Mastering React Events: Understanding, Debugging, and Optimizing Event Handling
Events are the backbone of user interactions in any web application. Whether it's a button click, a form submission, or a keyboard shortcut, events dictate how users engage with our applications. React, like the DOM, provides an event system, but it's not just a direct wrapper around native events—it introduces a synthetic event system that optimizes and standardizes event handling across different browsers. At first glance, event handling in React seems straightforward—attach an event listener to a component, and React takes care of the rest. But as applications scale, handling events efficiently becomes crucial to preventing unexpected behavior, performance bottlenecks, and debugging nightmares. The Curious Case of the Disappearing Tooltip It started as a simple feature: A custom tooltip component that should appear when an element is hovered and disappear when the mouse leaves. Yet, during rapid user interactions—such as continuous clicking—the tooltip would sometimes fail to appear at all. Debugging this issue led to a deep dive into how React handles events, how propagation works, and why certain event handlers behave unexpectedly. This experience highlighted why understanding event flow, propagation, and optimizations is crucial for building robust React applications. Understanding React's Synthetic Event System Unlike traditional DOM event handling, React wraps native events with its own abstraction: SyntheticEvent. This system provides a consistent interface across browsers, improving event handling efficiency. Why Synthetic Events? Performance Optimizations: React pools events to reduce memory consumption. Instead of creating a new event object for every interaction, React reuses event objects and resets them after the callback executes. Cross-Browser Compatibility: React normalizes event properties across different browsers, so developers don't have to worry about inconsistencies. Event Delegation: Unlike direct DOM event listeners, React attaches a single event listener at the root (document or root container). This improves performance by reducing the number of event listeners in the DOM tree. How Synthetic Events Work When an event occurs, React: Captures the native event. Wraps it inside a SyntheticEvent. Calls the appropriate React event handlers. Returns the event to a pool for reuse. The Evolution of Event Pooling In React versions before 17, React reused event objects, meaning accessing properties like event.target asynchronously would return null. To persist event data, you had to use event.persist(). // Pre-React 17 approach const handleClick = (event: React.MouseEvent) => { event.persist(); // Prevents React from reusing the event object setTimeout(() => { console.log(event.target); // Accessible even after the event lifecycle }, 1000); }; But good news! Since React 17, event pooling has been removed. This means we no longer need to call event.persist() to access event properties asynchronously. React now creates a new event object for each event, which is more intuitive and easier to work with. // React 17+ approach const handleClick = (event: React.MouseEvent) => { // No need for event.persist() anymore! setTimeout(() => { console.log(event.target); // Works fine in React 17+ }, 1000); }; This change made a huge difference in my debugging process! I was initially calling persist() everywhere and later realized it wasn't needed anymore. Learning these version differences is part of the journey! Common React Events and Their Use Cases React categorizes events into several groups: 1. Mouse Events onClick, onDoubleClick, onMouseDown, onMouseUp onMouseEnter, onMouseLeave, onMouseOver, onMouseOut onContextMenu (Right-click handling) 2. Keyboard Events onKeyDown, onKeyUp 3. Form & Input Events onChange, onInput, onFocus, onBlur onSubmit, onReset 4. Clipboard Events onCopy, onCut, onPaste 5. Drag & Drop Events onDragStart, onDragEnd, onDragEnter, onDragLeave onDragOver, onDrop 6. Touch Events (Mobile) onTouchStart, onTouchMove, onTouchEnd, onTouchCancel 7. Focus Events onFocus, onBlur 8. Composition Events (IME input handling) onCompositionStart, onCompositionUpdate, onCompositionEnd 9. Wheel Events onWheel 10. Media Events onPlay, onPause, onEnded, onVolumeChange Event Propagation: Bubbling, Capturing, and Stopping Events Events in React follow the bubbling and capturing phases, just like native DOM events. This means an event can trigger multiple handlers as it moves from the target element up (bubbling) or down (capturing) the DOM tree. To prevent unwanted behaviors, we use: event.stopPropagation() – Prevents the event from reaching parent elements. event.preven
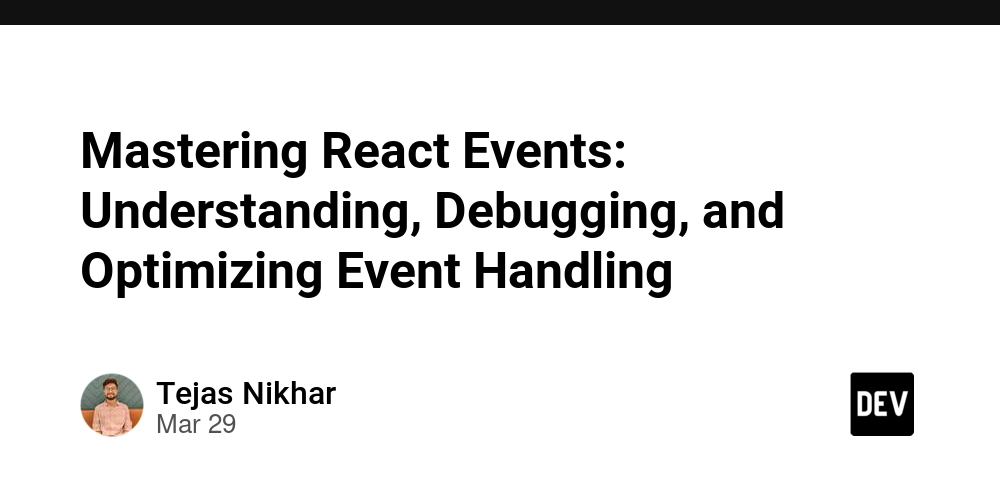
Events are the backbone of user interactions in any web application. Whether it's a button click, a form submission, or a keyboard shortcut, events dictate how users engage with our applications. React, like the DOM, provides an event system, but it's not just a direct wrapper around native events—it introduces a synthetic event system that optimizes and standardizes event handling across different browsers.
At first glance, event handling in React seems straightforward—attach an event listener to a component, and React takes care of the rest. But as applications scale, handling events efficiently becomes crucial to preventing unexpected behavior, performance bottlenecks, and debugging nightmares.
The Curious Case of the Disappearing Tooltip
It started as a simple feature: A custom tooltip component that should appear when an element is hovered and disappear when the mouse leaves. Yet, during rapid user interactions—such as continuous clicking—the tooltip would sometimes fail to appear at all. Debugging this issue led to a deep dive into how React handles events, how propagation works, and why certain event handlers behave unexpectedly.
This experience highlighted why understanding event flow, propagation, and optimizations is crucial for building robust React applications.
Understanding React's Synthetic Event System
Unlike traditional DOM event handling, React wraps native events with its own abstraction: SyntheticEvent. This system provides a consistent interface across browsers, improving event handling efficiency.
Why Synthetic Events?
- Performance Optimizations: React pools events to reduce memory consumption. Instead of creating a new event object for every interaction, React reuses event objects and resets them after the callback executes.
- Cross-Browser Compatibility: React normalizes event properties across different browsers, so developers don't have to worry about inconsistencies.
-
Event Delegation: Unlike direct DOM event listeners, React attaches a single event listener at the root (
document
orroot
container). This improves performance by reducing the number of event listeners in the DOM tree.
How Synthetic Events Work
When an event occurs, React:
- Captures the native event.
- Wraps it inside a
SyntheticEvent
. - Calls the appropriate React event handlers.
- Returns the event to a pool for reuse.
The Evolution of Event Pooling
In React versions before 17, React reused event objects, meaning accessing properties like event.target
asynchronously would return null
. To persist event data, you had to use event.persist()
.
// Pre-React 17 approach
const handleClick = (event: React.MouseEvent) => {
event.persist(); // Prevents React from reusing the event object
setTimeout(() => {
console.log(event.target); // Accessible even after the event lifecycle
}, 1000);
};
But good news! Since React 17, event pooling has been removed. This means we no longer need to call event.persist()
to access event properties asynchronously. React now creates a new event object for each event, which is more intuitive and easier to work with.
// React 17+ approach
const handleClick = (event: React.MouseEvent) => {
// No need for event.persist() anymore!
setTimeout(() => {
console.log(event.target); // Works fine in React 17+
}, 1000);
};
This change made a huge difference in my debugging process! I was initially calling persist()
everywhere and later realized it wasn't needed anymore. Learning these version differences is part of the journey!
Common React Events and Their Use Cases
React categorizes events into several groups:
1. Mouse Events
-
onClick
,onDoubleClick
,onMouseDown
,onMouseUp
-
onMouseEnter
,onMouseLeave
,onMouseOver
,onMouseOut
-
onContextMenu
(Right-click handling)
2. Keyboard Events
-
onKeyDown
,onKeyUp
3. Form & Input Events
-
onChange
,onInput
,onFocus
,onBlur
-
onSubmit
,onReset
4. Clipboard Events
-
onCopy
,onCut
,onPaste
5. Drag & Drop Events
-
onDragStart
,onDragEnd
,onDragEnter
,onDragLeave
-
onDragOver
,onDrop
6. Touch Events (Mobile)
-
onTouchStart
,onTouchMove
,onTouchEnd
,onTouchCancel
7. Focus Events
-
onFocus
,onBlur
8. Composition Events (IME input handling)
-
onCompositionStart
,onCompositionUpdate
,onCompositionEnd
9. Wheel Events
onWheel
10. Media Events
-
onPlay
,onPause
,onEnded
,onVolumeChange
Event Propagation: Bubbling, Capturing, and Stopping Events
Events in React follow the bubbling and capturing phases, just like native DOM events. This means an event can trigger multiple handlers as it moves from the target element up (bubbling) or down (capturing) the DOM tree.
To prevent unwanted behaviors, we use:
-
event.stopPropagation()
– Prevents the event from reaching parent elements. -
event.preventDefault()
– Prevents the default browser action.
const handleClick = (e: React.MouseEvent) => {
e.stopPropagation();
console.log("Click prevented from bubbling!");
};
Understanding the Event Flow
When I first started with React, I was confused about why my event handlers were triggering in what seemed like a random order. Then I learned about event flow:
- Capturing Phase: Event travels down from the root to the target element
- Target Phase: Event reaches the target element
- Bubbling Phase: Event bubbles up from the target back to the root
React event handlers typically operate during the bubbling phase, but you can capture events during the capturing phase by adding Capture
to the event name:
<div
onClickCapture={() => console.log("Capture phase!")}
onClick={() => console.log("Bubble phase!")}
>
<button onClick={() => console.log("Button clicked!")}>Click mebutton>
div>
When clicking the button, the logs will appear in this order:
- "Capture phase!" (during capturing)
- "Button clicked!" (at target)
- "Bubble phase!" (during bubbling)
This knowledge helped me solve many event-related issues!
Handling Events Like a Pro: Custom Wrappers
To manage events effectively, we can abstract common patterns into utility functions:
export const preventDefaultOnly = (e: React.MouseEvent | React.ChangeEvent) => {
e.preventDefault();
};
export const stopPropagationOnly = (e: React.MouseEvent | React.ChangeEvent) => {
e.stopPropagation();
};
export const preventAndStopEvent = (e: React.MouseEvent | React.ChangeEvent) => {
preventDefaultOnly(e);
stopPropagationOnly(e);
};
Debouncing and Throttling Events
Handling rapid event firing efficiently is crucial for performance. Here's an improved debouncing function that creates a separate timer for each handler:
export const createDebounceHandler = <T extends (...args: any[]) => void>(
handler: T,
delay: number = 300
): T => {
let timer: NodeJS.Timeout | null = null;
return ((...args: any[]) => {
if (timer) clearTimeout(timer);
timer = setTimeout(() => handler(...args), delay);
}) as T;
};
// Usage example
const debouncedSearch = createDebounceHandler((searchTerm) => {
// Search API call here
console.log("Searching for:", searchTerm);
}, 500);
Throttling for Scroll and Resize Events
Throttling is similar to debouncing but ensures a function runs at most once in a specified time interval:
export const createThrottleHandler = <T extends (...args: any[]) => void>(
handler: T,
interval: number = 300
): T => {
let lastRun: number = 0;
let timeout: NodeJS.Timeout | null = null;
return ((...args: any[]) => {
const now = Date.now();
if (now - lastRun >= interval) {
if (timeout) {
clearTimeout(timeout);
timeout = null;
}
lastRun = now;
handler(...args);
} else if (!timeout) {
timeout = setTimeout(
() => {
lastRun = Date.now();
timeout = null;
handler(...args);
},
interval - (now - lastRun)
);
}
}) as T;
};
// Usage example
const throttledScroll = createThrottleHandler(() => {
console.log("Scroll position:", window.scrollY);
}, 200);
I've learned that throttling works better for continuous events like scrolling, while debouncing is ideal for discrete events like typing or button clicks.
React Hooks and Event Handling
When I started using React Hooks, event handling got both easier and trickier! Here's what I've learned:
Using useCallback for Event Handlers
To prevent unnecessary re-renders, we can memoize event handlers with useCallback
:
import { useCallback, useState } from "react";
function SearchComponent() {
const [query, setQuery] = useState("");
// This handler is memoized and won't be recreated on each render
const handleInputChange = useCallback((e: React.ChangeEvent<HTMLInputElement>) => {
setQuery(e.target.value);
}, []); // Empty dependency array means this function never changes
return <input type="text" value={query} onChange={handleInputChange} />;
}
Adding Global Event Listeners with useEffect
Sometimes we need to listen for events outside our components:
import { useEffect, useState } from "react";
function KeyboardShortcuts() {
const [isCtrlPressed, setIsCtrlPressed] = useState(false);
useEffect(() => {
const handleKeyDown = (e: KeyboardEvent) => {
if (e.key === "Control") setIsCtrlPressed(true);
};
const handleKeyUp = (e: KeyboardEvent) => {
if (e.key === "Control") setIsCtrlPressed(false);
};
// Add global event listeners
window.addEventListener("keydown", handleKeyDown);
window.addEventListener("keyup", handleKeyUp);
// Cleanup function - VERY IMPORTANT to prevent memory leaks!
return () => {
window.removeEventListener("keydown", handleKeyDown);
window.removeEventListener("keyup", handleKeyUp);
};
}, []); // Empty dependency array means this only runs once
return <div>{isCtrlPressed ? "Ctrl is pressed" : "Ctrl is not pressed"}div>;
}
This pattern has been super helpful for keyboard shortcuts, click-outside detection, and other global interactions!
Common Event Handling Patterns
Controlled Forms
React's approach to forms is different from vanilla JavaScript. Instead of letting the DOM manage form state, we control it with React state:
import { useState } from "react";
function ContactForm() {
const [formData, setFormData] = useState({
name: "",
email: "",
message: "",
});
const handleChange = (e: React.ChangeEvent<HTMLInputElement | HTMLTextAreaElement>) => {
const { name, value } = e.target;
setFormData((prev) => ({
...prev,
[name]: value,
}));
};
const handleSubmit = (e: React.FormEvent) => {
e.preventDefault(); // Prevent browser from refreshing
console.log("Form submitted:", formData);
// Submit to API here
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
name="name"
value={formData.name}
onChange={handleChange}
placeholder="Your name"
/>
<input
type="email"
name="email"
value={formData.email}
onChange={handleChange}
placeholder="Your email"
/>
<textarea
name="message"
value={formData.message}
onChange={handleChange}
placeholder="Your message"
/>
<button type="submit">Sendbutton>
form>
);
}
Event Delegation in Lists
When rendering lists in React, it's more efficient to handle events at the parent level rather than attaching handlers to each item:
function TodoList({ todos }) {
const handleItemClick = (e: React.MouseEvent, todoId: string) => {
e.stopPropagation(); // Prevent bubbling if needed
console.log("Todo clicked:", todoId);
};
return (
<ul>
{todos.map((todo) => (
<li key={todo.id} onClick={(e) => handleItemClick(e, todo.id)}>
{todo.text}
li>
))}
ul>
);
}
React 18 Event Handling Considerations
React 18 introduced some changes that affect event handling:
Automatic Batching
Before React 18, updates inside event handlers were batched (multiple state updates resulted in a single render), but updates in promises, setTimeout, or native event handlers weren't batched.
React 18 introduced automatic batching for all updates, which improves performance but can change behavior:
// In React 18, these state updates are batched into a single render
function handleClick() {
setCount((c) => c + 1);
setFlag((f) => !f);
// Only ONE render will occur, not two!
}
useTransition for Non-Urgent Updates
React 18 introduced useTransition
to mark state updates as non-urgent, which helps with large updates:
import { useTransition, useState } from "react";
function SearchResults() {
const [query, setQuery] = useState("");
const [results, setResults] = useState([]);
const [isPending, startTransition] = useTransition();
const handleSearch = (e: React.ChangeEvent<HTMLInputElement>) => {
// Update input immediately (urgent update)
setQuery(e.target.value);
// Mark results update as non-urgent
startTransition(() => {
// This could be an expensive operation
const searchResults = performExpensiveSearch(e.target.value);
setResults(searchResults);
});
};
return (
<>
<input type="text" value={query} onChange={handleSearch} />
{isPending ? <p>Loading...p> : results.map(/* render results */)}
>
);
}
I've found this pattern really helpful when handling events that trigger expensive operations!
Performance Optimization Tips
Through trial and error, I've discovered several ways to optimize event handling:
Avoid Creating Functions in Render
Creating new functions on every render can cause unnecessary re-renders in child components:
//