Mastering React Debugging: 15 Essential Steps Every Developer Should Know
Bug Finding for React Developer Overview Bug finding in React development is an essential skill for creating robust and efficient applications. This guide outlines a systematic, step-by-step approach to identifying, isolating, and resolving issues within React applications. Following these steps, developers will enhance their debugging skills and ensure their applications are error-free and perform optimally. Step 1: Check the Console for Errors Before diving into the code, always begin with inspecting the browser’s console for any error messages. React will typically display informative messages in the console that can direct you to the issue. It could be an issue related to: Incorrectly used props or states Uncaught JavaScript errors Warnings related to missing or invalid keys in lists By reading these messages, you can narrow down where the problem might lie, whether it's with component rendering, data fetching, or user interactions. Step 2: Replicate the Issue Consistently Once an error message is spotted, make sure you can consistently replicate the issue. Try to identify specific actions or conditions under which the bug appears: Does the bug appear on a specific route or component? Is it triggered by a user interaction, such as a button click or form submission? Does it happen after a certain timeout or API response? By understanding exactly how to replicate the issue, you are better equipped to trace the cause. Step 3: Examine the Component Tree with React DevTools React DevTools is a powerful debugging tool that provides an in-depth view of your component hierarchy. It allows you to: Inspect the props and state of individual components Track component re-renders and performance Check for unnecessary re-renders that might be causing performance issues By inspecting the component tree, you can pinpoint which components are not receiving the correct props or state and whether they are re-rendering unnecessarily. Step 4: Check for Missing or Incorrect Props Props are a core feature in React for passing data to components. When bugs appear related to the UI not rendering as expected, check if: The parent component is passing the correct props to the child component. The child component is correctly receiving and using the props. There are missing props that should be provided. Ensure the types and values of props are consistent and match the expected structure. Step 5: Verify the State and State Updates State management issues are common culprits for bugs. In React, improper state initialization or incorrect state updates often lead to: UI not reflecting the latest data Performance issues due to unnecessary re-renders Verify that state is being initialized correctly, updated in response to user actions, and is in sync with the component's UI. Make sure state updates use setState (for class components) or the state setter function (useState) in functional components. Step 6: Ensure Proper Usage of Hooks For functional components, React hooks like useState, useEffect, and useContext are pivotal in managing state and side effects. Ensure: useState is being used properly for state updates. useEffect is being correctly used for side effects (e.g., data fetching, subscriptions). Hooks follow the rules of hooks, such as being called at the top level of the component and not inside loops or conditions. Incorrect hook usage can lead to issues like infinite loops, unexpected behavior, or improper re-renders. Step 7: Investigate Asynchronous Operations Many React applications rely on asynchronous operations such as fetching data from APIs. Issues can arise when asynchronous calls are: Not handled properly with async/await or promises. Missing error handling or fallback states (e.g., loading indicators or error messages). Triggered in an incorrect lifecycle method, resulting in race conditions. Ensure that your asynchronous code is executed correctly, and that UI states are updated accordingly when data is fetched or errors occur. Step 8: Check Component Lifecycle Methods In class components, lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount play a crucial role in managing the component’s behavior. When debugging: Ensure that state updates or side effects are correctly triggered within lifecycle methods. Double-check for memory leaks caused by improper cleanup in componentWillUnmount. In functional components, use the useEffect hook to manage side effects, ensuring that effects are properly cleaned up when the component unmounts. Step 9: Look for Unnecessary Re-Renders Unnecessary re-renders can drastically affect performance. React re-renders components whenever state or props change, but this can sometimes happen too frequently: Use React.memo or PureComponent to prevent unnecessary re-renders for stateless com
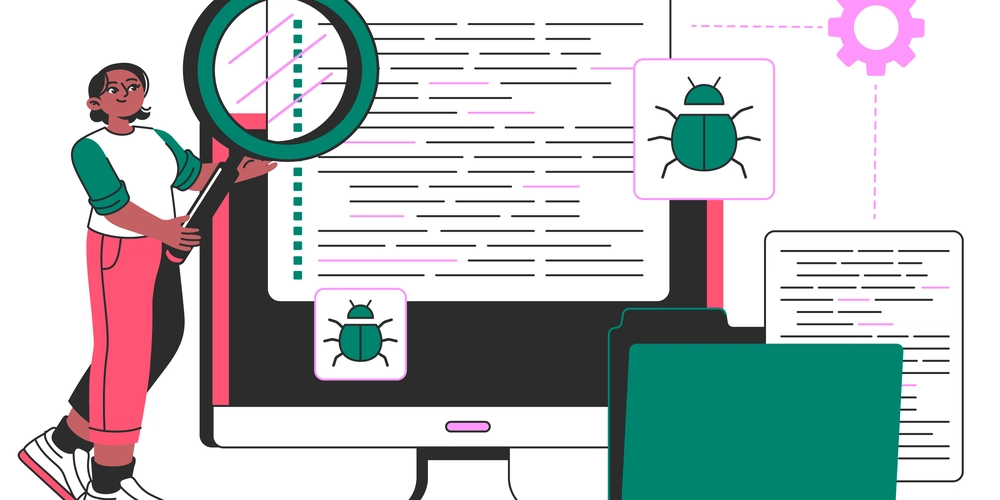
Bug Finding for React Developer
Overview
Bug finding in React development is an essential skill for creating robust and efficient applications. This guide outlines a systematic, step-by-step approach to identifying, isolating, and resolving issues within React applications. Following these steps, developers will enhance their debugging skills and ensure their applications are error-free and perform optimally.
Step 1: Check the Console for Errors
Before diving into the code, always begin with inspecting the browser’s console for any error messages. React will typically display informative messages in the console that can direct you to the issue. It could be an issue related to:
- Incorrectly used props or states
- Uncaught JavaScript errors
- Warnings related to missing or invalid keys in lists
By reading these messages, you can narrow down where the problem might lie, whether it's with component rendering, data fetching, or user interactions.
Step 2: Replicate the Issue Consistently
Once an error message is spotted, make sure you can consistently replicate the issue. Try to identify specific actions or conditions under which the bug appears:
- Does the bug appear on a specific route or component?
- Is it triggered by a user interaction, such as a button click or form submission?
- Does it happen after a certain timeout or API response?
By understanding exactly how to replicate the issue, you are better equipped to trace the cause.
Step 3: Examine the Component Tree with React DevTools
React DevTools is a powerful debugging tool that provides an in-depth view of your component hierarchy. It allows you to:
- Inspect the props and state of individual components
- Track component re-renders and performance
- Check for unnecessary re-renders that might be causing performance issues
By inspecting the component tree, you can pinpoint which components are not receiving the correct props or state and whether they are re-rendering unnecessarily.
Step 4: Check for Missing or Incorrect Props
Props are a core feature in React for passing data to components. When bugs appear related to the UI not rendering as expected, check if:
- The parent component is passing the correct props to the child component.
- The child component is correctly receiving and using the props.
- There are missing props that should be provided.
Ensure the types and values of props are consistent and match the expected structure.
Step 5: Verify the State and State Updates
State management issues are common culprits for bugs. In React, improper state initialization or incorrect state updates often lead to:
- UI not reflecting the latest data
- Performance issues due to unnecessary re-renders
Verify that state is being initialized correctly, updated in response to user actions, and is in sync with the component's UI. Make sure state updates use setState
(for class components) or the state setter function (useState
) in functional components.
Step 6: Ensure Proper Usage of Hooks
For functional components, React hooks like useState
, useEffect
, and useContext
are pivotal in managing state and side effects. Ensure:
-
useState
is being used properly for state updates. -
useEffect
is being correctly used for side effects (e.g., data fetching, subscriptions). - Hooks follow the rules of hooks, such as being called at the top level of the component and not inside loops or conditions.
Incorrect hook usage can lead to issues like infinite loops, unexpected behavior, or improper re-renders.
Step 7: Investigate Asynchronous Operations
Many React applications rely on asynchronous operations such as fetching data from APIs. Issues can arise when asynchronous calls are:
- Not handled properly with
async/await
or promises. - Missing error handling or fallback states (e.g., loading indicators or error messages).
- Triggered in an incorrect lifecycle method, resulting in race conditions.
Ensure that your asynchronous code is executed correctly, and that UI states are updated accordingly when data is fetched or errors occur.
Step 8: Check Component Lifecycle Methods
In class components, lifecycle methods like componentDidMount
, componentDidUpdate
, and componentWillUnmount
play a crucial role in managing the component’s behavior. When debugging:
- Ensure that state updates or side effects are correctly triggered within lifecycle methods.
- Double-check for memory leaks caused by improper cleanup in
componentWillUnmount
.
In functional components, use the useEffect
hook to manage side effects, ensuring that effects are properly cleaned up when the component unmounts.
Step 9: Look for Unnecessary Re-Renders
Unnecessary re-renders can drastically affect performance. React re-renders components whenever state or props change, but this can sometimes happen too frequently:
- Use
React.memo
orPureComponent
to prevent unnecessary re-renders for stateless components. - Ensure that you are not causing re-renders in an inefficient way, such as passing inline functions as props, which causes a new function instance on every render.
Track and optimize components that are re-rendering unnecessarily, which can improve performance.
Step 10: Investigate Conditional Rendering
React’s conditional rendering can cause issues if not managed correctly. Common mistakes include:
- Conditional rendering without fallback content or loading states.
- Rendering components in loops or conditions that may result in incorrect UI or layout shifts.
Ensure that all conditionally rendered elements or components are handled in a structured way to avoid rendering bugs.
Step 11: Ensure Proper Key Usage in Lists
When rendering lists in React, it is essential to provide a unique key
prop for each list item. React uses the key
to identify which items have changed, been added, or removed:
- Ensure that the key is a unique, stable identifier (such as a unique id from your data).
- Avoid using indices as keys, as it can lead to issues with reordering or dynamically changing data.
Incorrect or missing keys can cause rendering issues and make React inefficient at managing the DOM.
Step 12: Check for Global State Conflicts
If you're using global state management solutions like Redux or React Context, ensure that:
- The global state is properly initialized.
- State updates are correctly propagated across components.
- Components are subscribing to the global state and using it as expected.
Incorrect management of global state can lead to inconsistent or stale data being rendered in components.
Step 13: Examine Styles and Layout Issues
Sometimes, bugs may arise not from logic but from incorrect CSS or layout issues:
- Ensure that CSS classes are applied correctly.
- Check for layout shifts or elements being misplaced due to missing or conflicting styles.
- Use browser developer tools to inspect the elements and their computed styles.
Ensure your layout behaves responsively across different screen sizes and that styles don’t conflict with JavaScript-rendered elements.
Step 14: Verify Test Coverage
Unit and integration tests are crucial in identifying bugs early. If tests are not already written, add tests to cover components and features that are causing bugs:
- Write tests to verify the expected behavior of state and props updates.
- Use testing libraries like Jest and React Testing Library to simulate user interactions and component rendering.
Test coverage helps prevent regression and ensures that new changes don't break existing functionality.
Step 15: Perform Regression Testing
Finally, after fixing a bug, always perform regression testing to ensure that other parts of the application are not affected. This includes:
- Running the full test suite to verify that previously fixed bugs remain resolved.
- Manually testing critical workflows to ensure no new issues have been introduced.
Regression testing ensures that the application remains stable and that previously identified bugs are no longer present.