Mastering Dependency Injection in Spring Boot: Real-World Examples and Best Practices
Table of Contents What is Dependency Injection (DI)? How Dependency Injection Works in Spring Boot Types of Dependency Injection 3.1 Constructor Injection 3.2 Setter Injection 3.3 Field Injection Real-World Use Cases Where DI Shines Professional Project Structure Based on DI Golden Rules for Clean Dependency Injection Proper, Professional Spring Boot Project Structure That Naturally Forces Good Dependency Injection Practices Final Thoughts What is Dependency Injection (DI)? Dependency Injection means: You don't build your own dependencies. You get them handed to you. Instead of manually creating objects (using new), Spring Boot automatically creates, manages, and injects dependencies into your classes. Why care? Loose coupling Easier unit testing Configuration flexibility How Dependency Injection Works in Spring Boot Spring Boot scans your code. It finds classes annotated with @Component, @Service, @Repository, etc. It creates those objects ("beans") and stores them in the ApplicationContext. It injects these beans into any class that declares a dependency. Behind the scenes, it’s a giant, organized factory, automatically wiring your app together. Types of Dependency Injection Constructor Injection (Best Practice) @Service public class OrderService { private final PaymentService paymentService; @Autowired public OrderService(PaymentService paymentService) { this.paymentService = paymentService; } } ✅ Guarantees immutability. ✅ Easier to test. Setter Injection @Component public class InvoiceService { private TaxService taxService; @Autowired public void setTaxService(TaxService taxService) { this.taxService = taxService; } } ✅ Good for optional dependencies. Field Injection (Not Recommended) @Component public class UserService { @Autowired private EmailService emailService; } ❌ Harder to test. ❌ Hidden dependencies. Real-World Use Cases Where DI Shines 1. Switching Payment Gateways interface PaymentGateway { void pay(Order o); } @Service @Primary class PaypalGateway implements PaymentGateway { ... } @Service class StripeGateway implements PaymentGateway { ... } Switching Stripe to PayPal? Just change wiring, no code edits. 2. Mocking for Unit Testing @Test void testOrder() { PaymentService mockPayment = mock(PaymentService.class); OrderService service = new OrderService(mockPayment); // Assertions... } No Spring context needed, pure Java tests. 3. Supporting Multiple Databases @Repository @Profile("mysql") class MySqlUserRepository implements UserRepository { ... } @Repository @Profile("mongo") class MongoUserRepository implements UserRepository { ... } Switch DBs by flipping a profile. 4. Multi-channel Notifications @Service class NotificationService { public NotificationService(List notifiers) { this.notifiers = notifiers; } } Email + SMS + Push notifications. Adding new channels? No service edits needed. 5. Dynamic Pricing Strategies interface PricingStrategy { BigDecimal calculatePrice(Product p); } @Service class SeasonalDiscount implements PricingStrategy { ... } Plug new pricing logic without touching existing code. Proper, Professional Spring Boot Project Structure That Naturally Forces Good Dependency Injection Practices src/main/java/com/example/app ├── config/ │ ├── SwaggerConfig.java │ ├── SecurityConfig.java │ └── ApplicationConfig.java
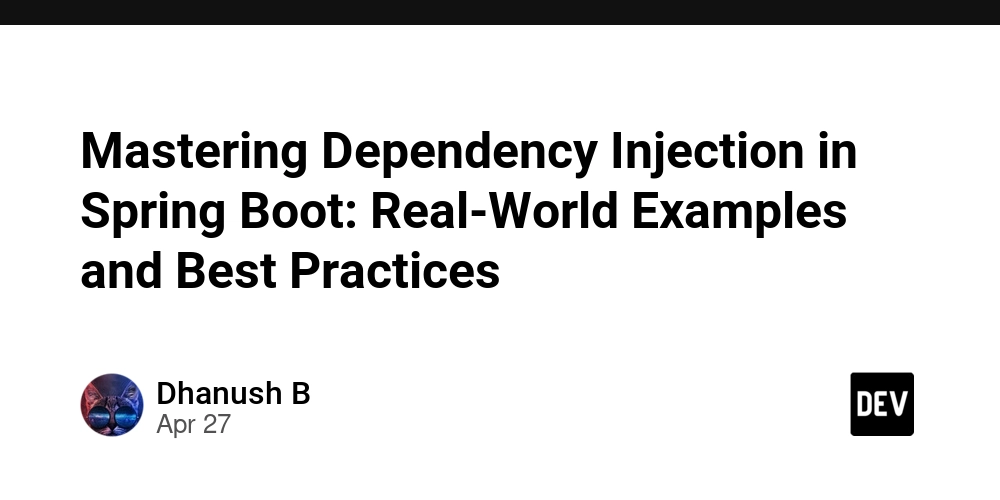
Table of Contents
- What is Dependency Injection (DI)?
- How Dependency Injection Works in Spring Boot
-
Types of Dependency Injection
- 3.1 Constructor Injection
- 3.2 Setter Injection
- 3.3 Field Injection
- Real-World Use Cases Where DI Shines
- Professional Project Structure Based on DI
- Golden Rules for Clean Dependency Injection
- Proper, Professional Spring Boot Project Structure That Naturally Forces Good Dependency Injection Practices
- Final Thoughts
What is Dependency Injection (DI)?
Dependency Injection means: You don't build your own dependencies. You get them handed to you.
Instead of manually creating objects (using new
), Spring Boot automatically creates, manages, and injects dependencies into your classes.
Why care?
- Loose coupling
- Easier unit testing
- Configuration flexibility
How Dependency Injection Works in Spring Boot
- Spring Boot scans your code.
- It finds classes annotated with
@Component
,@Service
,@Repository
, etc. - It creates those objects ("beans") and stores them in the
ApplicationContext
. - It injects these beans into any class that declares a dependency.
Behind the scenes, it’s a giant, organized factory, automatically wiring your app together.
Types of Dependency Injection
Constructor Injection (Best Practice)
@Service
public class OrderService {
private final PaymentService paymentService;
@Autowired
public OrderService(PaymentService paymentService) {
this.paymentService = paymentService;
}
}
✅ Guarantees immutability.
✅ Easier to test.
Setter Injection
@Component
public class InvoiceService {
private TaxService taxService;
@Autowired
public void setTaxService(TaxService taxService) {
this.taxService = taxService;
}
}
✅ Good for optional dependencies.
Field Injection (Not Recommended)
@Component
public class UserService {
@Autowired
private EmailService emailService;
}
❌ Harder to test.
❌ Hidden dependencies.
Real-World Use Cases Where DI Shines
1. Switching Payment Gateways
interface PaymentGateway { void pay(Order o); }
@Service @Primary
class PaypalGateway implements PaymentGateway { ... }
@Service
class StripeGateway implements PaymentGateway { ... }
Switching Stripe to PayPal? Just change wiring, no code edits.
2. Mocking for Unit Testing
@Test
void testOrder() {
PaymentService mockPayment = mock(PaymentService.class);
OrderService service = new OrderService(mockPayment);
// Assertions...
}
No Spring context needed, pure Java tests.
3. Supporting Multiple Databases
@Repository @Profile("mysql")
class MySqlUserRepository implements UserRepository { ... }
@Repository @Profile("mongo")
class MongoUserRepository implements UserRepository { ... }
Switch DBs by flipping a profile.
4. Multi-channel Notifications
@Service
class NotificationService {
public NotificationService(List<Notifier> notifiers) { this.notifiers = notifiers; }
}
Email + SMS + Push notifications. Adding new channels? No service edits needed.
5. Dynamic Pricing Strategies
interface PricingStrategy { BigDecimal calculatePrice(Product p); }
@Service
class SeasonalDiscount implements PricingStrategy { ... }
Plug new pricing logic without touching existing code.
Proper, Professional Spring Boot Project Structure That Naturally Forces Good Dependency Injection Practices
src/main/java/com/example/app
├── config/
│ ├── SwaggerConfig.java
│ ├── SecurityConfig.java
│ └── ApplicationConfig.java <-- Bean @Configuration classes
│
├── controller/
│ ├── OrderController.java
│ ├── UserController.java
│ └── PaymentController.java <-- Only calls Services, never Repositories directly
│
├── service/
│ ├── OrderService.java
│ ├── PaymentService.java
│ └── impl/
│ ├── StripePaymentService.java
│ ├── PaypalPaymentService.java <-- Multiple implementations
│
├── repository/
│ ├── UserRepository.java
│ └── OrderRepository.java <-- Extends JpaRepository/CrudRepository
│
├── model/
│ ├── Order.java
│ ├── User.java
│ └── Payment.java <-- Pure data classes (POJOs / Entities)
│
├── dto/
│ ├── OrderRequest.java
│ ├── PaymentRequest.java
│ └── ApiResponse.java <-- Request/Response objects
│
├── exception/
│ ├── GlobalExceptionHandler.java
│ ├── ResourceNotFoundException.java
│ └── CustomBadRequestException.java <-- Custom exceptions
│
├── util/
│ ├── JwtTokenUtil.java
│ ├── DateTimeUtil.java
│ └── EmailValidatorUtil.java <-- Helper utilities
│
└── AppApplication.java <-- Main @SpringBootApplication class
Example:
@RestController
public class OrderController {
private final OrderService orderService;
@Autowired
public OrderController(OrderService orderService) {
this.orderService = orderService;
}
}
@Service
public class OrderServiceImpl implements OrderService {
private final OrderRepository orderRepository;
@Autowired
public OrderServiceImpl(OrderRepository orderRepository) {
this.orderRepository = orderRepository;
}
}
@Repository
public interface OrderRepository extends JpaRepository<Order, Long> {}
What This Structure Gives You (that 99% miss)
Layer | Responsibility | DI Action |
---|---|---|
Controller | Handle HTTP requests | Inject Services via Constructor |
Service | Business logic orchestration | Inject Repositories and other Services |
Repository | Pure data access | Spring injects automatically |
Model | Data holders (Entities/POJOs) | No DI needed |
DTO | API Request/Response classes | No DI needed |
Config | Application setup (beans, profiles) | Define and inject custom beans |
Golden Project Rules:
- Controllers should only call services, not repositories.
- Services should orchestrate business logic.
- Repositories should handle only data access.
- Beans are injected via constructors.
- No manual
new
calls unless absolutely necessary.
Golden Rules for Clean Dependency Injection
- Prefer constructor injection always.
-
Use
@Primary
or@Qualifier
to resolve conflicts. - Never manually
new
services or repos. - Controllers call only services, not repositories directly.
- Configuration goes in
@Configuration
classes.
Final Thoughts
Dependency Injection isn’t just an "enterprise thing." It's about writing modular, scalable, maintainable code.
"If your code isn't clean without Spring, Spring Boot won't save it."
DI turns your app into a network of self-contained, plug-and-play modules instead of a brittle tower of jenga blocks.
Master it now, and your future self (and your sleep schedule) will thank you later.