribution( `${namePrefix}-distribution`, { enabled: true, isIpv6Enabled: true, defaultRootObject: "index.html", aliases: [environmentDomain], origins: [ { domainName: siteBucket.bucketRegionalDomainName, originId: siteBucket.arn, s3OriginConfig: { originAccessIdentity: originAccessIdentity.cloudfrontAccessIdentityPath, }, }, ], // Additional configuration... } ); GitHub Repository and CI/CD Automation: export const repo = new github.Repository( `${namePrefix}-repo`, { name: environment === "prod" ? "portfolio-website-pipeline-prod" : "portfolio-website-pipeline-new", visibility: "private", hasIssues: true, // Additional configuration... }, { provider: githubProvider, protect: true } ); // GitHub Actions workflow automation export const workflowFile = new github.RepositoryFile( `${namePrefix}-workflow-file`, { repository: repo.name, file: ".github/workflows/deploy.yml", content: workflowContent, branch: "main", // Additional configuration... } ); DNS Management with Cloudflare: export const mainRecord = new cloudflare.Record( `${namePrefix}-record`, { zoneId: zone.id, name: environment === "prod" ? "@" : environment, content: distribution.domainName.apply(name => name), type: "CNAME", proxied: false, ttl: 1, allowOverwrite: true, }, { provider: cloudflareProvider } ); Prerequisites To use this solution, you'll need: Development Environment: Pulumi CLI (v3.0+) Node.js (v14+) Git Cloud Accounts: AWS Account with IAM user having permissions for: S3, CloudFront, ACM, CloudWatch, Secrets Manager GitHub Account with: Personal access token (repo and workflow permissions) Cloudflare Account with: Registered domain with DNS managed by Cloudflare API token with Zone Read and DNS Edit permissions Configuration: Domain name registered and managed in Cloudflare AWS access/secret keys GitHub personal access token Pulumi access token For detailed setup instructions and complete configuration steps, please refer to the comprehensive README.md in the GitHub repository. My Journey Getting Started I began with mapping out the architecture for a robust multi-environment deployment system. I needed a solution that would: Deploy to different environments (dev, staging, prod) with consistent infrastructure Provide global content delivery with proper SSL/TLS Automate the entire CI/CD process Include monitoring and alerting Challenges and How I Fixed Them 1. Managing Environment-Specific Configurations Challenge: Maintaining separate configurations for each environment without duplicating code. Solution: I leveraged Pulumi's stack feature to create environment-specific configurations: export const environment = config.require("environment"); export const domain = config.require("domain"); export const environmentDomain = environment === "prod" ? domain : `${environment}.${domain}`; This approach allowed conditional resource creation based on the active environment: export const dashboard = environment === "prod" ? new aws.cloudwatch.Dashboard(/* production config */) : undefined; 2. Cloudflare DNS and AWS Certificate Integration Challenge: Certifica
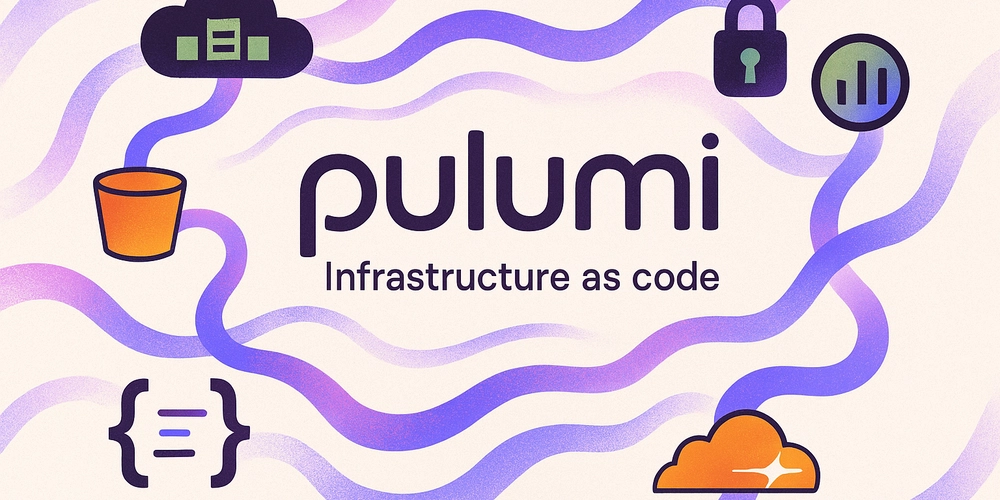
This is a submission for the Pulumi Deploy and Document Challenge under both the "Fast Static Website Deployment" and "Get Creative with Pulumi and GitHub" categories.
What I Built
I built a multi-environment portfolio website pipeline using Pulumi's Infrastructure as Code capabilities. The solution deploys static websites to AWS with separate environments (dev, staging, production), automated CI/CD through GitHub Actions, and comprehensive monitoring. It showcases how Pulumi can orchestrate AWS services (S3, CloudFront, ACM, CloudWatch), Cloudflare DNS management, and GitHub repository configuration from a single codebase. ✨
You can see the live sites here:
- Production: https://jacksonkasi.xyz/
- Development/Preview: https://dev.jacksonkasi.xyz/
Project Repositories
Infrastructure Code:
portfolio-website-pipeline
This repo contains the Pulumi infrastructure code that provisions all resources.Website Repository (created by Pulumi):
portfolio-website-pipeline-new
This repo is automatically created and configured by Pulumi with CI/CD workflows.
Technical Core Components
The infrastructure consists of several key components defined as Pulumi resources:
- S3 Website Hosting:
export const siteBucket = new aws.s3.Bucket(`${namePrefix}-bucket`, {
bucket: environmentDomain,
website: {
indexDocument: "index.html",
errorDocument: "error.html",
},
tags,
});
- CloudFront Distribution:
export const distribution = new aws.cloudfront.Distribution(
`${namePrefix}-distribution`,
{
enabled: true,
isIpv6Enabled: true,
defaultRootObject: "index.html",
aliases: [environmentDomain],
origins: [
{
domainName: siteBucket.bucketRegionalDomainName,
originId: siteBucket.arn,
s3OriginConfig: {
originAccessIdentity: originAccessIdentity.cloudfrontAccessIdentityPath,
},
},
],
// Additional configuration...
}
);
- GitHub Repository and CI/CD Automation:
export const repo = new github.Repository(
`${namePrefix}-repo`,
{
name: environment === "prod" ? "portfolio-website-pipeline-prod" : "portfolio-website-pipeline-new",
visibility: "private",
hasIssues: true,
// Additional configuration...
},
{ provider: githubProvider, protect: true }
);
// GitHub Actions workflow automation
export const workflowFile = new github.RepositoryFile(
`${namePrefix}-workflow-file`,
{
repository: repo.name,
file: ".github/workflows/deploy.yml",
content: workflowContent,
branch: "main",
// Additional configuration...
}
);
- DNS Management with Cloudflare:
export const mainRecord = new cloudflare.Record(
`${namePrefix}-record`,
{
zoneId: zone.id,
name: environment === "prod" ? "@" : environment,
content: distribution.domainName.apply(name => name),
type: "CNAME",
proxied: false,
ttl: 1,
allowOverwrite: true,
},
{ provider: cloudflareProvider }
);
Prerequisites
To use this solution, you'll need:
-
Development Environment:
- Pulumi CLI (v3.0+)
- Node.js (v14+)
- Git
-
Cloud Accounts:
-
AWS Account with IAM user having permissions for:
- S3, CloudFront, ACM, CloudWatch, Secrets Manager
-
GitHub Account with:
- Personal access token (repo and workflow permissions)
-
Cloudflare Account with:
- Registered domain with DNS managed by Cloudflare
- API token with Zone Read and DNS Edit permissions
-
AWS Account with IAM user having permissions for:
-
Configuration:
- Domain name registered and managed in Cloudflare
- AWS access/secret keys
- GitHub personal access token
- Pulumi access token
For detailed setup instructions and complete configuration steps, please refer to the comprehensive README.md in the GitHub repository.
My Journey
Getting Started
I began with mapping out the architecture for a robust multi-environment deployment system. I needed a solution that would:
- Deploy to different environments (dev, staging, prod) with consistent infrastructure
- Provide global content delivery with proper SSL/TLS
- Automate the entire CI/CD process
- Include monitoring and alerting
Challenges and How I Fixed Them
1. Managing Environment-Specific Configurations
Challenge: Maintaining separate configurations for each environment without duplicating code.
Solution: I leveraged Pulumi's stack feature to create environment-specific configurations:
export const environment = config.require("environment");
export const domain = config.require("domain");
export const environmentDomain = environment === "prod" ? domain : `${environment}.${domain}`;
This approach allowed conditional resource creation based on the active environment:
export const dashboard = environment === "prod"
? new aws.cloudwatch.Dashboard(/* production config */)
: undefined;
2. Cloudflare DNS and AWS Certificate Integration
Challenge: Certificate validation required specific DNS records, and CloudFront requires certificates in the us-east-1 region.
Solution: I created a dedicated AWS provider for the us-east-1 region and automated DNS validation record creation:
// AWS provider for us-east-1 region (required for CloudFront certificates)
const usEast1 = new aws.Provider("us-east-1", { region: "us-east-1" });
// Certificate in us-east-1
export const certificate = new aws.acm.Certificate(
`${namePrefix}-certificate`,
{
domainName: domainNames[0],
subjectAlternativeNames: domainNames.length > 1 ? domainNames.slice(1) : undefined,
validationMethod: "DNS",
tags,
},
{ provider: usEast1 }
);
// DNS validation records
export const validationOptions = certificate.domainValidationOptions.apply(options => {
options.forEach((option, index) => {
// Create DNS validation records in Cloudflare
// ...
});
return options;
});
3. GitHub Repository and Workflow Automation
Challenge: Creating and configuring GitHub repositories programmatically while ensuring secrets are properly managed.
Solution: I used Pulumi's GitHub provider to create repositories and securely configure GitHub Actions secrets:
// Creating GitHub secrets for deployment
export const awsAccessKeySecret = new github.ActionsSecret(
`${namePrefix}-aws-key-secret`,
{
repository: repo.name,
secretName: "AWS_ACCESS_KEY_ID",
plaintextValue: awsAccessKeyId,
},
{ provider: githubProvider }
);
Lessons Learned
This project reinforced several important lessons:
- Infrastructure as Code Benefits: Pulumi enables consistent, repeatable deployments across environments
- DNS Complexity: DNS and certificate management require careful planning and understanding of propagation delays
- CI/CD Automation: Automating the entire pipeline significantly reduces operational overhead
Using Pulumi with GitHub
The combination of Pulumi and GitHub provided several key advantages:
- Complete Repository Automation: Pulumi not only provisions cloud resources but also creates and configures the GitHub repository itself
- Secure Secret Management: Sensitive credentials are stored securely and injected into GitHub Actions
- End-to-End CI/CD: The entire workflow from code commit to deployment is automated
The power of this integration is most evident in how Pulumi automatically creates GitHub workflows that then use the infrastructure Pulumi provisioned:
const workflowContent = `
name: Portfolio Website CI/CD
on:
push:
branches: [ main, develop ]
pull_request:
branches: [ main, develop ]
jobs:
build-and-test:
// Configuration...
deploy-dev:
if: github.ref == 'refs/heads/develop'
// Deploy to development environment...
deploy-prod:
if: github.ref == 'refs/heads/main'
// Deploy to production environment...
`;
export const workflowFile = new github.RepositoryFile(
`${namePrefix}-workflow-file`,
{
repository: repo.name,
file: ".github/workflows/deploy.yml",
content: workflowContent,
// Additional configuration...
}
);
Final Thoughts
This project demonstrates how Pulumi can create not just cloud infrastructure, but an entire deployment ecosystem that spans multiple services and providers. The result is a professional-grade portfolio website pipeline that's secure, scalable, and automated.
For detailed setup instructions, troubleshooting tips, and advanced configuration options, please refer to the comprehensive documentation in the GitHub repository README. The README includes step-by-step guides for setting up your own instance of this pipeline, along with detailed explanations of all components and configurations.