Move All Special Characters to the End of the String in Java: A Detailed Guide
In Java programming, string manipulation is a crucial skill that every developer should master. Strings, being one of the most commonly used data types, often require various operations such as reversing, finding substrings, concatenation, and more. One interesting problem that frequently comes up in Java tutorials and coding challenges is how to move all special characters in a string to the end while keeping the alphabetical characters intact. Special characters can be anything other than alphanumeric characters (letters and numbers), such as punctuation marks, spaces, or symbols like @, #, $, and so on. In real-world applications, there might be scenarios where you need to organize your string in a more structured manner, for example, to clean up user input, format data for display, or prepare it for processing. This is where this problem of shifting special characters to the end becomes relevant. Understanding the Problem To begin, let’s define the task clearly. Given a string that contains both alphanumeric characters and special characters, the goal is to move all the special characters to the end of the string while preserving the relative order of both alphanumeric characters and special characters. For example, consider the string: "ab!@cd#ef$" The result after moving all special characters to the end should look like: "abcdef!@#$" This means: The order of alphabetic characters ('a', 'b', 'c', 'd', 'e', 'f') remains the same. The special characters ('!', '@', '#', '$') are moved to the end in the order they originally appeared. Breaking Down the Solution Approach Before diving into the coding aspect, it is important to understand the steps logically. The most intuitive approach for solving this problem involves two major steps: Separate alphanumeric characters: Loop through the string and collect all alphanumeric characters (letters and digits) in one collection (such as a list or a string). Collect special characters: While iterating through the string, simultaneously collect all special characters in another collection (such as a list or a string). Combine the two collections: After processing the entire string, append the special characters to the end of the alphanumeric characters. Now let’s delve deeper into the steps. 1. Loop Through the String The first step is to loop through the string and check each character. You need to distinguish between alphanumeric characters and special characters. This can be done using the Character.isLetterOrDigit() method in Java, which returns true if a character is either a letter or a digit, and false if it’s a special character. This allows you to segregate the characters into two different collections. 2. Create Separate Collections Once the string is traversed, you will have two distinct collections: A collection (such as a StringBuilder or String) holding all the alphanumeric characters. A collection (such as a StringBuilder or String) holding all the special characters. You don’t need to worry about the order of characters at this point because both collections will maintain the order in which the characters appear in the original string. 3. Combine Alphanumeric and Special Characters Finally, after you’ve successfully collected the alphanumeric and special characters, you concatenate the two collections—first the alphanumeric characters, followed by the special characters. This combined result will give you the desired string with special characters moved to the end. Key Considerations Time Complexity The time complexity of this approach is O(n), where n is the length of the string. This is because we are simply traversing the string once to categorize the characters and then performing an O(n) concatenation operation to combine the results. This makes the approach efficient for even relatively large strings. Edge Cases It is always important to consider edge cases when solving problems. In this case, the following edge cases should be kept in mind: Empty Strings: If the string is empty, there are no characters to process, and the result should be an empty string as well. Strings with No Special Characters: If there are no special characters in the string, the result will be the same as the original string, since no modifications are needed. Strings with Only Special Characters: Similarly, if the string contains only special characters, the result will be the same as the original string because the characters will be "moved" to the end, but they are already at the end. Strings with Only Alphanumeric Characters: In this case, the result will again be the same as the original string, as there are no special characters to move. Conclusion Moving all special characters to the end of a string in Java is a great exercise in string manipulation. Through this problem, you not only gain familiarity with handling al
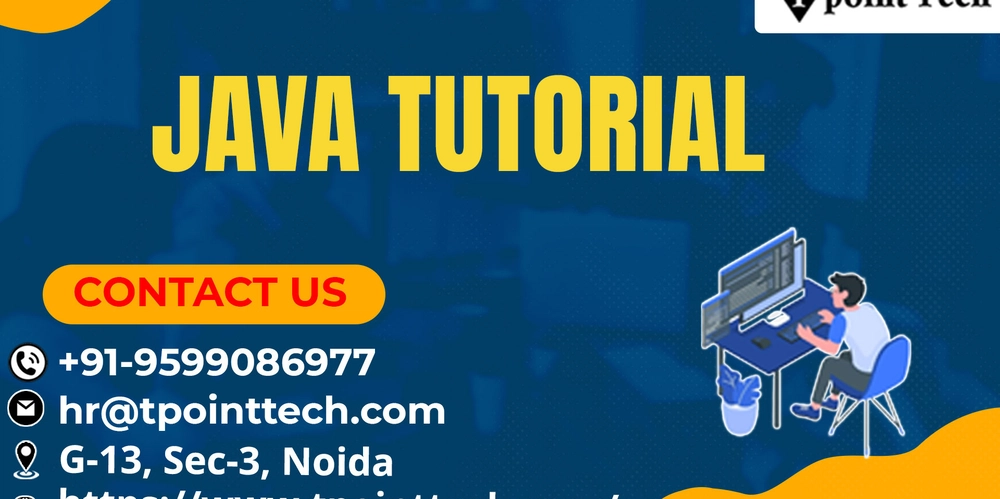
In Java programming, string manipulation is a crucial skill that every developer should master. Strings, being one of the most commonly used data types, often require various operations such as reversing, finding substrings, concatenation, and more. One interesting problem that frequently comes up in Java tutorials and coding challenges is how to move all special characters in a string to the end while keeping the alphabetical characters intact.
Special characters can be anything other than alphanumeric characters (letters and numbers), such as punctuation marks, spaces, or symbols like @
, #
, $
, and so on. In real-world applications, there might be scenarios where you need to organize your string in a more structured manner, for example, to clean up user input, format data for display, or prepare it for processing. This is where this problem of shifting special characters to the end becomes relevant.
Understanding the Problem
To begin, let’s define the task clearly. Given a string that contains both alphanumeric characters and special characters, the goal is to move all the special characters to the end of the string while preserving the relative order of both alphanumeric characters and special characters.
For example, consider the string:
"ab!@cd#ef$"
The result after moving all special characters to the end should look like:
"abcdef!@#$"
This means:
- The order of alphabetic characters ('a', 'b', 'c', 'd', 'e', 'f') remains the same.
- The special characters ('!', '@', '#', '$') are moved to the end in the order they originally appeared.
Breaking Down the Solution Approach
Before diving into the coding aspect, it is important to understand the steps logically. The most intuitive approach for solving this problem involves two major steps:
- Separate alphanumeric characters: Loop through the string and collect all alphanumeric characters (letters and digits) in one collection (such as a list or a string).
- Collect special characters: While iterating through the string, simultaneously collect all special characters in another collection (such as a list or a string).
- Combine the two collections: After processing the entire string, append the special characters to the end of the alphanumeric characters.
Now let’s delve deeper into the steps.
1. Loop Through the String
The first step is to loop through the string and check each character. You need to distinguish between alphanumeric characters and special characters. This can be done using the Character.isLetterOrDigit()
method in Java, which returns true
if a character is either a letter or a digit, and false
if it’s a special character. This allows you to segregate the characters into two different collections.
2. Create Separate Collections
Once the string is traversed, you will have two distinct collections:
- A collection (such as a StringBuilder or String) holding all the alphanumeric characters.
- A collection (such as a StringBuilder or String) holding all the special characters.
You don’t need to worry about the order of characters at this point because both collections will maintain the order in which the characters appear in the original string.
3. Combine Alphanumeric and Special Characters
Finally, after you’ve successfully collected the alphanumeric and special characters, you concatenate the two collections—first the alphanumeric characters, followed by the special characters. This combined result will give you the desired string with special characters moved to the end.
Key Considerations
Time Complexity
The time complexity of this approach is O(n), where n
is the length of the string. This is because we are simply traversing the string once to categorize the characters and then performing an O(n) concatenation operation to combine the results. This makes the approach efficient for even relatively large strings.
Edge Cases
It is always important to consider edge cases when solving problems. In this case, the following edge cases should be kept in mind:
- Empty Strings: If the string is empty, there are no characters to process, and the result should be an empty string as well.
- Strings with No Special Characters: If there are no special characters in the string, the result will be the same as the original string, since no modifications are needed.
- Strings with Only Special Characters: Similarly, if the string contains only special characters, the result will be the same as the original string because the characters will be "moved" to the end, but they are already at the end.
- Strings with Only Alphanumeric Characters: In this case, the result will again be the same as the original string, as there are no special characters to move.
Conclusion
Moving all special characters to the end of a string in Java is a great exercise in string manipulation. Through this problem, you not only gain familiarity with handling alphanumeric and special characters but also get to practice important concepts like loops, conditionals, and string concatenation. Additionally, understanding the logic behind this operation can help you in a variety of other real-world scenarios that require manipulating strings efficiently.
By following the steps outlined above, you can solve this problem with minimal effort and in an efficient manner, which is a common goal in Java tutorials. Whether you are preparing for an interview or learning to work with strings in Java, mastering such problems will enhance your skills as a developer. Keep exploring other string manipulation tasks as they are essential for writing clean and efficient code.
This solution can be easily extended to handle more complex string manipulations as well, providing a strong foundation for solving a wide range of programming challenges.