Microservices Patterns: A Comprehensive Guide
Microservices architecture has transformed modern software development by enabling scalability, resilience, and independent deployments. However, the complexity of distributed systems requires robust patterns to manage common challenges. This guide presents a detailed exploration of essential microservices patterns, categorized for easier understanding. 1. Service Decomposition Patterns Defining service boundaries is crucial to prevent future integration issues. 1.1. Decompose by Business Capability Break down services based on core business functions. Example: Inventory, Billing, and Shipping services. Pros: Aligns with organizational teams, promotes autonomy. Cons: Requires deep domain knowledge. 1.2. Decompose by Subdomain Leverages Domain-Driven Design (DDD) to split services based on bounded contexts. Example: Order Management, Product Catalog, Payment Processing. Pros: Reflects real-world domains, reduces coupling. Cons: Needs extensive domain analysis. 1.3. Strangler Fig Pattern Gradually replace monolithic components with microservices. Approach: Introduce new services alongside existing ones and redirect traffic progressively. Pros: Low-risk migration. Cons: Requires dual system maintenance. 2. Data Management Patterns Data decentralization is a key principle, but it introduces complexity. 2.1. Database per Service Each service owns and manages its database. Example: Order Service uses PostgreSQL, Inventory Service uses MongoDB. Pros: Loose coupling, service independence. Cons: Distributed data consistency challenges. 2.2. Shared Database Multiple services use a common database. Example: Order and Inventory services share a MySQL database. Pros: Simpler queries, easier consistency. Cons: Tight coupling, schema dependencies. 2.3. Saga Pattern Orchestrates distributed transactions using a sequence of local transactions with compensating actions. Example: Booking a trip involving flight, hotel, and car reservations. Pros: Ensures eventual consistency. Cons: Requires complex rollback logic. 2.4. CQRS (Command Query Responsibility Segregation) Separates read and write models for scalability. Example: Write operations update the data model; reads query precomputed views. Pros: Improves performance. Cons: Synchronization complexity. 2.5. Event Sourcing Captures changes as a sequence of immutable events. Example: Instead of storing an account balance, log transactions like Deposited, Withdrawn. Pros: Full audit trail, historical state reconstruction. Cons: Complex event storage and retrieval. 3. Communication Patterns Microservices must interact seamlessly across the network. 3.1. API Gateway Acts as a single entry point for clients. Example: Netflix Zuul. Pros: Centralized access control, load balancing. Cons: Single point of failure if not highly available. 3.2. Backend for Frontend (BFF) Custom API gateways for specific clients. Example: Separate BFF for mobile and web applications. Pros: Tailored experiences, reduced complexity for clients. Cons: Increased development effort. 3.3. Service Mesh Handles service-to-service communication. Example: Istio, Linkerd. Pros: Built-in observability, traffic management. Cons: Adds operational complexity. 3.4. Remote Procedure Invocation (RPI) Synchronous service communication via HTTP, gRPC. Pros: Simple request-response interaction. Cons: Network latency, tight coupling. 3.5. Asynchronous Messaging Event-driven or message queue-based communication. Example: Kafka, RabbitMQ. Pros: Loose coupling, resilience. Cons: Message ordering and duplication challenges. 4. Resilience Patterns Failure is inevitable; resilience patterns safeguard system stability. 4.1. Circuit Breaker Stops calls to failing services. Example: Netflix Hystrix. Pros: Prevents cascading failures. Cons: Threshold tuning complexity. 4.2. Retry Pattern Retries failed requests with backoff strategies. Pros: Enhances reliability. Cons: Risks overwhelming services if misconfigured. 4.3. Bulkhead Pattern Isolates services by partitioning resources. Pros: Limits failure impact. Cons: Resource allocation complexity. 4.4. Timeout Pattern Defines maximum waiting time for responses. Pros: Avoids blocking resources. Cons: Optimal timeout configuration. 5. Deployment Patterns Safe and efficient deployment is key in microservices. 5.1. Blue-Green Deployment Two production environments; traffic switches after updates. Pros: Zero downtime. Cons: Requires infrastructure duplication. 5.2. Canary Deployment Gradually roll out updates to a small subset of users. Pros: Detect issues early. Cons: Requires advanced monitoring. 5.3. Rolling Updates Updates instances incrementally. Pros: Co
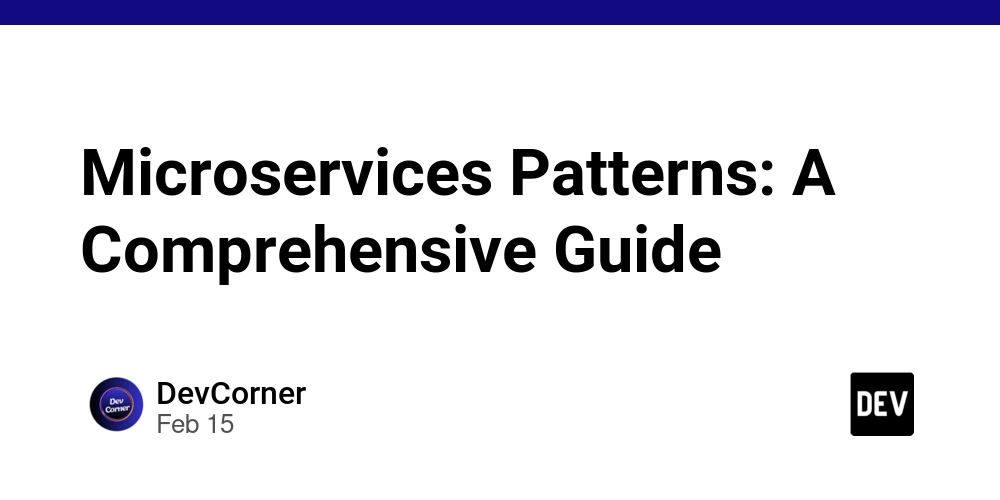
Microservices architecture has transformed modern software development by enabling scalability, resilience, and independent deployments. However, the complexity of distributed systems requires robust patterns to manage common challenges. This guide presents a detailed exploration of essential microservices patterns, categorized for easier understanding.
1. Service Decomposition Patterns
Defining service boundaries is crucial to prevent future integration issues.
1.1. Decompose by Business Capability
- Break down services based on core business functions.
- Example: Inventory, Billing, and Shipping services.
- Pros: Aligns with organizational teams, promotes autonomy.
- Cons: Requires deep domain knowledge.
1.2. Decompose by Subdomain
- Leverages Domain-Driven Design (DDD) to split services based on bounded contexts.
- Example: Order Management, Product Catalog, Payment Processing.
- Pros: Reflects real-world domains, reduces coupling.
- Cons: Needs extensive domain analysis.
1.3. Strangler Fig Pattern
- Gradually replace monolithic components with microservices.
- Approach: Introduce new services alongside existing ones and redirect traffic progressively.
- Pros: Low-risk migration.
- Cons: Requires dual system maintenance.
2. Data Management Patterns
Data decentralization is a key principle, but it introduces complexity.
2.1. Database per Service
- Each service owns and manages its database.
- Example: Order Service uses PostgreSQL, Inventory Service uses MongoDB.
- Pros: Loose coupling, service independence.
- Cons: Distributed data consistency challenges.
2.2. Shared Database
- Multiple services use a common database.
- Example: Order and Inventory services share a MySQL database.
- Pros: Simpler queries, easier consistency.
- Cons: Tight coupling, schema dependencies.
2.3. Saga Pattern
- Orchestrates distributed transactions using a sequence of local transactions with compensating actions.
- Example: Booking a trip involving flight, hotel, and car reservations.
- Pros: Ensures eventual consistency.
- Cons: Requires complex rollback logic.
2.4. CQRS (Command Query Responsibility Segregation)
- Separates read and write models for scalability.
- Example: Write operations update the data model; reads query precomputed views.
- Pros: Improves performance.
- Cons: Synchronization complexity.
2.5. Event Sourcing
- Captures changes as a sequence of immutable events.
- Example: Instead of storing an account balance, log transactions like Deposited, Withdrawn.
- Pros: Full audit trail, historical state reconstruction.
- Cons: Complex event storage and retrieval.
3. Communication Patterns
Microservices must interact seamlessly across the network.
3.1. API Gateway
- Acts as a single entry point for clients.
- Example: Netflix Zuul.
- Pros: Centralized access control, load balancing.
- Cons: Single point of failure if not highly available.
3.2. Backend for Frontend (BFF)
- Custom API gateways for specific clients.
- Example: Separate BFF for mobile and web applications.
- Pros: Tailored experiences, reduced complexity for clients.
- Cons: Increased development effort.
3.3. Service Mesh
- Handles service-to-service communication.
- Example: Istio, Linkerd.
- Pros: Built-in observability, traffic management.
- Cons: Adds operational complexity.
3.4. Remote Procedure Invocation (RPI)
- Synchronous service communication via HTTP, gRPC.
- Pros: Simple request-response interaction.
- Cons: Network latency, tight coupling.
3.5. Asynchronous Messaging
- Event-driven or message queue-based communication.
- Example: Kafka, RabbitMQ.
- Pros: Loose coupling, resilience.
- Cons: Message ordering and duplication challenges.
4. Resilience Patterns
Failure is inevitable; resilience patterns safeguard system stability.
4.1. Circuit Breaker
- Stops calls to failing services.
- Example: Netflix Hystrix.
- Pros: Prevents cascading failures.
- Cons: Threshold tuning complexity.
4.2. Retry Pattern
- Retries failed requests with backoff strategies.
- Pros: Enhances reliability.
- Cons: Risks overwhelming services if misconfigured.
4.3. Bulkhead Pattern
- Isolates services by partitioning resources.
- Pros: Limits failure impact.
- Cons: Resource allocation complexity.
4.4. Timeout Pattern
- Defines maximum waiting time for responses.
- Pros: Avoids blocking resources.
- Cons: Optimal timeout configuration.
5. Deployment Patterns
Safe and efficient deployment is key in microservices.
5.1. Blue-Green Deployment
- Two production environments; traffic switches after updates.
- Pros: Zero downtime.
- Cons: Requires infrastructure duplication.
5.2. Canary Deployment
- Gradually roll out updates to a small subset of users.
- Pros: Detect issues early.
- Cons: Requires advanced monitoring.
5.3. Rolling Updates
- Updates instances incrementally.
- Pros: Continuous service availability.
- Cons: Compatibility between versions.
5.4. Sidecar Pattern
- Auxiliary services run alongside main service.
- Example: Logging, monitoring agents.
- Pros: Enhances modularity.
- Cons: Increases resource consumption.
6. Observability Patterns
Insights into the system’s behavior enable proactive issue resolution.
6.1. Log Aggregation
- Centralizes logs across services.
- Example: ELK Stack.
- Pros: Easier debugging.
6.2. Distributed Tracing
- Tracks requests across services.
- Example: Jaeger, Zipkin.
- Pros: Identifies latency bottlenecks.
6.3. Metrics Collection
- Monitors performance indicators.
- Example: Prometheus.
- Pros: Real-time health monitoring.
6.4. Health Check Pattern
- Services expose endpoints to signal health.
- Pros: Supports automated recovery.
7. Security Patterns
Security Patterns are approaches to protect distributed systems, ensuring only authorized users and services can interact safely.
-
Access Token:
- Uses tokens like OAuth2 or JWT for user authentication.
- Tokens are issued after login and sent with every request to prove identity.
- Pros: Secure, stateless (no need to store session data on the server), scalable.
-
API Gateway Security:
- An API Gateway acts as a single entry point for all requests and applies security checks like authentication, rate limiting, and input validation.
- Pros: Centralizes security, ensures consistent protection across all services.
-
Mutual TLS (mTLS):
- Requires both client and server to authenticate each other using certificates during communication.
- Ensures encrypted, trusted connections between services.
- Pros: Prevents unauthorized access, secures internal service-to-service traffic.
8. Cross-cutting Patterns
Cross-cutting Patterns are design patterns that solve common problems across different systems, services, or applications. They are not specific to one component but affect the entire system.
-
Configuration Management:
- Centralized way to manage and update settings (like database URLs, API keys) for all services, instead of configuring each one separately.
- Ensures consistency and allows updates without redeploying applications.
-
Service Discovery:
- Automatically identifies and connects services within a distributed system.
- Helps services find each other without needing hard-coded addresses, especially useful in cloud environments.
-
Centralized Logging:
- Gathers logs from different services into a single location.
- Makes it easier to monitor, debug, and analyze system behavior, especially in complex distributed systems.
-
Distributed Caching:
- Stores frequently accessed data across multiple servers.
- Reduces load on databases and speeds up responses by serving data from nearby cache nodes.
These patterns improve system scalability, reliability, and ease of management in large, distributed environments.
Conclusion
Combining these patterns helps design scalable, resilient, and maintainable microservices architectures. Proper pattern selection is crucial to address specific challenges in distributed systems.